はじめに
Markdown AI は、マークダウン記法で Web サイトが作れるサービスです。そして、script タグが使えるため、JavaScript を埋め込むこともできます。
私は、この JavaScript を埋め込めるという特徴に着目し「ちょっとしたゲームくらいなら作れるかも」と思いました。そして、それは正解でして、意外と簡単に作れてしまったのです。
今回、AI の力をかりて 2 つのゲームを作ってきましたので、本記事で紹介させてください。(遊んでいただくことも可能です。)
作成したゲーム
①選択肢で "1024" を目指すゲーム
②ランダムな数字を当てるゲーム
①選択肢で "1024" を目指すゲーム
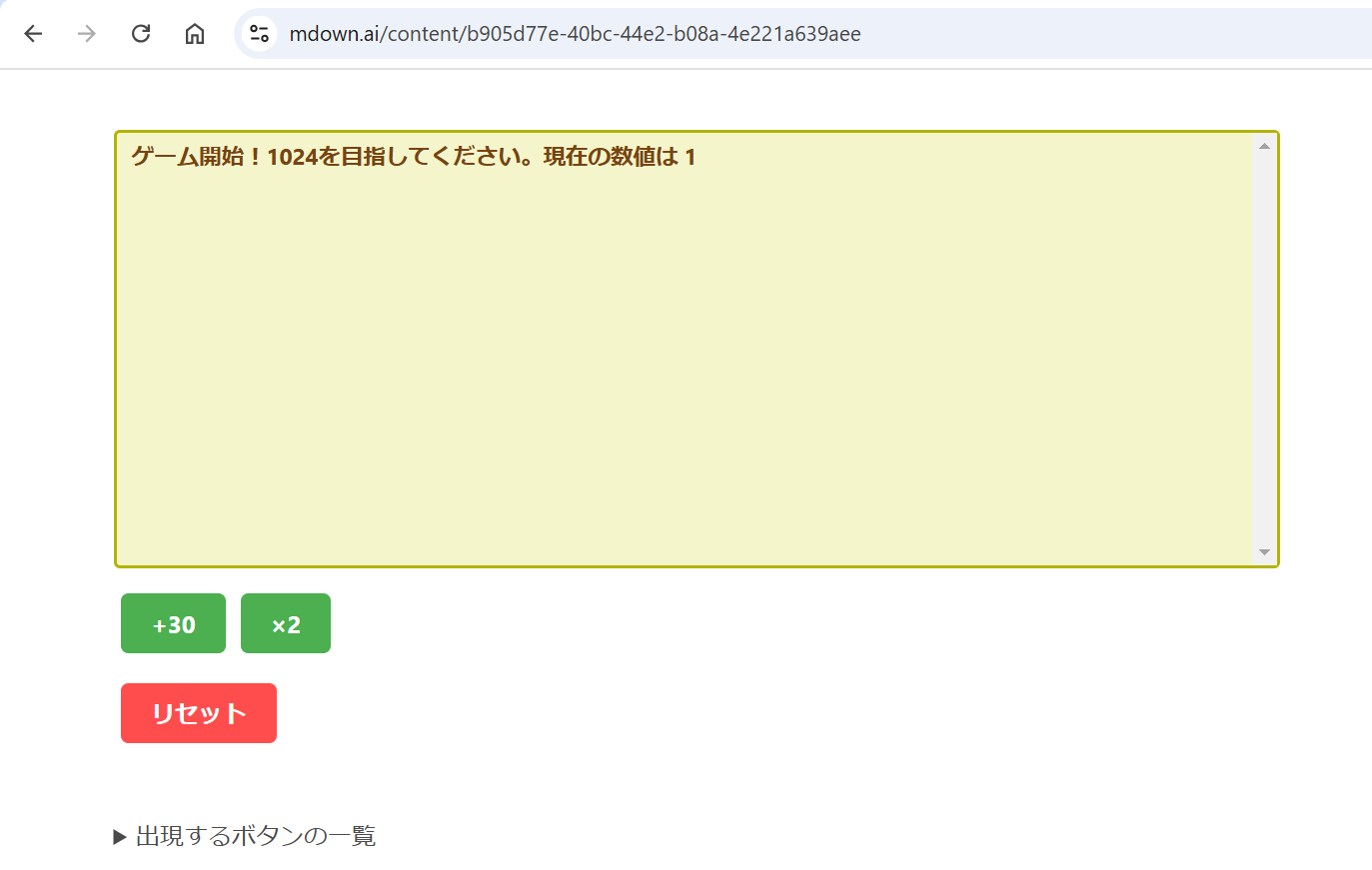
緑色のボタン(選択肢)によって数字が増えていくゲームです。選択肢は選ぶたびにランダムで変更されます。出現する選択肢は画面下部で確認することができます。1024 を超えるとゲームオーバーです。
マークダウン
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>1024</title>
<style>
body {
font-family: Arial, sans-serif;
padding: 20px;
}
textarea {
width: 70%;
background-color: #f5f5cc;
color: #774411;
font-size: 15px;
font-weight: bold;
height: 50vh;
overflow-y: scroll;
resize: none;
border: 2px solid #b3b300;
border-radius: 4px;
padding-top: 5px;
padding-left: 10px;
}
#buttonsContainer {
margin: 5px 0;
}
button {
padding: 10px 20px;
margin: 5px;
font-size: 16px;
font-weight: bold;
color: white;
background-color: #4CAF50;
border: none;
border-radius: 5px;
cursor: pointer;
transition: background-color 0.3s, transform 0.2s;
height: 40px; /* 固定の高さを設定 */
line-height: 1.2; /* テキストの高さをボタン内で調整 */
}
button:disabled {
background-color: #ccc;
cursor: not-allowed;
}
button:hover:enabled {
background-color: #45a049;
transform: scale(1.05);
}
#resetButton {
background-color: #ff4d4d;
margin-top: 10px;
}
#resetButton:hover {
background-color: #ff3333;
}
</style>
</head>
<body>
<textarea readonly id="gameDisplay"></textarea>
<div id="buttonsContainer">
<!-- ボタンが動的に生成されます -->
</div>
<button id="resetButton" onClick="resetGame()">リセット</button>
<script>
let number = 1;
let steps = 0;
const actions = [
{ label: "+1", apply: () => { number += 1; } },
{ label: "+2", apply: () => { number += 2; } },
{ label: "+3", apply: () => { number += 3; } },
{ label: "+5", apply: () => { number += 5; } },
{ label: "+10", apply: () => { number += 10; } },
{ label: "+20", apply: () => { number += 20; } },
{ label: "+30", apply: () => { number += 30; } },
{ label: "+50", apply: () => { number += 50; } },
