##LeetCodeとは
- __実際に出題された技術テスト__を解くことができるサイト
- カテゴリー(algo/database/system design...) && 難易度別などに分けられた1000近くの問題
- Go/Scala/Kotlinなど多数の言語が使用可能
- GAFAなどから実際に出題された問題を掲載
- サブスクリプションで企業別の問題が解ける(テック界の赤本!?)
- モバイル版もあり(iOS: Leetcode clientなど)
- 詳しい問題の解説など、魅力満載
- English or Chinese!
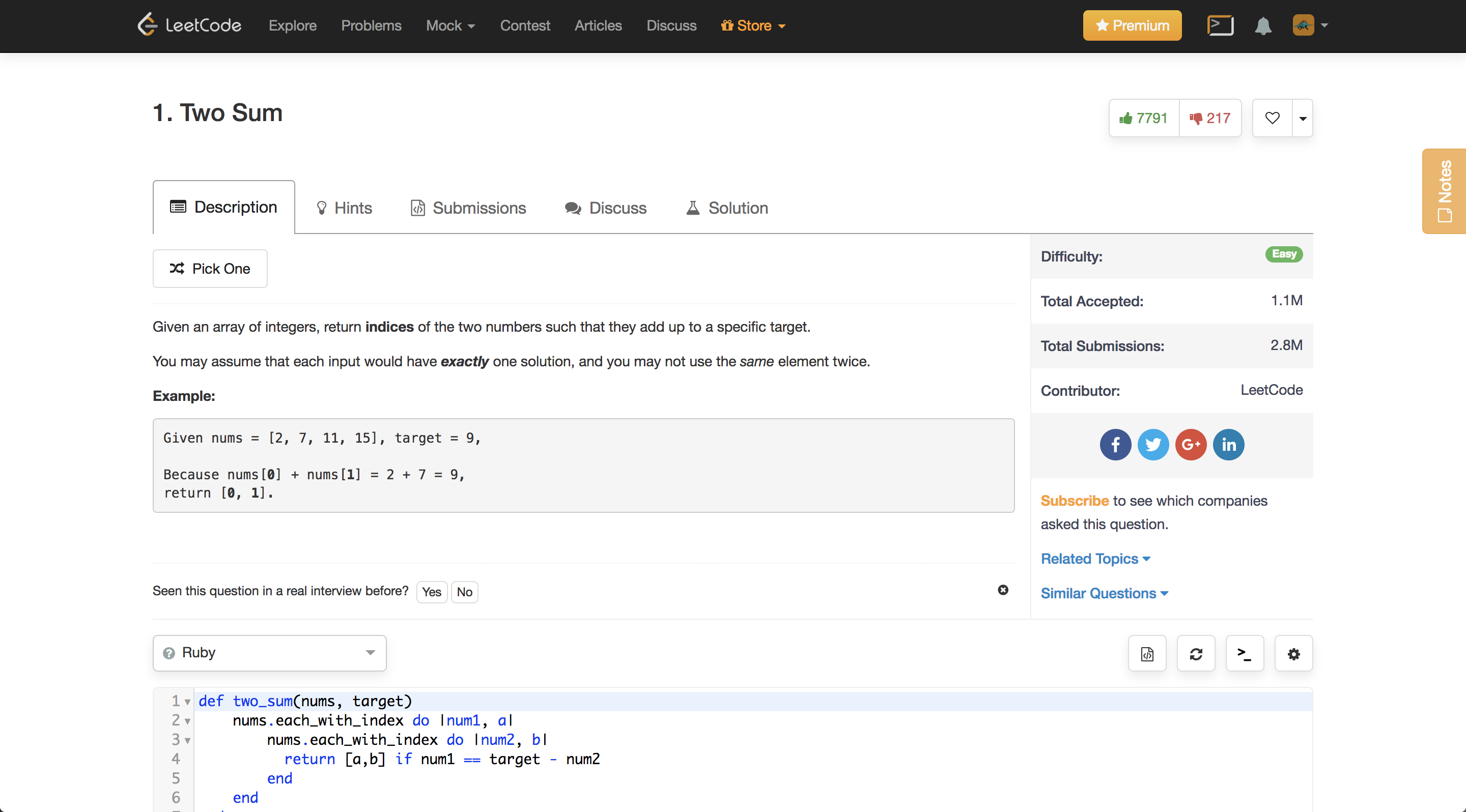
###Tips
問題数が多いのですが、Problems -> Lists -> __Top 100 Liked Questions__でソートする事ができていいです。本家の解答が準備されていない問題もありますが、Youtube等で検索すればヒットするものが多いです。
注意: 下記回答は不完全なものを含みます。
#1. TwoSum (easy)
Question:
Given an array of integers, return indices of the two numbers such that they add up to a specific target. You may assume that each input would have exactly one solution, and you may not use the same element twice.
