tmlib.jsでSlider (この名称で合ってるか知らない) を実装しました。
今回はShapeを使用しています。画像指定すればSpriteも可。
そして引数が多い…
/**
* Sliderクラス
*/
var Slider = tm.createClass({
superClass: tm.app.Shape,
value: 0, // Sliderの現在値
min: null, // 最小値
max: null, // 最大値
default: null, // 初期値
dragging: false, // スライド中フラグ
disabled: false, // disabled
init: function(x, y, width, height, margin, min, max, def, sliderImage, gripImage) {
this.superInit(width, height + (margin==null ? 100 : margin));
this.setPosition(x, y);
// 値の初期化
this.min = ((min == null) ? 1 : min) - 1;
this.max = ((max == null) ? 100 : max);
this.default = (def == null) ? this.min : def;
this.value = this.default;
// Slider追加
if (sliderImage != null) {
var img = tm.graphics.TextureManager.get(sliderImage);
this.slider = tm.app.Sprite(img.width, img.height, sliderImage).setPosition(0, 0);
} else {
this.slider = tm.app.RectangleShape(width, height / 2, {
fillStyle: "gray",
strokeStyle: "black",
lineWidth: "1"
});
}
this.slider.setPosition(0, 0);
this.slider.addChildTo(this);
// グリップ初期位置
var pos = this.value * width / (this.max - this.min);
// 初期位置のずれ防止
if (this.value <= this.min) { pos = 0; }
if (this.value >= this.max) { pos = this.width; }
// グリップ追加
if (gripImage != null) {
var img = tm.graphics.TextureManager.get(gripImage);
this.slideGrip = tm.app.Sprite(img.width, img.height, gripImage);
} else {
this.slideGrip = tm.app.CircleShape(this.height/2.5, this.height/2.5, {
fillStyle: "white",
strokeStyle: "black",
lineWidth: "5",
});
}
this.slideGrip.setPosition(pos - (this.width/2), 0);
this.slideGrip.addChildTo(this);
// マウスイベントを有効化
this.interaction.enabled = true;
this.interaction.boundingType = "rect";
},
// タッチ開始
onpointingstart: function(e) {
if (this.disabled) {
this.dragging = false;
return;
}
// スライド開始はグリップ上をポイントしていること
var gp = this.localToGlobal(this.pointing);
if (this.slideGrip.isHitPoint(e.app.pointing.x - gp.x, e.app.pointing.y - gp.y)) {
this.dragging = true;
}
},
// スワイプ
onpointingmove: function(e) {
// グリップがドラッグ状態であればSlider上のポインタ座標に移動する
var gp = this.localToGlobal(this.pointing);
if (this.dragging && this.isHitPoint(e.app.pointing.x, e.app.pointing.y)) {
this.slideGrip.setPosition(e.app.pointing.x - gp.x, this.slideGrip.y);
this.value = (this.slideGrip.x + (this.width / 2)) * ((this.max - this.min) / this.width);
}
},
// タッチ終了
onpointingend: function() {
this.dragging = false;
},
// 現在値をIntで受け取る
getIntValue: function() {
return parseInt(this.value);
},
// 現在値をFloat(加工無し)で受け取る
getFloatValue: function() {
return this.value;
},
});
使用例(Sliderクラスの定義は省略)
/*
* constant
*/
var SCREEN_WIDTH = 640;
var SCREEN_HEIGHT = 920;
var SCREEN_CENTER_X = SCREEN_WIDTH / 2;
var SCREEN_CENTER_Y = SCREEN_HEIGHT / 2;
/*
* メイン処理(ページ読み込み後に実行される)
*/
tm.main(function() {
// アプリケーション作成
var app = tm.app.CanvasApp("#world");
app.resize(SCREEN_WIDTH, SCREEN_HEIGHT); // リサイズ
app.fitWindow(); // 自動フィット
app.background = "white";
// シーンを切り替える
app.replaceScene(MainScene());
// 実行
app.run();
});
tm.define("MainScene", {
superClass: "tm.app.Scene",
init: function() {
// 親の初期化
this.superInit();
// Slider
this.slider = Slider(320, 400, 400, 21, 100, 0, 200, 100);
this.slider.addChildTo(this);
// Sliderの現在値表示用ラベル
this.sliderValue = tm.app.Label("0");
this.sliderValue.setPosition(70, 410).setFillStyle("black").addChildTo(this);
},
update: function(app) {
this.sliderValue.text = this.slider.getIntValue();
},
});
実行結果 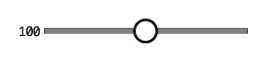 グッと掴んでスッと動かせば数値が変わります。 横方向の認識範囲のマージンが取れてないので改善の余地あり。