{ label: "+100", apply: () => { number += 100; } },
{ label: "+200", apply: () => { number += 200; } },
{ label: "+300", apply: () => { number += 300; } },
{ label: "+500", apply: () => { number += 500; } },
{ label: "+1024", apply: () => { number += 1024; } },
{ label: "×2", apply: () => { number *= 2; } },
{ label: "1桁目を2倍", apply: () => { number = Math.floor(number / 10) * 10 + (number % 10) * 2; } },
{ label: "2桁目を2倍", apply: () => {
const hundreds = Math.floor(number / 100) * 100;
const tens = Math.floor((number % 100) / 10) * 2 * 10;
const units = number % 10;
number = hundreds + tens + units;
}
},
{ label: "3桁目を2倍", apply: () => {
const thousands = Math.floor(number / 1000) * 1000;
const hundreds = Math.floor((number % 1000) / 100) * 2 * 100;
const tensAndUnits = number % 100;
number = thousands + hundreds + tensAndUnits;
}
},
];
function randomizeButtons() {
const container = document.getElementById("buttonsContainer");
container.innerHTML = "";
const shuffledActions = actions.sort(() => Math.random() - 0.5).slice(0, 2);
shuffledActions.forEach(action => {
const button = document.createElement("button");
button.innerText = action.label;
button.onclick = () => {
action.apply();
steps += 1;
updateDisplay(action.label);
randomizeButtons();
};
if (number > 1024) {
button.disabled = true;
}
container.appendChild(button);
});
}
function updateDisplay(action) {
const target = document.getElementById("gameDisplay");
let message = `${steps}回目の操作 (${action}): 現在の数値は ${number}`;
if (number === 1024) {
message += "\n\nおめでとう!1024に到達しました!";
} else if (number > 1024) {
message += "\n\n残念!1024を超えました。『リセット』をしてください。";
}
target.value += "\n" + message;
}
function resetGame() {
number = 1;
steps = 0;
const target = document.getElementById("gameDisplay");
target.value = "ゲーム開始!1024を目指してください。現在の数値は 1";
randomizeButtons();
}
// 初期メッセージをセット
document.getElementById("gameDisplay").value = "ゲーム開始!1024を目指してください。現在の数値は 1";
// 初期化
randomizeButtons();
</script>
</body>
</html>
<br><!-- 改行を追加 -->
<details><summary>出現するボタンの一覧</summary>
