はじめに
pythonでグラフを作成するときの定番はmatplotlib
ですが、設定項目が多いのでいちいち調べて使うのは大変です。
基本の使用方法を列挙して、すぐに使えるようにしておこうと思います。
参考
- https://matplotlib.org/api/_as_gen/matplotlib.pyplot.plot.html
- http://ailaby.com/plot_marker/
- http://bicycle1885.hatenablog.com/entry/2014/02/14/023734
- http://ailaby.com/subplots_adjust/
- https://qiita.com/ponnhide/items/2b6279324ae792523020
- https://qiita.com/termoshtt/items/f6d6ee7a1f93175870e4
環境
- Google colab
- matplotlib==3.0.2
手順
散布図
まずは基本の散布図。
import matplotlib.pyplot as plt
import numpy as np
import numpy.random as random
random.seed(0) # シード値の固定
x = np.random.randn(100)
y = np.sin(x) + np.random.randn(100)
# グラフの大きさ指定
plt.figure(figsize=(5, 5))
# グラフの描写
plt.plot(x, y, 'o', label='first')
# これはplt.plot(x, y, marker='o', label='first', linestyle='')と同じ。
plt.title('title') # タイトル
plt.xlabel('x-axis label') # x軸のラベル
plt.ylabel('y-axis label') # y軸のラベル
plt.grid(True) # gridの表示
plt.legend() # 凡例の表示

plt.plot(x, y, marker='o', label='first', linestyle='')
は、線を引かずに、各点のマーカーのみ表示するという意味になります。
軸のアスペクト比を1:1にする
plt.axis('equal')
で軸のアスペクト比を1:1にできます。その他座標軸の設定はmatplotlibで座標軸を調整するにあります。
import matplotlib.pyplot as plt
import numpy as np
import numpy.random as random
random.seed(0) # シード値の固定
x = np.random.randn(100)
y = np.sin(x) + np.random.randn(100)
# グラフの大きさ指定
plt.figure(figsize=(5, 5))
# グラフの描写
plt.plot(x, y, 'o', label='first')
# plt.plot(x, y, marker='o', label='first', linestyle='')
plt.title('title') # タイトル
plt.xlabel('x-axis label') # x軸のラベル
plt.ylabel('y-axis label') # y軸のラベル
plt.grid(True) # gridの表示
plt.legend() # 凡例の表示
plt.axis('equal') # x軸とy軸の比を等しくする
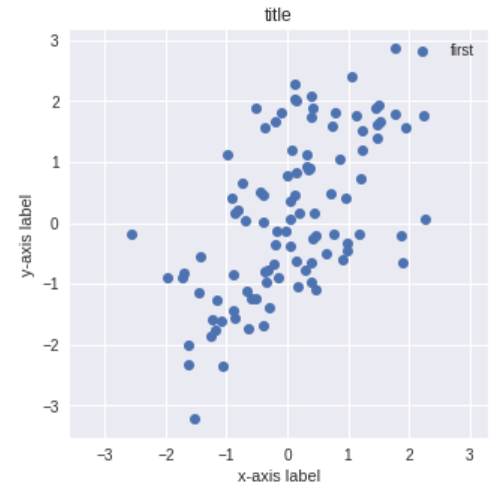
軸の範囲、最小単位、軸の文字サイズ、軸のラベルサイズを変更
軸の範囲はplt.xlim
、plt.ylim
、最小単位はplt.xticks
、plt.yticks
、文字を表示するものは大体引数にfontsize
があります。
import matplotlib.pyplot as plt
import numpy as np
import numpy.random as random
