Flipper
Facebookが開発しているAndroid/iOSアプリのデータの参照等が行える便利ツール
これまでもAndroidであれば Stetho や Hyperion-Androidなどのデバッグツールがありましたが、開発中にサクッと色々なログを見れたりする点ではFlipperだと良さそうな気がします またプラグインを追加でき拡張できたりして使い勝手が良さそうな印象です。
クライアントツール
Mac, Linux, Windows用の専用クライアントを使用してログを確認します。
環境構築
扱いたいアプリの AndroidManifest.xml
に以下を追加
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.ACCESS_WIFI_STATE" />
※ Build Variants が debug の時のみ有効にしたい場合などは debug/AndroidManifest.xml
に追加する等する
app/build.gradle
に依存ライブラリを追加
repositories {
jcenter()
}
dependencies {
// Build Variants が debug の時のみ有効
debugImplementation 'com.facebook.flipper:flipper:0.25.0'
debugImplementation 'com.facebook.soloader:soloader:0.8.0'
// Build Variants が release の時のみ有効
releaseImplementation 'com.facebook.flipper:flipper-noop:0.25.0'
}
Applicationを継承したカスタムのApplicationのonCreate内で以下を追加する
SoLoader.init(this,false)
if(BuildConfig.DEBUG && FlipperUtils.shouldEnableFlipper(this)){
val client = AndroidFlipperClient.getInstance(this).apply {
// pluginを追加する場合ここに追加していく
addPlugin(DatabasesFlipperPlugin(this@RootApplication))
}
client.start()
}
あとはアプリを実行して、ダウンロードしたクライアントツールを使って確認すると
以下の様にログやデータが確認出来ます。
クライアントツールの他機能として、プラグイン追加しない状態だと
- CPUの情報
- Crash Reporter のログ
- Logs
- スクリーンキャプチャ
- 動画
が使える様でした
プラグイン
Layout Inspector
Viewの階層の詳細が見れます。
if(BuildConfig.DEBUG && FlipperUtils.shouldEnableFlipper(this)){
val client = AndroidFlipperClient.getInstance(this).apply {
....
// Layout Inspector
val descriptorMapping = DescriptorMapping.withDefaults()
addPlugin(InspectorFlipperPlugin(this@RootApplication, descriptorMapping))
}
client.start()
}
addPluginに上記を追加してアプリを起動すると Layout
項目が増えていて
Viewの状態を確認できます。
Network
OkHttpClientのInterceptorにFlipperOkhttpInterceptorを設定することで通信ログを見れる様になります。
こちらは事前に以下のdependenciesを追加する必要があります。
debugImplementation 'com.facebook.flipper:flipper-network-plugin:0.25.0'
if(BuildConfig.DEBUG && FlipperUtils.shouldEnableFlipper(this)){
val client = AndroidFlipperClient.getInstance(this).apply {
....
// Network
val networkPlugin = NetworkFlipperPlugin()
addPlugin(networkPlugin)
}
client.start()
}
Shared Preferences
Shared Preferencesの確認や、変更等が行えます。
また、変更された内容はCHANGELOGとして確認できます。
if(BuildConfig.DEBUG && FlipperUtils.shouldEnableFlipper(this)){
val client = AndroidFlipperClient.getInstance(this).apply {
....