- +1
- +2
- +3
- +5
- +10
- +20
- +30
- +50
- +100
- +200
- +300
- +500
- +1024
- 1桁目を2倍
- 2桁目を2倍
- 3桁目を2倍
</details>
②ランダムな数字を当てるゲーム
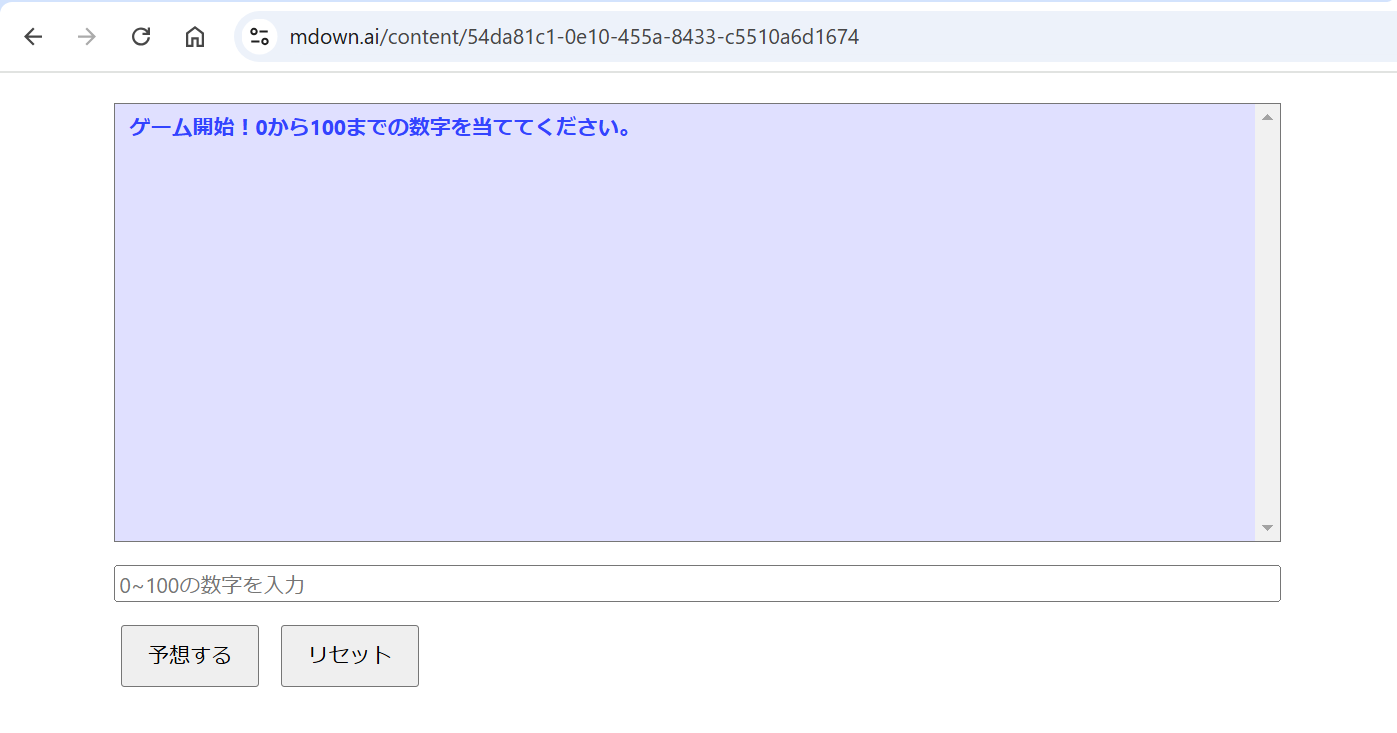
最初に 0 から 100 のランダムな数字(これを当てる)が作成されます。好きな数字を入力し「予想する」をクリックすると、その数字より大きいか小さいかが表示されます。それをヒントに、何回のヒントで当てることができるか?のゲームです。
マークダウン
<style>
textarea {
width: 70%;
background-color: #e0e0ff;
color: #3344ff;
font-size: 14px;
font-weight: bold;
height: 50vh;
overflow-y: scroll;
resize: none;
padding-top: 5px;
padding-left: 10px;
}
#guessInput {
width: 70%;
margin-top: 10px;
font-size: 14px;
}
.button-container {
margin-top: 10px;
}
.button-container button {
margin: 5px;
padding: 8px 16px;
font-size: 14px;
}
</style>
<textarea readonly>
ゲーム開始!0から100までの数字を当ててください。
</textarea>
<input type="number" id="guessInput" placeholder="0~100の数字を入力">
<div class="button-container">
<button onClick="guessNumber()">予想する</button>
<button onClick="resetGame()">リセット</button>
</div>
<script>
let targetNumber = Math.floor(Math.random() * 101);
let attempts = 0;
function guessNumber() {
const guess = Number(document.getElementById("guessInput").value);
const target = document.querySelector("textarea");
attempts += 1;
let message = "";
if (guess < targetNumber) {
message = `残念!もっと小さいよ! ${attempts}回目の予想: ${guess}`;
} else if (guess > targetNumber) {
message = `残念!もっと大きいよ! ${attempts}回目の予想: ${guess}`;
} else {
message = `正解! ${attempts}回で当てました!`;
}
target.value += "\n" + message;
document.getElementById("guessInput").value = "";
}
function resetGame() {
targetNumber = Math.floor(Math.random() * 101);
attempts = 0;
const target = document.querySelector("textarea");
target.value = "ゲーム開始!0から100までの数字を当ててください。\n"; // メッセージの後に改行
document.getElementById("guessInput").value = "";
}
</script>
おわりに
AI に作ってもらったゲームをコピペして、ボタンひとつで Web サイトにしてみました。作業時間としては 30 分ほどで、こんな簡単に公開できたことに驚いています。
また、この記事では Markdown AI の JavaScript を埋め込めるという特徴に着目しましたが、実は「簡単に Web 公開できる」というのも素晴らしい特徴です。そのおかげで、皆さんにも遊んでもらうことができていますし、SNSなどで簡単に共有することもできています。
次は「簡単に Web 公開できる」という特徴もうまく使ってみたいですね。Markdown AI、まだまだいろいろ使えそうです。
以上です。