前回の記事でマテリアルのフェイスアサインがあるかないかを判別するコードを書きましたが、通常のオブジェクトアサインがあるかどうかの判定も合わせて、詳細な判定ができるようにコードを記述してみます。
サクッと作ってみる
def has_object_assign(shape):
"""
シェイプにマテリアルのオブジェクトアサインがあるかどうか確認する
:param shape: チェックするシェイプ
:type shape: pymel.nodetypes.Shape
:rtype: bool
"""
instanceNumber = shape.instanceNumber()
instObjGroup = shape.instObjGroups[instanceNumber]
cons = pm.listConnections(instObjGroup, plugs=False)
for con in cons:
if con.type() == 'shadingEngine':
return True
return False
instObjGroupにSGのコネクションがあるかどうか調べているだけです。
これと前回のhas_face_assign
関数を組み合わせると、シェイプに何らかのマテリアルがアサインされているかがわかります。
def has_any_assign(shape):
"""
シェイプに何らかのマテリアルのオブジェクトアサインがあるかどうか確認する
:param shape: チェックするシェイプ
:type shape: pymel.nodetypes.Shape
:rtype: bool
"""
return has_face_assign(shape) or has_object_assign(shape)
処理に重複があるのと、そもそも重そうな処理判定なので、現実的なパフォーマンスが出るかはさておきですが。。
ところで、shapeには関連しているsetsを返すメソッドがあるので、
def has_any_assign2(shape):
set_list = shape.listSets()
for s in set_list:
if s.type() == 'shadingEngine':
return True
return False
何らかのマテリアルアサインがあるかどうかの判定は、こんな感じでもいけました。この処理でもちゃんとインスタンスの区別がされていました。
フェイスアサイン、オブジェクトアサインの判定もこんな感じでもっとスマートに判定できる方法がありそうな気もしますが、動くものを作って理解を進めているということで。。
動作検証
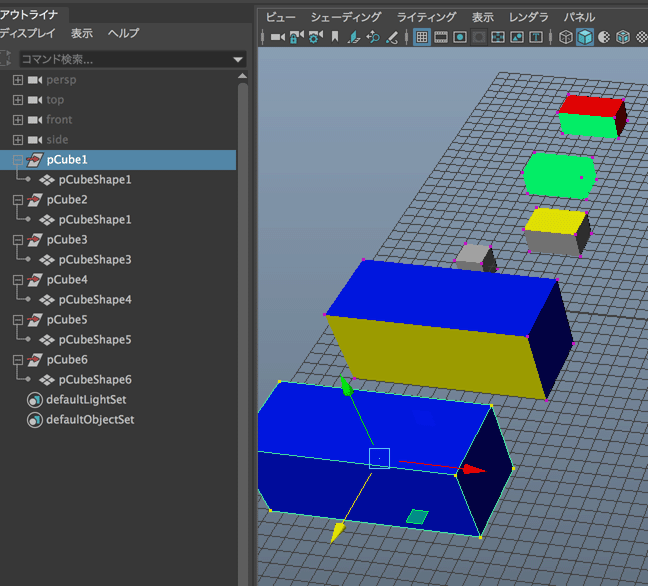
shape = pm.PyNode('pCube1|pCubeShape1') # フェイスアサインのないインスタンスシェイプ
print(has_face_assign(shape)) # -> False
print(has_object_assign(shape)) # -> True
print(has_any_assign(shape)) # -> True
print(has_any_assign2(shape)) # -> True
print('')
shape = pm.PyNode('pCube2|pCubeShape1') # フェイスアサインのあるインスタンスシェイプ
print(has_face_assign(shape)) # -> True
print(has_object_assign(shape)) # -> False
print(has_any_assign(shape)) # -> True
print(has_any_assign2(shape)) # -> True
print('')
shape = pm.PyNode('pCube3|pCubeShape3') # インスタンスが存在しないフェイスアサインのないシェイプ
print(has_face_assign(shape)) # -> False
print(has_object_assign(shape)) # -> True
print(has_any_assign(shape)) # -> True
print(has_any_assign2(shape)) # -> True
print('')
shape = pm.PyNode('pCube4|pCubeShape4') # インスタンスが存在しないフェイスアサインのあるシェイプ
print(has_face_assign(shape)) # -> True
print(has_object_assign(shape)) # -> False
print(has_any_assign(shape)) # -> True
print(has_any_assign2(shape)) # -> True
print('')
shape = pm.PyNode('pCube5|pCubeShape5') # マテリアルが外れてしまったシェイプ
print(has_face_assign(shape)) # -> False
print(has_object_assign(shape)) # -> False
print(has_any_assign(shape)) # -> False
print(has_any_assign2(shape)) # -> False
print('')
shape = pm.PyNode('pCube6|pCubeShape6') # 一部faceのマテリアルが外れてしまったシェイプ
print(has_face_assign(shape)) # -> True
print(has_object_assign(shape)) # -> False
print(has_any_assign(shape)) # -> True
print(has_any_assign2(shape)) # -> True