こんにちは。
多くの先人たちがブログなどで知識を残していってくれているので書く必要はないかと思いますが、自分用に記事を書いてみました。
導入方法
こちらの記事をみてください。
[iPhone] Google Maps SDK for iOS を使って地図を表示する
iOS向けGoogleマップSDKチュートリアル(Swift)-SDKのダウンロード
ドットインストール
注意点としては
Googleでキーを取得する認証情報上のライブラリを選択し、Maps SDK for ios のAPIを有効にすることを忘れないでください。
参考動画 Google Maps SDK for iOSのインストール、設定や地図を含めたiOSマップのアプリの起動ガイド
ピンを画像に変換する方法
import UIKit
import GoogleMaps
import GooglePlaces
class ViewController: UIViewController, GMSMapViewDelegate {
var mapView: GMSMapView!
override func viewDidLoad() {
super.viewDidLoad()
let camera = GMSCameraPosition.camera(withLatitude: 34.077875549971,
longitude: 134.56156512254,
zoom: 10.0)
let mapView = GMSMapView.map(withFrame: view.frame,
camera: camera)
self.view = mapView //Main.storyboardのviewに表示
let marker = GMSMarker()
//marker.icon = GMSMarker.markerImage(with: .black) //ピンの色変更
marker.position = CLLocationCoordinate2D(latitude: 34.077875549971,
longitude: 134.56156512254)
marker.title = "メインタイトル"
marker.snippet = "サブタイトル"
marker.icon = self.imageWithImage(image: UIImage(named: "camera_1")!, scaledToSize: CGSize(width: 50.0, height: 50.0))
marker.tracksViewChanges = true
marker.map = mapView
mapView.delegate = self //いらないかも
}
//ピンの縮尺を変更する
func imageWithImage(image:UIImage, scaledToSize newSize:CGSize) -> UIImage{
UIGraphicsBeginImageContextWithOptions(newSize, false, 1.0)
image.draw(in: CGRect(x: 0, y: 0, width: newSize.width, height: newSize.height))
let newImage:UIImage = UIGraphicsGetImageFromCurrentImageContext()!
UIGraphicsEndImageContext()
return newImage
}
camera_1という画像を表示させた
プロジェクト名を右クリックしAdd files to "プロジェクト名" を選んで追加する。
ピンをタップした際、xibファイル(ボタンやラベル、ImageView)を表示する方法
このサイトと同じことをやってください
Interactive InfoWindow GoogleMaps iOS
ただコードが写真だったのでコピペできるようにマークダウンで書いておきます。
全コード
ViewController.swift
import UIKit
import GoogleMaps
import GooglePlaces
class ViewController: UIViewController, GMSMapViewDelegate {
var mapView: GMSMapView!
var tappedMarker : GMSMarker? //追加
var customInfoWindow : CustomInfoWindow? //追加
override func viewDidLoad() {
super.viewDidLoad()
let camera = GMSCameraPosition.camera(withLatitude: 34.077875549971,
longitude: 134.56156512254,
zoom: 10.0)
let mapView = GMSMapView.map(withFrame: view.frame,
camera: camera)
self.view = mapView //Main.storyboardのviewに表示
let marker = GMSMarker()
//marker.icon = GMSMarker.markerImage(with: .black) //ピンの色変更
marker.position = CLLocationCoordinate2D(latitude: 34.077875549971,
longitude: 134.56156512254)
marker.title = "メインタイトル"
marker.snippet = "サブタイトル"
marker.icon = self.imageWithImage(image: UIImage(named: "camera_1")!, scaledToSize: CGSize(width: 50.0, height: 50.0))
marker.tracksViewChanges = true
marker.map = mapView
mapView.delegate = self
self.tappedMarker = GMSMarker() //追加
self.customInfoWindow = CustomInfoWindow().loadView() //追加
}
//ピンの縮尺を変更する
func imageWithImage(image:UIImage, scaledToSize newSize:CGSize) -> UIImage{
UIGraphicsBeginImageContextWithOptions(newSize, false, 1.0)
image.draw(in: CGRect(x: 0, y: 0, width: newSize.width, height: newSize.height))
let newImage:UIImage = UIGraphicsGetImageFromCurrentImageContext()!
UIGraphicsEndImageContext()
return newImage
}
//以下 追加
func mapView(_ mapView: GMSMapView, didTap marker: GMSMarker) -> Bool {
NSLog("marker was tapped")
tappedMarker = marker
let position = marker.position
mapView.animate(toLocation: position)
let point = mapView.projection.point(for: position)
let newPoint = mapView.projection.coordinate(for: point)
let camera = GMSCameraUpdate.setTarget(newPoint)
mapView.animate(with: camera)
let opaqueWhite = UIColor(white: 1, alpha: 0.85)
customInfoWindow?.layer.backgroundColor = opaqueWhite.cgColor
customInfoWindow?.layer.cornerRadius = 8
customInfoWindow?.center = mapView.projection.point(for: position)
mapView.addSubview(customInfoWindow!)
return false
}
func mapView(_ mapView: GMSMapView, markerInfoWindow marker: GMSMarker) -> UIView? {
return UIView()
}
func mapView(_ mapView: GMSMapView, didTapAt coordinate: CLLocationCoordinate2D) {
customInfoWindow?.removeFromSuperview()
}
func mapView(_ mapView: GMSMapView, didChange position: GMSCameraPosition) {
let position = tappedMarker?.position
customInfoWindow?.center = mapView.projection.point(for: position!)
customInfoWindow?.center.y -= 140
}
参考サイトと少し違うよ
CustomInfoWindow.swift
import UIKit
import Foundation
class CustomInfoWindow: UIView {
var view : UIView!