random.seed(0) # シード値の固定
x = np.random.randn(100)
y = np.sin(x) + np.random.randn(100)
# グラフの大きさ指定
plt.figure(figsize=(5, 5))
# グラフの描写
plt.plot(x, y, 'o', label='first')
# これはplt.plot(x, y, marker='o', label='first', linestyle='')と同じ。
plt.title('title', fontsize=20) # タイトル
plt.xlabel('x-axis label', fontsize=15) # x軸のラベル
plt.ylabel('y-axis label', fontsize=15) # y軸のラベル
plt.xlim([-10,10]) # x軸の範囲
plt.ylim([-10,10]) # y軸の範囲
plt.xticks(np.arange(-10, 11, 1), fontsize=7) # x軸の最小単位
plt.yticks(np.arange(-10, 11, 1), fontsize=7) # y軸の最小単位
plt.grid(True) # gridの表示
plt.legend() # 凡例の表示
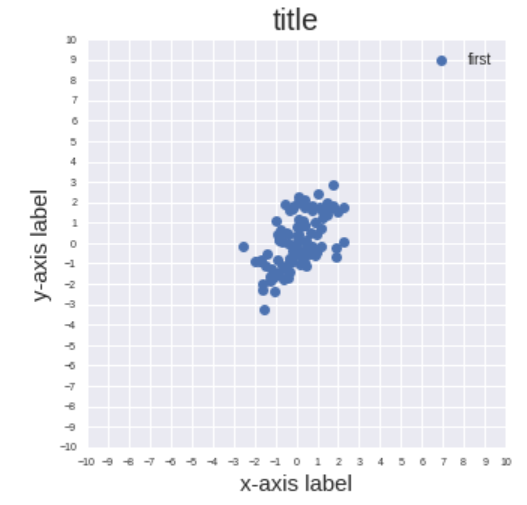
軸をlogスケールにする
xscale
、yscale
で軸のスケールを変えられます。
import matplotlib.pyplot as plt
import numpy as np
import numpy.random as random
random.seed(0) # シード値の固定
x = np.random.randn(100)
y = np.exp(x) + np.random.randn(100)
# グラフの大きさ指定
plt.figure(figsize=(5, 5))
# グラフの描写
plt.plot(x, y, 'o', label='first')
# これはplt.plot(x, y, marker='o', label='first', linestyle='')と同じ。
plt.title('title') # タイトル
plt.xlabel('x-axis label') # x軸のラベル
plt.ylabel('y-axis label') # y軸のラベル
plt.yscale('log') # y軸のスケールをlogにする
plt.grid(True) # gridの表示
plt.legend() # 凡例の表示

グラフを重ねる
複数グラフを重ねるときはplt.plot()
を複数にします。
import matplotlib.pyplot as plt
import numpy as np
import numpy.random as random
random.seed(0) # シード値の固定
x = np.random.randn(100)
y = np.sin(x) + np.random.randn(100)
# グラフの大きさ指定
plt.figure(figsize=(5, 5))
# グラフの描写
plt.plot(x, y, 'o', label='first')
plt.plot(x, x+y, 'o', label='second')
plt.title('title') # タイトル
plt.xlabel('x-axis label') # x軸のラベル
plt.ylabel('y-axis label') # y軸のラベル
plt.grid(True) # gridの表示
plt.legend()
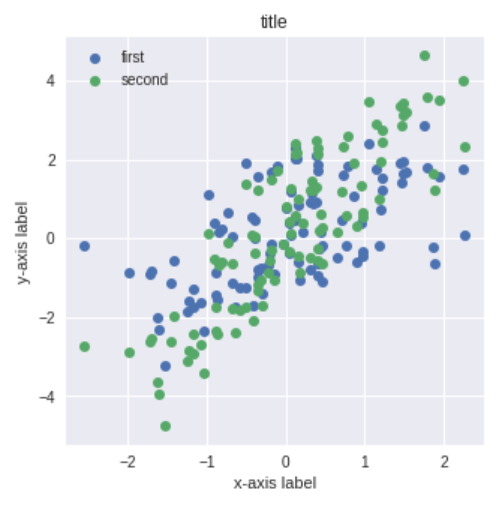
透過と色
alpha
で透過度を、color
で色を指定できます。
import matplotlib.pyplot as plt
import numpy as np
import numpy.random as random
random.seed(0) # シード値の固定
