前の投稿の調査を元にインスタンスを選択して実行すると親インスタンスを選択し直すコードを作ってみたので貼っておきます。(実際はインスタンスは親も子もなくて全て兄弟ぽいのですが、わかりやすく表現しています。)
スクリプトエディタにペーストする用です。シェルフに登録すると楽に使えると思います。
MAYA2018 + MacOS Sierraで動作確認しています。
使い方
- ノードを選択状態にします。対象としてはインスタンスメッシュそのもの、またはその親トランスフォームとなります。
- Scriptを実行します。
- 親インスタンスノードがあれば選択状態になります。なければエラーメッセージが表示されます。



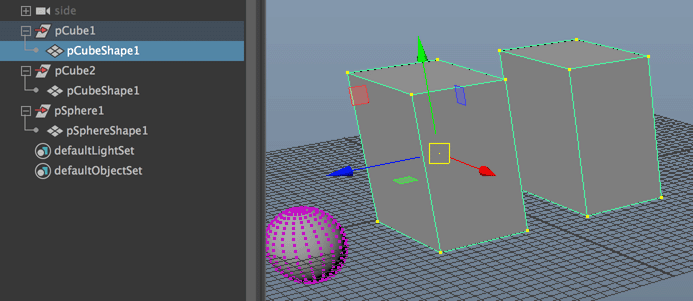



対応していないパターン
選択ノードの直接の子供のうちで1つもインスタンスがない場合。ツリーを再帰的に探索はしません。
クイック実行用コード
import pymel.core as pm
def get_first_instance(node):
"""
インスタンスの親(=0番目のインスタンス)を得る
:param node: 親を探すインスタンスノード参照
:type node: pymel.nodetypes.DagNode
:return: 見つかった親ノードとエラー情報ストリング
:rtype: tuple of DagNode and str
"""
org_node = node
target_is_children = False
is_instanced = node.isInstanced()
if not is_instanced:
# 選択がインスタンスでない場合は子供を探す
target_is_children = True
children = pm.listRelatives(node)
node = None
for child_node in children:
if child_node.isInstanced():
node = child_node
break
if node is None:
return None, '対象インスタンスが見つかりません。'
instances = node.getOtherInstances()
instance_number = node.instanceNumber()
if instance_number == 0:
return org_node, 'ノード自身が親です。'
found = None
for ins_node in instances:
if ins_node.instanceNumber() == 0:
found = ins_node
break
if found is not None:
if target_is_children:
parents = pm.listRelatives(found, parent=True)
return parents[0], None
else:
return found, None
return None, '親インスタンスが見つかりません。'
def select_first_instance():
selected = pm.selected()
if len(selected) == 0:
pm.confirmDialog(title='Info', message='ノードを選択して下さい。', button=['Ok'])
return
node = selected[0]
first_node, error_info = get_first_instance(node)
if error_info is not None:
pm.confirmDialog(title='Info', message=error_info, button=['Ok'])
if first_node is not None:
print first_node
pm.select(first_node)
select_first_instance()
del select_first_instance
del get_first_instance