@IBOutlet weak var Image: UIImageView!
@IBOutlet weak var label: UILabel!
@IBAction func button(_ sender: UIButton) {
label.text = "タップされました"
}
override init(frame: CGRect) {
super.init(frame: frame)
}
required init?(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)
}
func loadView() -> CustomInfoWindow{
let customInfoWindow = Bundle.main.loadNibNamed("CustomInfoWindow", owner: self, options: nil)?[0] as! CustomInfoWindow
return customInfoWindow
}
}
xibファイル
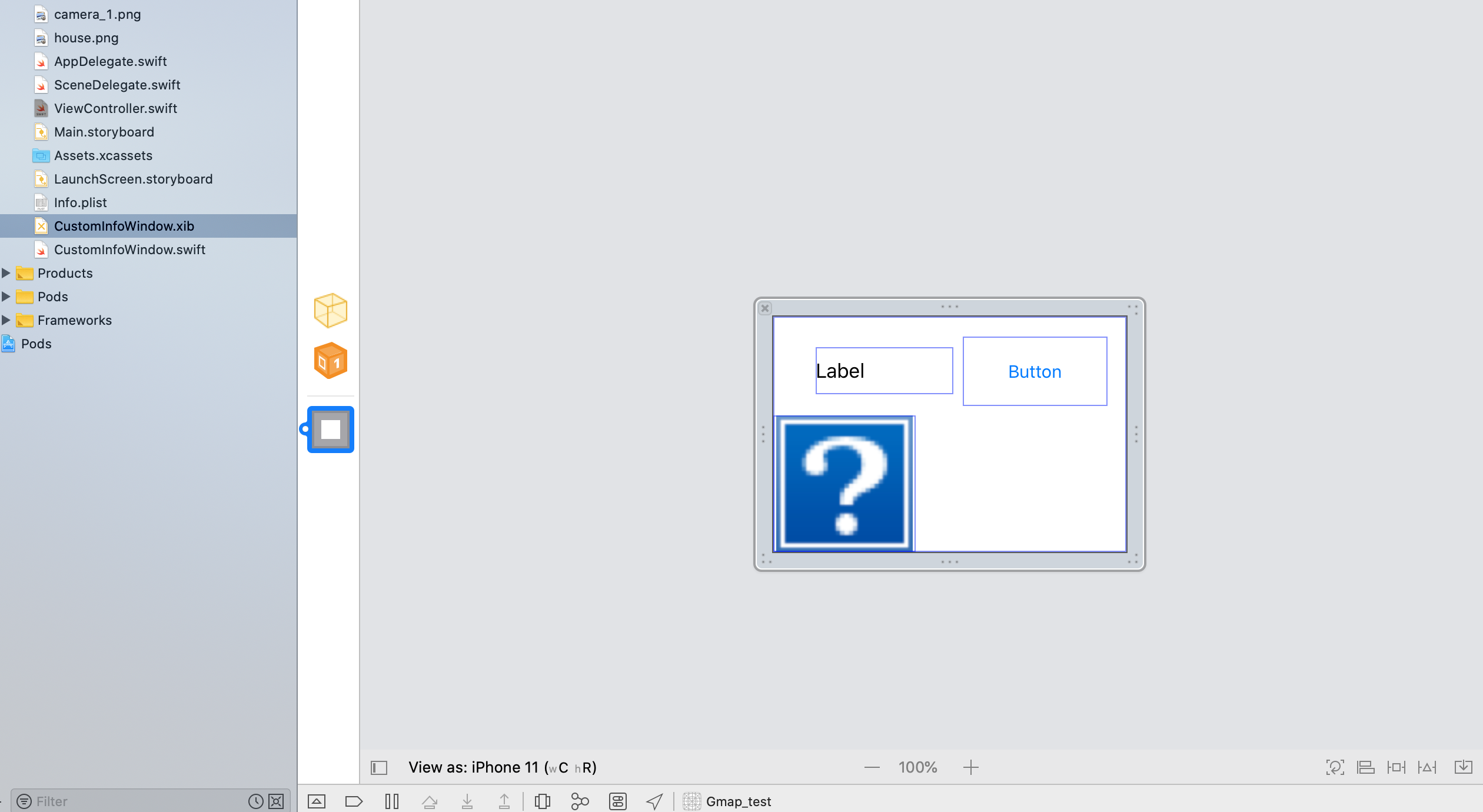
おわり
次はgeoJsonについて書こうと思う
ありがとうございました。