// Shared Preferences
addPlugin(SharedPreferencesFlipperPlugin(this@RootApplication, "shared_pref"))
}
client.start()
}
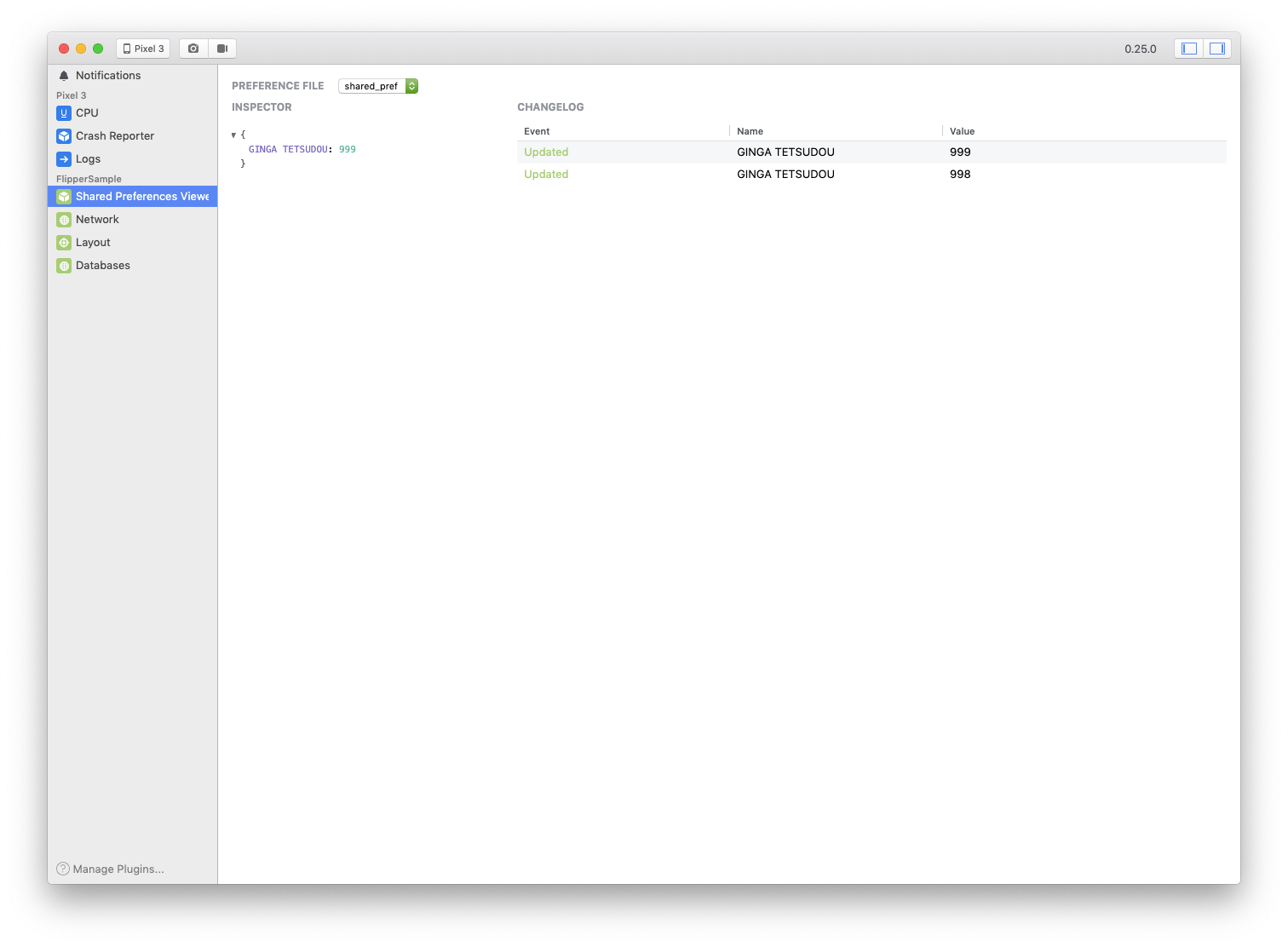
LeakCanary
メモリリーク検出の LeakCanary用のプラグインもあります。
こちらも事前に以下をdependencisに追加。
debugImplementation 'com.facebook.flipper:flipper-leakcanary-plugin:0.25.0'
debugImplementation 'com.squareup.leakcanary:leakcanary-android:1.6.3'
releaseImplementation 'com.squareup.leakcanary:leakcanary-android-no-op:1.6.3'
if(BuildConfig.DEBUG && FlipperUtils.shouldEnableFlipper(this)){
val client = AndroidFlipperClient.getInstance(this).apply {
....