Example:
Given nums = [2, 7, 11, 15], target = 9,
nums[a] + nums[b] = 2 + 7 = target,
return [0, 1].
def two_sum(nums, target)
nums.each_with_index do |num1, a|
nums.each_with_index do |num2, b|
return [a,b] if num1 + num2 == target
end
end
return "Cannot find two_sum"
end
puts two_sum([2, 6, 11, 15], 9)
#7. Reverse Integer (easy)
Question:
Given a 32-bit signed integer, reverse digits of an integer.
Example:
Input: 123
Output: 321
Input: -123
Output: -321
Input: 120
Output: 21
# ignore solution for the case: 32bit
def reverse(x)
a = x.to_s.chars
b = []
i = -1
while i >= -a.length
b.push(a[i])
i -= 1
end
if(x > 0)
return b.join().to_i
else(x < 0)
return -b.join().to_i
end
end
#412. Fizz Buzz (easy)
Question:
Write a program that outputs the string representation of numbers from 1 to n.
But for multiples of three it should output “Fizz” instead of the number and for the multiples of five output “Buzz”.
For numbers which are multiples of both three and five output “FizzBuzz”.
Example:
n = 15,
Return:
[
"1",
"2",
"Fizz",
"4",
"Buzz",
"Fizz",
"7",
"8",
"Fizz",
"Buzz",
"11",
"Fizz",
"13",
"14",
"FizzBuzz"
]
def FizzBuzz(n)
i = 0
n.times do |i|
i += 1
if (i % 3 == 0 && i % 5 == 0)
puts "FizzBuzz"
elsif (i % 3 == 0)
puts "Fizz"
elsif (i % 5 == 0)
puts "Buzz"
else
puts i
end
end
end
#136. Single Number (easy)
Question:
Given a non-empty array of integers, every element appears twice except for one. Find that single one.
Example:
Input: [2,2,1]
Output: 1
Input: [4,1,2,1,2]
Output: 4
def single_number(nums)
i = 0
a = []
while i < nums.length
if (a.include?(nums[i]))
a.delete(nums[i])
else
a.push(nums[i])
end
i += 1
end
puts a
end
#139. Word Break (medium)
Question:
Given a non-empty string s and a dictionary wordDict containing a list of non-empty words, determine if s can be segmented into a space-separated sequence of one or more dictionary words.
Example:
Example 1:
Input: s = "leetcode", wordDict = ["leet", "code"]
Output: true
Input: s = "applepenapple", wordDict = ["apple", "pen"]
Output: true
Input: s = "catsandog", wordDict = ["cats", "dog", "sand", "and", "cat"]
Output: false
#11 / 36 test cases passed.
def word_break(s, word_dict)
a = s.to_s.chars
b = []
i = 0
while i < s.length
b.push(a[0..i].join(''))
b.push(a[i+1..s.length].join(''))
i += 1
end
if (b.include?(word_dict[0] && word_dict[1]))
return true
end
end
##Ruby Basics Memo
####loop
#while
x = 0
while x<40
x+=1 #can't use ++,--
if (x%2) != 0
puts x
end
end
#for_in
nums = [21,54,32,6,4,23,5]
i = 0
for num in nums
i+=1
puts "##{i} is #{num}"
end
#each
nums.each do |num| #or (0..nums.length).each
puts "#{num} by another way"
end
#multi loop
array = [2, 7, 11, 15]
target = 9
array.each do |arr1, a|
array.each do |arr2, b|
return puts "#{arr1} and #{arr2}" if arr1 + arr2 == target
end
end
####function
x = 25
def tow_nums(nums)
x = 10
return (nums[0] + nums[1]).to_s + " and the x is #{x}" # return x=10
end
puts tow_nums([23,234])
####class
class Pokemon
attr_accessor :name, :hp, :attack
def initialize(name, hp, attack)
@name = name; @hp = hp; @attack = attack;
end
def bark
return "bowowow"
end
end
pikachu = Pokemon.new("pika","100","50")
puts pikachu.name #pika
puts pikachu.bark() #bowowow