x = np.random.randn(100)
y = np.sin(x) + np.random.randn(100)
# グラフの大きさ指定
plt.figure(figsize=(5, 5))
# グラフの描写
plt.plot(x, y, 'o', label='first', alpha=0.2, color='r')
plt.title('title') # タイトル
plt.xlabel('x-axis label') # x軸のラベル
plt.ylabel('y-axis label') # y軸のラベル
plt.grid(True) # gridの表示
plt.legend()
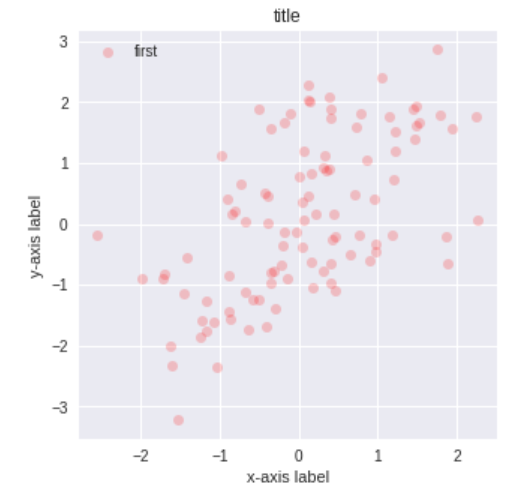
色の指定は次の通りです。
character | color |
---|---|
'b' | blue |
'g' | green |
'r' | red |
'c' | cyan |
'm' | magenta |
'y' | yellow |
'k' | black |
'w' | white |
連続曲線
linestyleを指定すると線の形状を変えられます。
import matplotlib.pyplot as plt
import numpy as np
import numpy.random as random
random.seed(0) # シード値の固定
x = np.linspace(0, 10, 100)
y = np.sin(x)
# グラフの大きさ指定
plt.figure(figsize=(5, 5))
# グラフの描写
plt.plot(x, y, '-', label='first')
# plt.plot(x, y, label='first', linestyle='-') # でも同じ
plt.title('title') # タイトル
plt.xlabel('x-axis label') # x軸のラベル
plt.ylabel('y-axis label') # y軸のラベル
plt.grid(True) # gridの表示
plt.legend()
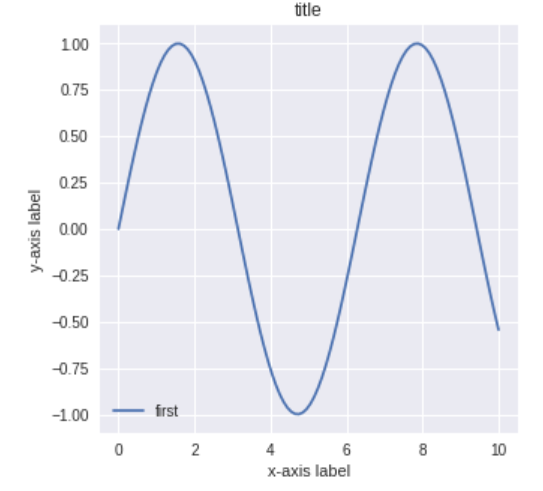
第3引数はlinestyle
とmarker
を勝手に判別してくれるようです。
import matplotlib.pyplot as plt
import numpy as np
import numpy.random as random
random.seed(0) # シード値の固定
x = np.linspace(0, 10, 100)
y = np.sin(x)
# グラフの大きさ指定
plt.figure(figsize=(5, 5))
# グラフの描写
plt.plot(x, y, '-o', label='first')
# plt.plot(x, y, marker='o', label='first', linestyle='-') # でも同じ
plt.title('title') # タイトル
plt.xlabel('x-axis label') # x軸のラベル
plt.ylabel('y-axis label') # y軸のラベル
plt.grid(True) # gridの表示
plt.legend(['new label'], loc='lower right')
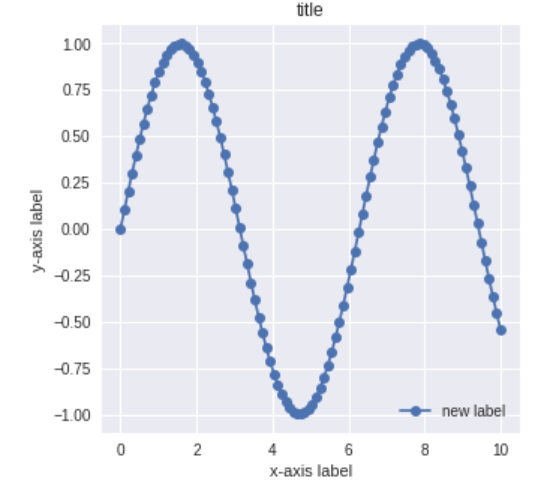
plt.legend()
に配列を渡すとラベルを変えられます。loc
で表示位置を決められます。
線の太さとマーカーの大きさはlinewidth
、markersize
で決められます。
import matplotlib.pyplot as plt
import numpy as np
import numpy.random as random
random.seed(0) # シード値の固定
x = np.linspace(0, 10, 100)
y = np.sin(x)
# グラフの大きさ指定
plt.figure(figsize=(5, 5))
