3.4以前では、型付をするのにas assertionでLiteralTypeを使う必要があり面倒でした。が、TS3.4から登場したas const
によってreduxの型付はとても手軽になりました。
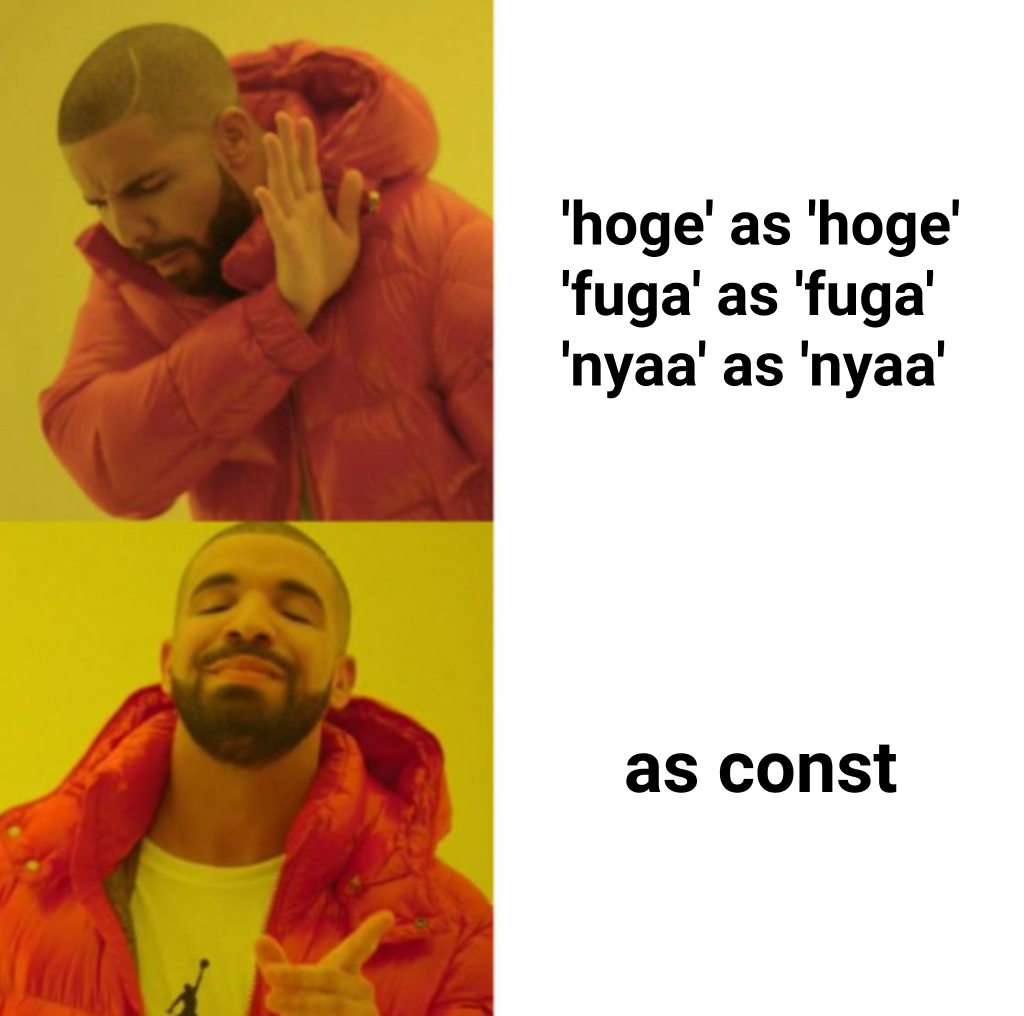
まずActionCreatorを作ります。このときtype属性にas const
を付けるよりオブジェクトにas const
を付ける方がすべての属性がreadonlyになるのでオススメします。
const increment = (payload: number) =>
({
type: 'increment',
payload,
} as const)
Action型を作るのは以下のような型定義が必要になります。やっていることは単純でそれぞれのActionCreator
から ReturnType
で戻り値の型を取り出しそのUnion型を作ります。AnyAction
は必須ではないですが、 type
プロパティを必ず含んでいることを保証することができます。
import { AnyAction } from 'redux'
export type ActionsType<ActionCreators extends object> = {
[Key in keyof ActionCreators]: ActionCreators[Key] extends (
...args: any[]
) => AnyAction
? ReturnType<ActionCreators[Key]>
: never
}
export type ActionType<
ActionCreators extends object,
Actions = ActionsType<ActionCreators>
> = { [Key in keyof Actions]: Actions[Key] }[keyof Actions]
使い方としては、as const
付きでActionCreatorを作成し、ActionType<typeof actions>
でAction型を作成できます。
export const increment = (payload: number) =>
({
type: 'increment',
payload,
} as const)
export const decrement = (payload: number) =>
({
type: 'decrement',
payload,
} as const)
export const typo = (payload: string) =>
({
// typo...
typo: 'typo',
payload,
} as const)
import { ActionType } from './redux-actions-type'
import * as actions from './actions'
type Action = ActionType<typeof actions>
/**
* type Action = {
* type: "increment";
* payload: number;
* } | {
* type: "decrement";
* payload: number;
* }
*/
type
プロパティだけでなく、payloadが正しく推論されていることがわかります。
また、type
プロパティがないものはnever
型になるので、Action型には現れることはないので安心して使えます。
あとは、普通にreducer
などで使うだけです。
import { Action } from './types'
type State = {
count: number
}
export const reducer = (state: State = { count: 0 }, action: Action): State => {
switch (action.type) {
case 'increment':
return { count: state.count + action.payload }
case 'decrement':
// 主題とはずれるが戻り値のStateを省略するとこの余計な`hoge`プロパティを検知できない。
// reduxのReducer型を使っても同じように検知されないので注意
// return { count: state.count - action.payload, hoge: 1 }
return { count: state.count - action.payload }
// Type Error...
// case 'typo':
// return { count: action.payload }
default:
return state
}
}
また、Action
はreducerごとに別々の型にするのではなく、プロジェクトで一つの型にした方が取り回しが楽です。
毎回この定義を書くは面倒なので、ライブラリからインストールできます。(スターするのはよい選択肢です🤭)
akameco/redux-actions-type: easy typing of redux action
$ npm install redux-actions-type
or
$ yarn add redux-actions-type
import { ActionType } from 'redux-actions-type'
import * as actions from './actions'
export type Action = ActionType<typeof actions>
また付属でActions
という型をexportできます。
これは個別のAction型を取れるので使う必要があれば(あまりそういうシーンはありませんが)使うことができます。たいていはActionCreater自身のReturnTypeで済むでしょう。
import { ActionsType } from 'redux-actions-type'
import * as actions from './actions'
export type Actions = ActionsType<typeof actions>
/**
* type IncrementAction = {
* type: "increment";
* payload: number;
* }
*/
type IncrementAction = Actions['increment']
TypeScriptの型非常に便利でいいですね。これからの進化にも期待です。
TypeScript 3.4 pic.twitter.com/wY45XfZPV2
— あかめ@孤独に歩め。悪をなさず、求めるところは少なく。林の中の象のように.js (@akameco) April 21, 2019
akameco/redux-actions-type: easy typing of redux action https://t.co/HpCKrmA84i 型のない環境から手軽にreduxのActionに型付けるところまで持っていくやつです pic.twitter.com/Hlup7e8Jjt
— あかめ@孤独に歩め。悪をなさず、求めるところは少なく。林の中の象のように.js (@akameco) March 14, 2019