// LeakCanary
addPlugin(LeakCanaryFlipperPlugin())
}
client.start()
}
実際に使う場合、RecordLeakService
をlistenerServiceに登録する
val refWatcher = LeakCanary.refWatcher(this)
.listenerServiceClass(RecordLeakService::class.java)
.buildAndInstall()
refWatcher.watch(this)
<service android:name="com.facebook.flipper.plugins.leakcanary.RecordLeakService" />
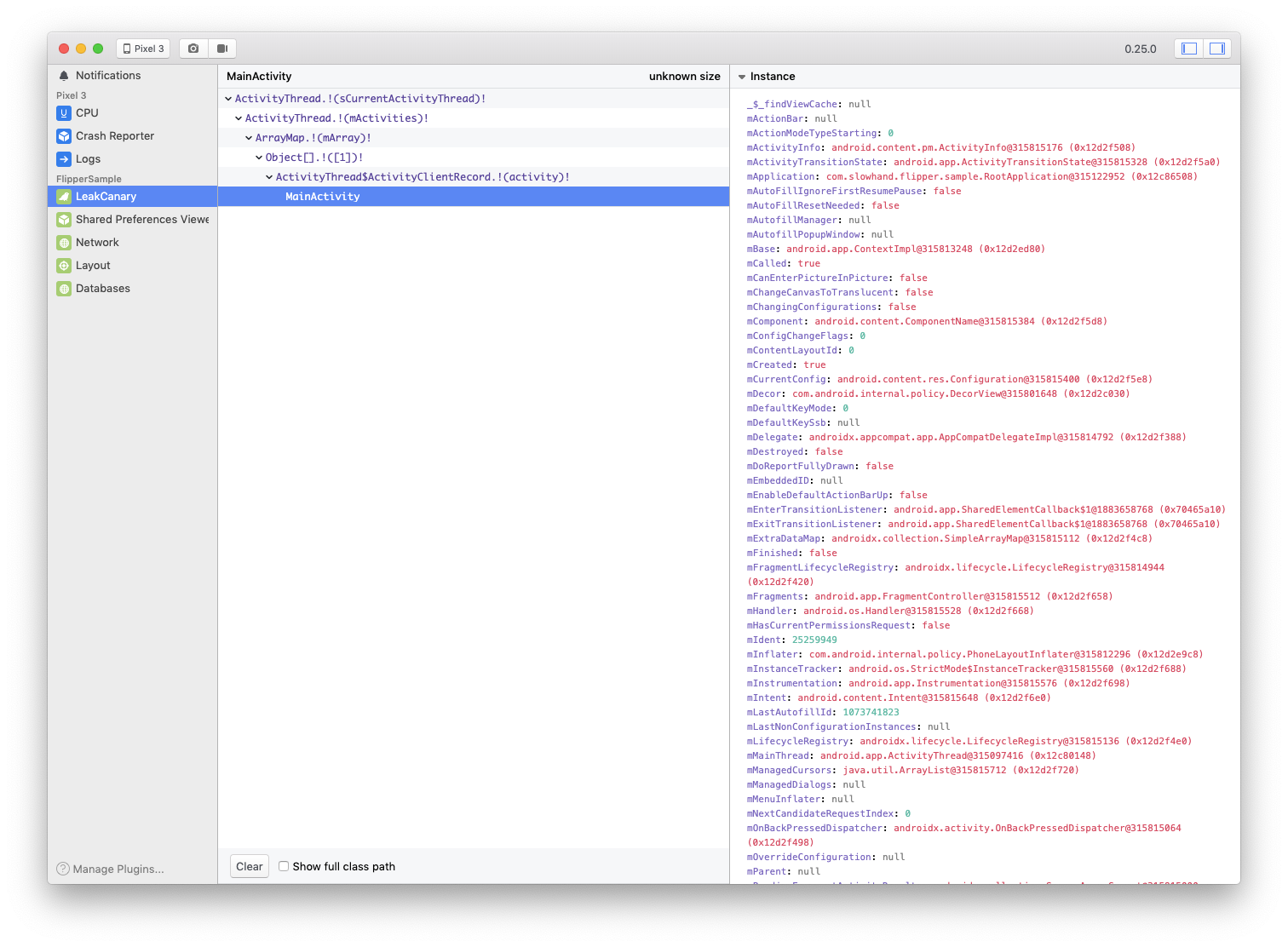
Database
テーブルの中身を確認できたり、SQLが使えたり、テーブルの詳細の確認などができます。
※今回はroomを使用して実装しました。
if(BuildConfig.DEBUG && FlipperUtils.shouldEnableFlipper(this)){
val client = AndroidFlipperClient.getInstance(this).apply {
addPlugin(DatabasesFlipperPlugin(this@RootApplication))
}
client.start()
}
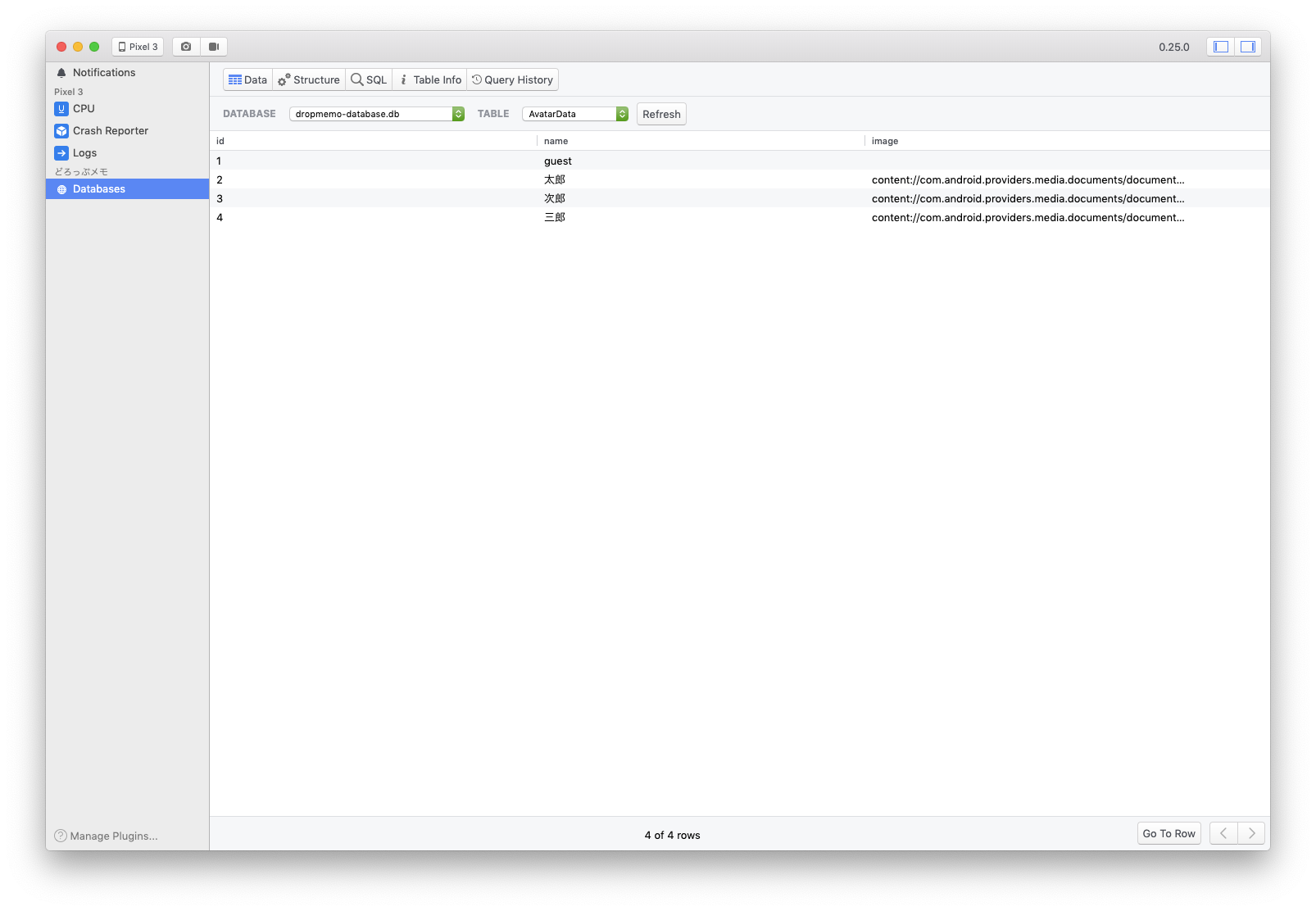