#INTRODUCTION
Hello everyone , So today's topic we are going to talk about IOS animation and we're going to create an sample app along with the article as well. This time we will using third party library which is Lottie.
###1. WHAT IS LOTTIE?
Lottie is a third party library which develop by Airbnb. It renders After Effects animations. Animations are exported as JSON files through an open-source After Effects extension called Bodymovin. Lottie loads the animation data in JSON format and by using that JSON file, it give us the ability to deploy the after effect animation in our IOS App.
###2. HOW CAN WE USE LOTTIE?
To use Lottie We need the animation JSON file that we export from After effect using Bodymovin but if we don't have or don't know how to use after effect, we also can use Lottie's Predefine Animation from LottieFile website Go ahead and download some Lottie animation!.
###3. SAMPLE APP USING LOTTIE
Ok. Now let create Xcode Project.After Create the project we need install Lottie via Cocoa Pod. Below is my pod file.
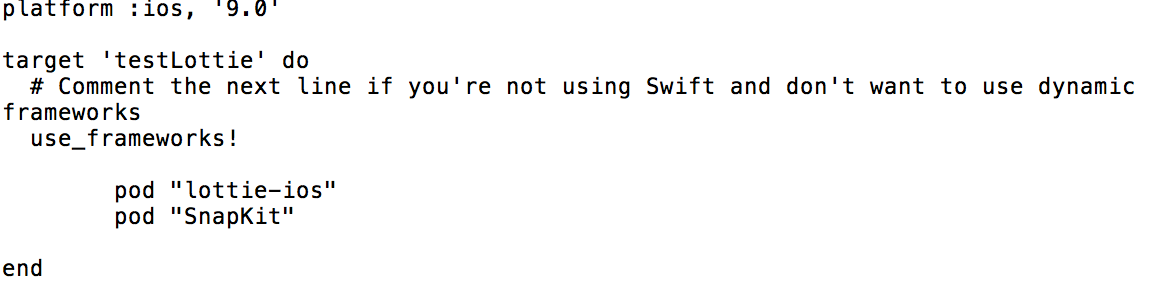
Do not confuse to use lottie we need to install only 1 pod which is lottie-ios.
- lottie-ios : Lottie Library - For Swift Animation.
-
SnapKit : SnapKit Library - For Swift Auto Layout.
Ok now let open the project and drag the Lottie JSON File in.
APPLICATION BREIF
So this app, There will be :
- Animation Object : Display the animation(Lottie JSON file).
- Button : The animation will start when user press button Click.
- Slider : User could control Animation Speed by drag slider
- Label : Show the animation Speed (Slider Value)
ViewController
import UIKit
import Lottie // Animation Library
import SnapKit // Layout Library
class ViewController: UIViewController {
var animation : LOTAnimationView!
var button : UIButton!
var label : UILabel!
var uiSlider : UISlider!
override func viewDidLoad() {
super.viewDidLoad()
self.view.backgroundColor = UIColor.white
createComponent()
addSupview()
setupLayout()
}
}
As Usual, There are 3 callback methods in the view controller to setup our UI.
createComponent Function
// MARK: - Create UI Component
extension ViewController {
func createComponent() -> Void {
//Lottie
animation = LOTAnimationView(name: "data")
//Label
label = UILabel()
label.textAlignment = .center
label.text = "50"
//Slider
uiSlider = UISlider()
uiSlider.minimumValue = 1
uiSlider.maximumValue = 100
uiSlider.value = 50
uiSlider.isContinuous = true
//Button
button = UIButton()
button.setTitle("START", for: .normal)
button.backgroundColor = UIColor.blue
}
}
In above class , I'm going to explain about LOTanimation only since I have explain many time about the creation of another component. So if you don't understand please go to see my previous article.
animation = LOTAnimationView(name: "data")
Here we are creating Lottie Object and passing the Lottie JSON file along (data is Animation JSON file's name).
addSupview Function
// MARK: - Add Supview
extension ViewController {
func addSupview() -> Void {
self.view.addSubview(label)
self.view.addSubview(animation)
self.view.addSubview(button)
self.view.addSubview(uiSlider)
}
}
setupLayout Function
// MARK: - Setup Layout
extension ViewController {
func setupLayout() -> Void {
//Lottie
animation.snp.makeConstraints { (make) in
make.width.equalToSuperview()
make.height.equalToSuperview()
}
//Label
label.snp.makeConstraints { (make) in
make.width.equalToSuperview()
make.height.equalTo(40)
make.centerX.equalTo(self.view.snp.centerX)
make.bottom.equalTo(uiSlider.snp.top)
}
//Slider
uiSlider.snp.makeConstraints { (make) in
make.width.equalTo(300)
make.height.equalTo(40)
make.centerX.equalTo(self.view.snp.centerX)
make.bottom.equalToSuperview().offset(-20)
}
//Button
button.snp.makeConstraints { (make) in
make.width.equalTo(100)
make.height.equalTo(30)
make.centerX.equalTo(self.view.snp.centerX)
make.bottom.equalTo(label.snp.top)
}
}
}
Ok Now we have completed setting up the UI now let add some action to Button and Slider.
ViewController
import UIKit
import Lottie // Animation Library
import SnapKit // Layout Library
class ViewController: UIViewController {
var animation : LOTAnimationView!
var button : UIButton!
var label : UILabel!
var uiSlider : UISlider!
override func viewDidLoad() {
super.viewDidLoad()
self.view.backgroundColor = UIColor.white
createComponent()
addSupview()
setupLayout()
//Slider Action
uiSlider.addTarget(self, action: #selector(valueChange), for: .valueChanged)
//Button Action
button.addTarget(self, action: #selector(btnClick), for: .touchUpInside)
}
//Slider Drag
@objc func valueChange() -> Void {
//Bind Slider Value to Label
label.text = String(Int(round(uiSlider.value)))
//Bind With AnimationSpeed
let speed = round(uiSlider.value) / 100
animation.animationSpeed = CGFloat(speed)
}
//Button Click
@objc func btnClick() -> Void {
animation.stop()
animation.play()
}
}
Action Explain
- Slider : Slider action is , The slider will trigger when the user drag the slider and we are passing the slider value to the label and we also control the animation speed.
- Button : Button action is , The animation will start when the button is click.
YES! That's it guys and for more detail you could see at Lottie Github.
https://github.com/airbnb/lottie-ios