Android7.0(API Level24)からNotificationのグループを作成することができるようになりました。
Notificationのグループ作成方法を以下で確認していきます。
グループの作成
1.グループの子となるNotification作成時にsetGroup()メソッドを使用してグループの名前を設定する
2.グループの親となるNotification作成時にsetGroup()メソッドでグループ名を設定し、setGroupSummary()でtrueを渡す
val CHANNEL_ID = "channel_id"
val SUMMARY_ID = 0
val GROUP_KEY = "group_key"
fun createChildNotification(num :Int): Notification {
val childNotification = NotificationCompat.Builder(this@MainActivity, CHANNEL_ID)
.setSmallIcon(R.drawable.ic_stat_name)
.setContentTitle("Content Title $num")
.setContentText("Content Text $num")
.setStyle(NotificationCompat.BigTextStyle()
.setBigContentTitle("BigContentTitle $num")
.setSummaryText("summary text $num")
.bigText("Big Text $num"))
.setGroup(GROUP_KEY)
.build()
return childNotification;
}
fun addSummaryNotification() {
val childNotification1 = createChildNotification(1)
val childNotification = createChildNotification(2)
val summaryNotification = NotificationCompat.Builder(this@MainActivity, CHANNEL_ID)
.setContentTitle("Summary")
.setSmallIcon(R.drawable.ic_stat_name)
.setGroup(GROUP_KEY)
.setGroupSummary(true)
.build()
val notificationManager = NotificationManagerCompat.from(this)
notificationManager.notify(1, childNotification1)
notificationManager.notify(2, childNotification)
notificationManager.notify(SUMMARY_ID, summaryNotification)
}
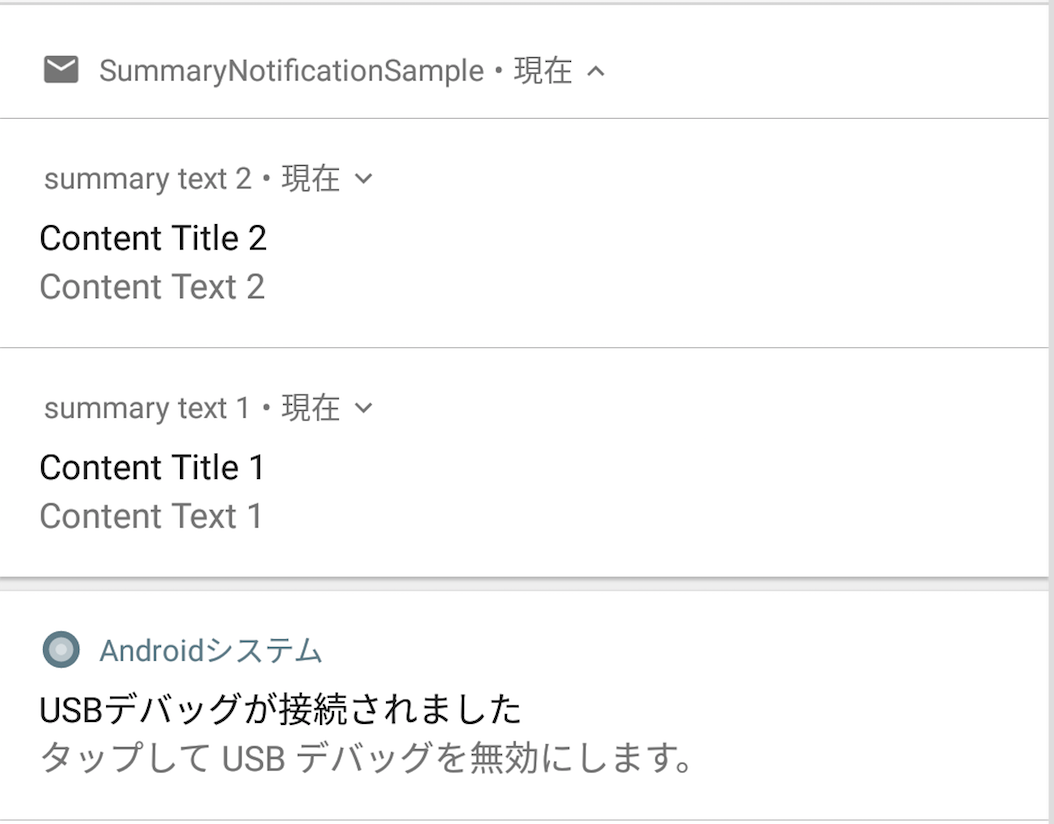
注意点
- 子となるNotificationが1つのときは、Notificationはグループ化して表示されません。
- 子は最大8個までしか表示されず、新しいNotificationから8個を表示します。
- 親のSummaryNotificationと子のSmallIconが異なる場合は、親と子両方にSmallIconが表示されます。
- Android7.0未満の端末ですと、Summaryのみ表示され子のNotificationが表示されません。
Notificationの自動グループ化
Android7.0以降ではNotificationのグループ化を設定しなくても、自動的にGroup化されます。
APIレベル26のソースコードで確認したところ、以下のクラスにてNotificationの自動グループ化の判定を行なっています。
com.android.server.notification.GroupHelper
自動的にグループ化する条件として、 AUTOGROUP_AT_COUNTが定義されており、同じパッケージ名のアプリが4つ以上のNotificationを表示しようとした時に、自動的にグループを作成します。
自動的に作成されたグループの親NotificationにはPendingIntentが設定されており、CATEGORY_LAUNCHERフラグが付与されているActivityが起動されます。
自動グループ化されたときのIntentでは問題がある場合、自分でグループ化し起動するActivityを指定する必要があります。
Gmailアプリではメールアカウントごとにグループ化されており、Summaryをタップしたときの動作はアカウントの受信ボックスを開くようになっています。
まとめ
- Android7.0未満の端末ではグループ設定していると、親Notificationしか表示されない
- 子の表示は新しい順に8個までで、古いものは隠れている
- Notificationは自動的にグループ化されるが、親をタップしたときのIntentに注意
参考
https://developer.android.com/training/notify-user/group
https://material.io/design/platform-guidance/android-notifications.html