準備
XCode
AppStoreからダウンロード
これがないと始まらない
MacのOSバージョンによってインストールできない可能性あり。
常に最新レベルじゃないとすぐにインストールできなくなるので注意。
Node/NPM
Node・・・Node.jsのこと。サーバーサイドで動くjavascriptです。
NPM・・・パッケージ管理ツール。ReactやReactNative、Babelなどのjsの拡張機能を管理するツール
Nodeのインストール
brew install node
NPMのインストール
watchman
brew install watchman
ファイルの変更を監視してくれるツール
RN CLI(React Native Command Line Interface)
npm i -g react-native-cli
※参考
https://rara-world.com/react_native-build/
https://rara-world.com/react-native-tutorial/
Hello Worldを表示
react-native init reactHello
※途中「info Installing required CocoaPods dependencies」で止まっているかのように遅い
cd reactHello
npm i
react-native run-ios
※シュミレーター起動(初回は遅い)
Welcome to Reactというタイトルの画面が出るはずです。
今後バージョンによって変わる可能性あり
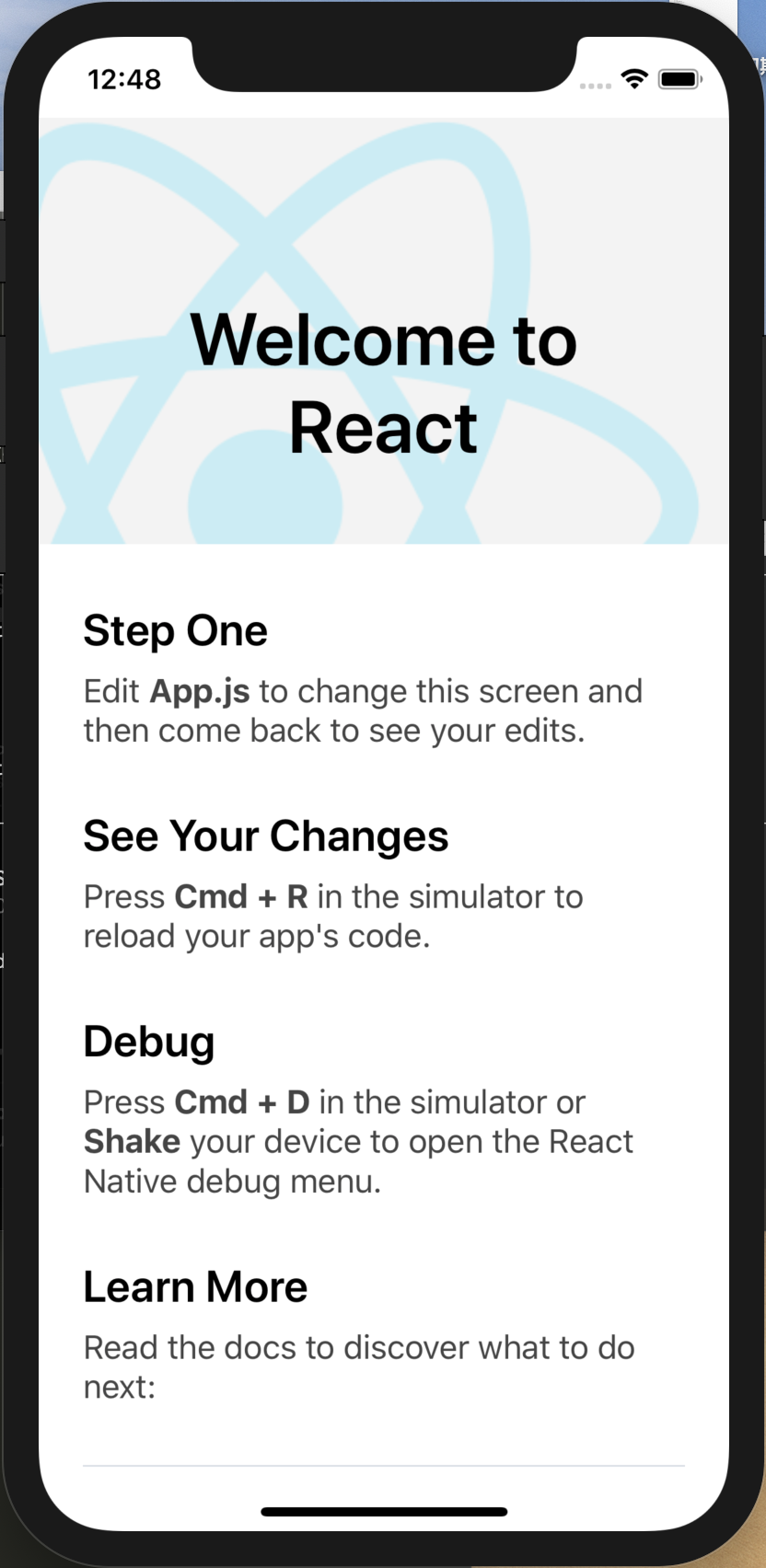
ディレクトリやファイル
├── App.js
├── tests
├── android
├── app.json
├── index.js
├── ios
├── node_modules
├── package-lock.json
├── package.json
└── yarn.lock
+追加
└── src この中に子コンポーネント入れていく
親Componentを作る
App.jsを書き換え
※参考記事でindex.jsになっていますが、記事ではおそらく前のバージョンでのことだと思います
import React from 'react';
import { Text, AppRegistry } from 'react-native';
const App = () => (
<Text>Hello World!</Text>
);
export default App;
// 記事の「AppRegistry.registerComponent('test', () => App);」だとエラーになった
これで「Hello World!」が表示されました.
子Componentを作る
import React from 'react';
import { Text, View } from 'react-native';
const Header = () => {
const { headerText, header } = styles;
return (
<View style={header}>
<Text style={headerText}>ヘッダー</Text>
</View>
);
};
const styles = {
header: {
backgroundColor: '#F8F8F8',
justifyContent: 'center',
alignItems: 'center',
height: 90,
paddingTop: 25,
elevation: 2,
position: 'relative'
},
headerText: {
fontSize: 20,
fontWeight: '600'
}
};
export default Header;
App.js修正
// 追加
import Header from './src/Header';
// 修正
const App = () => (
<View>
<Header />
</View>
);
親から子へパラメータを渡す
App.js修正
....
// 修正
const App = () => (
<View>
<Header showText={'Hello'} />
</View>
);
....
src/Header.js修正
....
const Header = (props) => {
const { headerText, header } = styles;
return (
<View style={header}>
<Text style={headerText}>{props.showText}</Text>
</View>
);
};
.....

font関係でエラーの場合の対処法
https://wp-kyoto.net/react-native-vector-icons-red-screen-with-unrecognized-font-family-error-on-ios/