概要
この記事ではLaravelとYoutube Data api(v3)を用いて
Youtubeの特定チャンネルのtitleを抽出し、
ブラウザに表示する機能のサンプルを実装します。
検索や一覧表示の
参考ドキュメント
手順
事前準備
- YoutubeのAPIを叩くためのアプリケーションキーを取得
- Youtube APIを有効化
Google Youtube API 認証などで検索すれば出てくるはずです。
実施手順
インストール
$ composer create-project --prefer-dist laravel/laravel bollate "5.8.*"
起動確認
APPキーを生成
$ php artisan key:generate
起動確認
いつものLaravel画面が表示できればOK
GoogleAPIをインストール
phpでGoogleのAPIを使うにあたり便利なライブラリが存在するので利用します(感謝)
google-api-php-client
公式の通りインストール
$ composer require google/apiclient:"^2.0"
GOOGLE_KEYを.envに設定
GOOGLE_API_KEY = "YOURKEYXXXX"
Controller生成
$php artisan make:controller YoutubeController
以下はサンプルです。ライブラリが保持するクラスの関数やプロパティは
APIのリファレンスやライブラリのドキュメントを参照ください。
<?php
namespace App\Http\Controllers;
use App\Http\Controllers\Controller;
use Google_Client;
use Google_Service_YouTube;
class YoutubeController extends Controller
{
const MAX_SNIPPETS_COUNT = 50;
const DEFAULT_ORDER_TYPE = 'viewCount';
public function getListByChannelId(string $channelId)
{
// Googleへの接続情報のインスタンスを作成と設定
$client = new Google_Client();
$client->setDeveloperKey(env('GOOGLE_API_KEY'));
// 接続情報のインスタンスを用いてYoutubeのデータへアクセス可能なインスタンスを生成
$youtube = new Google_Service_YouTube($client);
// 必要情報を引数に持たせ、listSearchで検索して動画一覧を取得
$items = $youtube->search->listSearch('snippet', [
'channelId' => $channelId,
'order' => self::DEFAULT_ORDER_TYPE,
'maxResults' => self::MAX_SNIPPETS_COUNT,
]);
// 連想配列だと扱いづらいのでcollection化して処理
$snippets = collect($items->getItems())->pluck('snippet')->all();
return view('youtube/index')->with(['snippets' => $snippets]);
}
}
Routing生成
Route::get('youtube/channels/{id}/titles', 'Api\YoutubeController@getListByChannelId');
View生成
<!doctype html>
<html lang="ja">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Youtube</title>
<link rel="stylesheet" href="/css/app.css">
<link href="https://fonts.googleapis.com/icon?family=Material+Icons" rel="stylesheet">
</head>
<body class="index">
<div class="main">
<div class="header">
<div class="header__title">
<h1 class="header__title__main">
Youtube Title List;
</h1>
<p class="header__title__sub">
This is Youtube Title GET;
</p>
</div>
</div>
<div class="content">
<div class="content__body">
@foreach ($snippets as $snippet)
<div class="content__body__videos">
<p>
{{ $snippet->title }}
</p>
</div>
@endforeach
</div>
</div>
</div>
</body>
</html>
実行
$ php artisan serve
チャネルのIDをURLで指定することで特定チャンネルの動画のタイトルリストを得ることができました。
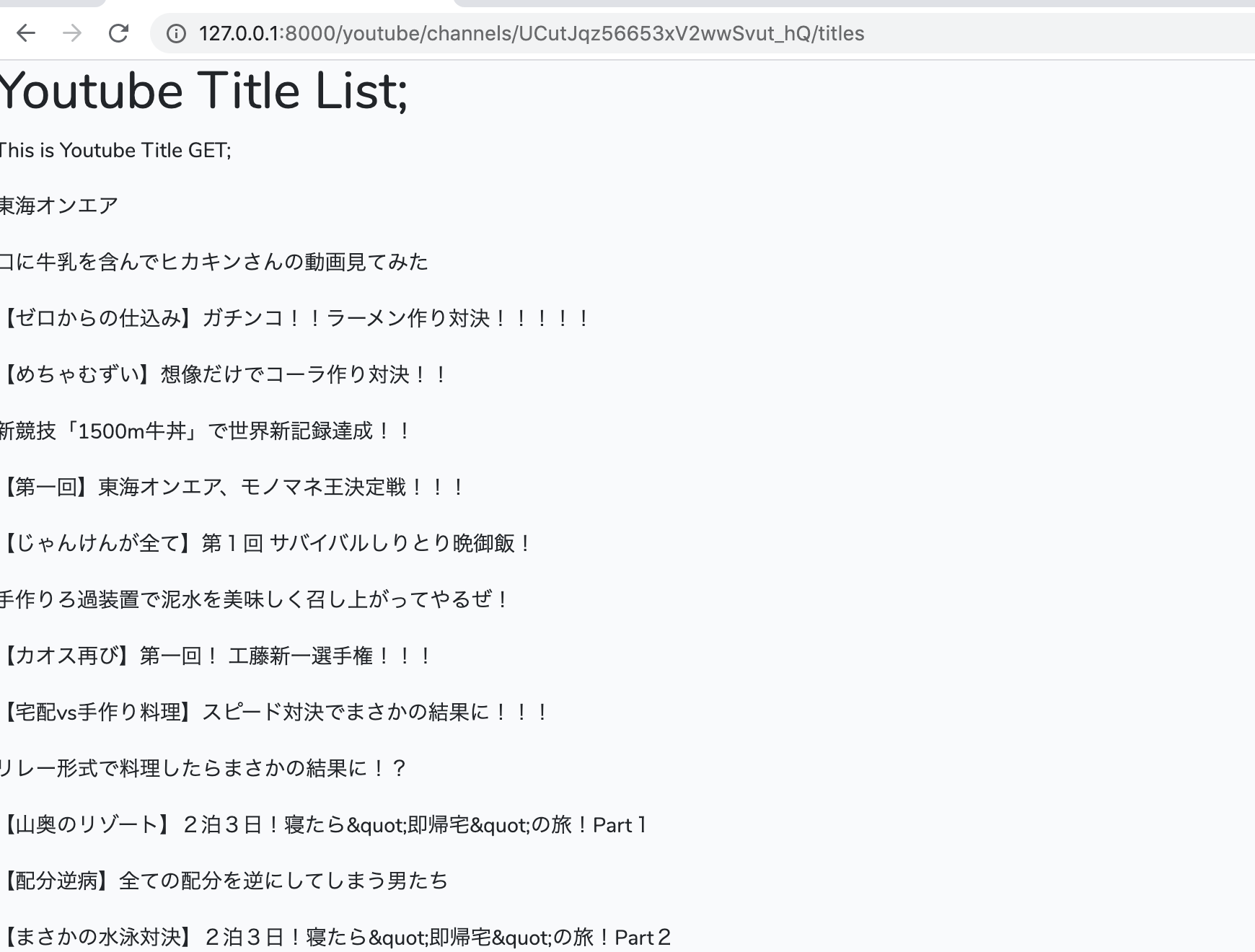
画像内容データ引用元:
【東海オンエア チャンネル】
https://www.youtube.com/channel/UCutJqz56653xV2wwSvut_hQ
他にも、動画をアップロード・再生リストの作成など、様々な操作を実行可能です。
よきAPIライフを