# グラフの描写
plt.plot(x, y, '-o', label='first', linewidth=0.1, markersize=8)
plt.title('title') # タイトル
plt.xlabel('x-axis label') # x軸のラベル
plt.ylabel('y-axis label') # y軸のラベル
plt.grid(True) # gridの表示
plt.legend()

linestyle
は下記が使用できます。
character | description |
---|---|
'-' | solid line style |
'--' | dashed line style |
'-.' | dash-dot line style |
':' | dotted line style |
marker
は下記が使用できます。(colabだと表示されないものがいくつかあるようです)
matplotlib で使える marker を全て試してみる-pythonでグラフが見られます。
character | description | 備考 |
---|---|---|
'.' | point marker | |
',' | pixel marker | |
'o' | circle marker | |
'v' | triangle_down marker | |
'^' | triangle_up marker | |
'<' | triangle_left marker | |
'>' | triangle_right marker | |
'1' | tri_down marker | colabだと表示されない |
'2' | tri_up marker | colabだと表示されない |
'3' | tri_left marker | colabだと表示されない |
'4' | tri_right marker | colabだと表示されない |
's' | square marker | |
'p' | pentagon marker | |
'*' | star marker | |
'h' | hexagon1 marker | |
'H' | hexagon2 marker | |
'+' | plus marker | colabだと表示されない |
'x' | x marker | colabだと表示されない |
'D' | diamond marker | |
'd' | thin_diamond marker | |
バーティカルバー | vline marker | colabだと表示されない |
'_' | hline marker | colabだと表示されない |
グラフを複数表示する
subplots
を使用します。subplots
はfig
と'ax'を戻り値として返します。ax
は配列で、subplots(n,m)
で決めた(n,m)の位置のグラフを作成するのに使用します。
plt.plot
とは軸ラベルやタイトルの設定の仕方が微妙に異なるので注意が必要です。
1行にグラフを3つ表示する場合。
import matplotlib.pyplot as plt
import numpy as np
import numpy.random as random
random.seed(0) # シード値の固定
x = np.linspace(0, 10, 100)
y = []
y.append(np.sin(x))
y.append(np.cos(x))
y.append(np.tan(x))
# タイトルを配列に格納
title=['title1','title2','title3']
# 3つのグラフを配置する枠をつくる
# figが3つのグラフが入る全体の枠で、axがそれぞれのグラフ
fig,ax = plt.subplots(1,3,figsize=(18,5))
# グラフ間の幅を調整
plt.subplots_adjust(wspace=0.4, hspace=0.3)
# グラフを作る
for i in range(3):
ax[i].plot(x, y[i], '-')
