UICircularProgressRingとは?
↓ こちらを見れば一目瞭然ですが、よく見かける丸型のProgressを簡単に実現できるライブラリです。
環境構築
UICircularProgressRing
自体はCocoaPods
やCarthage
でも導入できます。
今回はCarthage
で導入します。
まずは以下の内容でCartfile
を作成。
github "luispadron/UICircularProgressRing"
次に以下コマンドで手元にダウンロード&ビルドします。
$ carthage update --platform iOS
Xcodeに戻ってTargetsのGeneralの「Framework, Libraries, and Embedded Content」に
./Carthage/Build/iOS/UICircularProgressRing.framework
を追加。
次に「Build Phases」で新規に「New Run Script Phases」を追加し
/usr/local/bin/carthage copy-frameworks
上記を設定し、Input Files
に $(SRCROOT)/Carthage/Build/iOS/UICircularProgressRing.framework
を設定します。
ここまででライブラリの導入は完了になります。
実装
簡単なサンプル
README に乗っていた単純なサンプルを少し修正して試してみます。
import UIKit
import UICircularProgressRing
class ViewController: UIViewController {
private let progressRing = UICircularProgressRing()
override func viewDidLoad() {
super.viewDidLoad()
progressRing.maxValue = 50
progressRing.frame = CGRect(x: 0, y: 0, width: 200, height: 200)
progressRing.center = self.view.center
self.view.addSubview(progressRing)
}
// Viewのレイアウトが決定した後じゃないとアニメーションされない
override func viewDidAppear(_ animated: Bool) {
super.viewDidAppear(animated)
progressRing.startProgress(to: 50, duration: 2.0) {
print("Done animating!")
}
}
}
UICircularRingStyle
UICircularProgressRing
で設定できるスタイルとして以下があります。
-
inside (デフォルト ↑と同じ)
中のテキストを非表示にする
以下のプロパティ shouldShowValueText
を false
に設定すれば非表示になります。
progressRing.shouldShowValueText = false
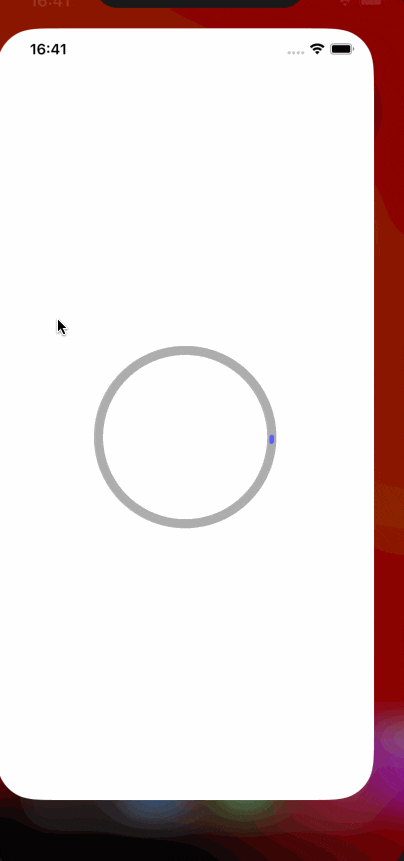
リングカラーを設定する
progressRing.outerRingColor = UIColor.red
progressRing.innerRingColor = UIColor.blue
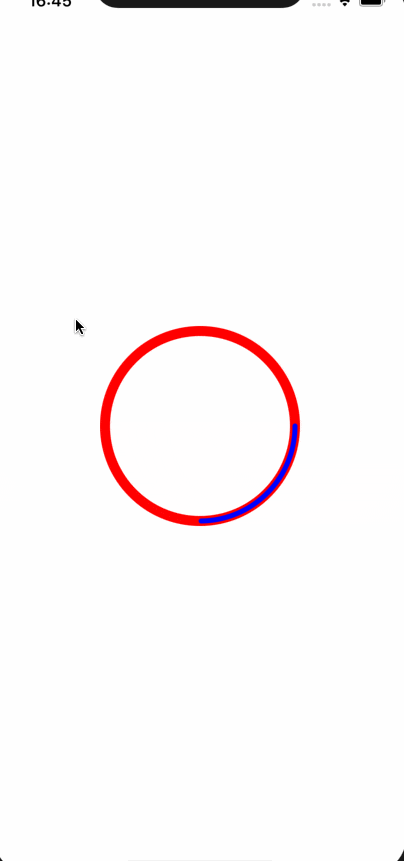
開始アングルを設定する
startAngle
で設定可能。デフォルトは 0
で設定されています。
UICircularTimerRing
Timerを設定できる様にしたクラスです。
import UIKit
import UICircularProgressRing
class ViewController: UIViewController {
private let timerRing = UICircularTimerRing()
override func viewDidLoad() {
super.viewDidLoad()
timerRing.frame = CGRect(x: 0, y: 0, width: 200, height: 200)
timerRing.center = self.view.center
self.view.addSubview(timerRing)
}
override func viewDidAppear(_ animated: Bool) {
super.viewDidAppear(animated)
timerRing.startTimer(to: 10) { state in
print("state: \(state)")
}
}
}
↑のサンプルでは10秒後にリングが閉じる様に設定しています。