ax[i].set_title(title[i]) # タイトル
ax[i].set_xlabel('x-axis label') # x軸のラベル
ax[i].set_ylabel('y-axis label') # y軸のラベル
plt.show()

2行3列のグラフをつくります。
import matplotlib.pyplot as plt
import numpy as np
import numpy.random as random
random.seed(0) # シード値の固定
x = np.linspace(0, 10, 100)
y = [[[] for i in range(3)] for i in range(2) ]
y[0][0] = np.sin(x) + np.random.randn(100)
y[0][1] = np.sin(x)
y[0][2] = np.cos(x)
y[1][0] = x**2
y[1][1] = np.log(x+1)
y[1][2] = np.exp(x)
names=['1','2','3']
fig,ax=plt.subplots(2,3,figsize=[10,5])
plt.subplots_adjust(wspace=0.6, hspace=0.6)
for i in range(2):
for j in range(3):
ax[i,j].plot(x,y[i][j])
ax[i,j].set_xlabel('x-axis label') # x軸のラベル
ax[i,j].set_ylabel('y-axis label') # x軸のラベル
ax[i,j].set_title('%s-%s'%(names[i],names[j]))
plt.show()
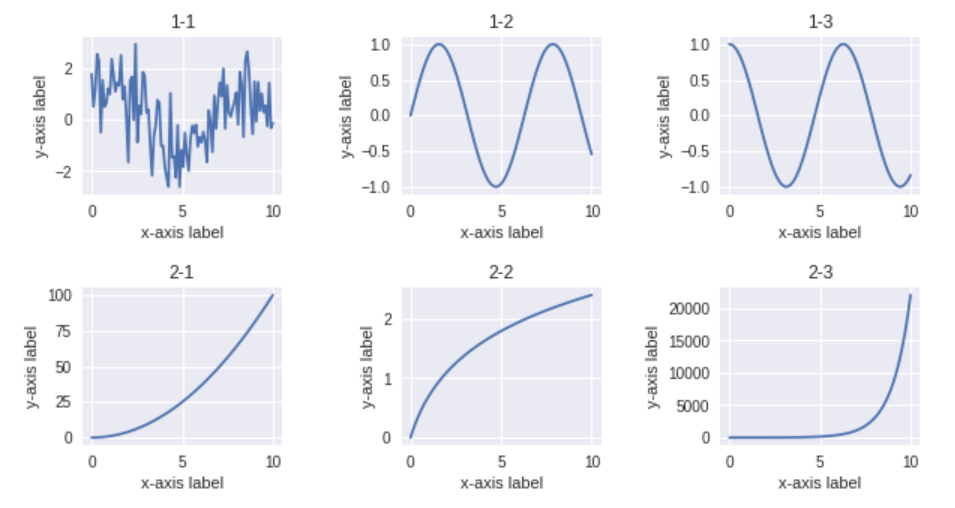
レイアウトの詳細はmatplotlib基礎 | figureやaxesでのグラフのレイアウトがわかりやすいです。
軸を揃える場合はsharey
を使います。row
、col
、all
で行ごと、列ごと、全てのグラフで軸を揃えることができます。
import matplotlib.pyplot as plt
import numpy as np
import numpy.random as random
random.seed(0) # シード値の固定
x = np.linspace(0, 10, 100)
y = [[[] for i in range(3)] for i in range(2) ]
y[0][0] = np.sin(x) + np.random.randn(100)
y[0][1] = np.sin(x)
y[0][2] = np.cos(x)
y[1][0] = x**2
y[1][1] = np.log(x+1)
y[1][2] = np.exp(x)
names=['1','2','3']
fig,ax=plt.subplots(2, 3, figsize=[10,5], sharey='row')
plt.subplots_adjust(wspace=0.6, hspace=0.6)
for i in range(2):
for j in range(3):
ax[i,j].plot(x,y[i][j])
ax[i,j].set_xlabel('x-axis label') # x軸のラベル
ax[i,j].set_ylabel('y-axis label') # x軸のラベル
ax[i,j].set_title('%s-%s'%(names[i],names[j]))
plt.show()
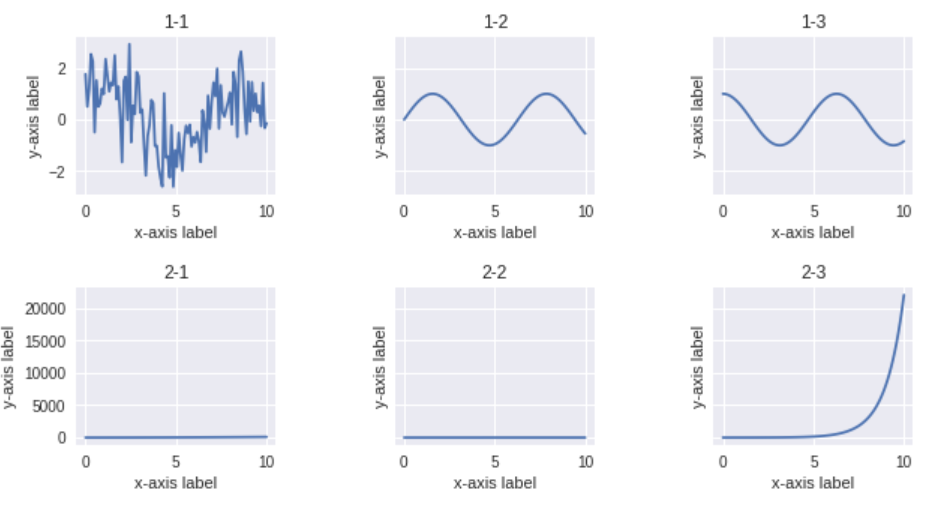
グラフ1個でもsubplots
は使えます。
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 2*np.pi, 400)
y = np.sin(x**2)
fig, ax = plt.subplots()
ax.plot(x, y)
ax.set_title('Simple plot')
