はじめに
Firebase Cloud Messaging (FCM)を利用して、サーバからスマホへのPush通知を試してみました。サーバからFirebase経由でPush通知を送信する方法を書いていきます。具体的には、以下の図の赤枠の範囲になります。
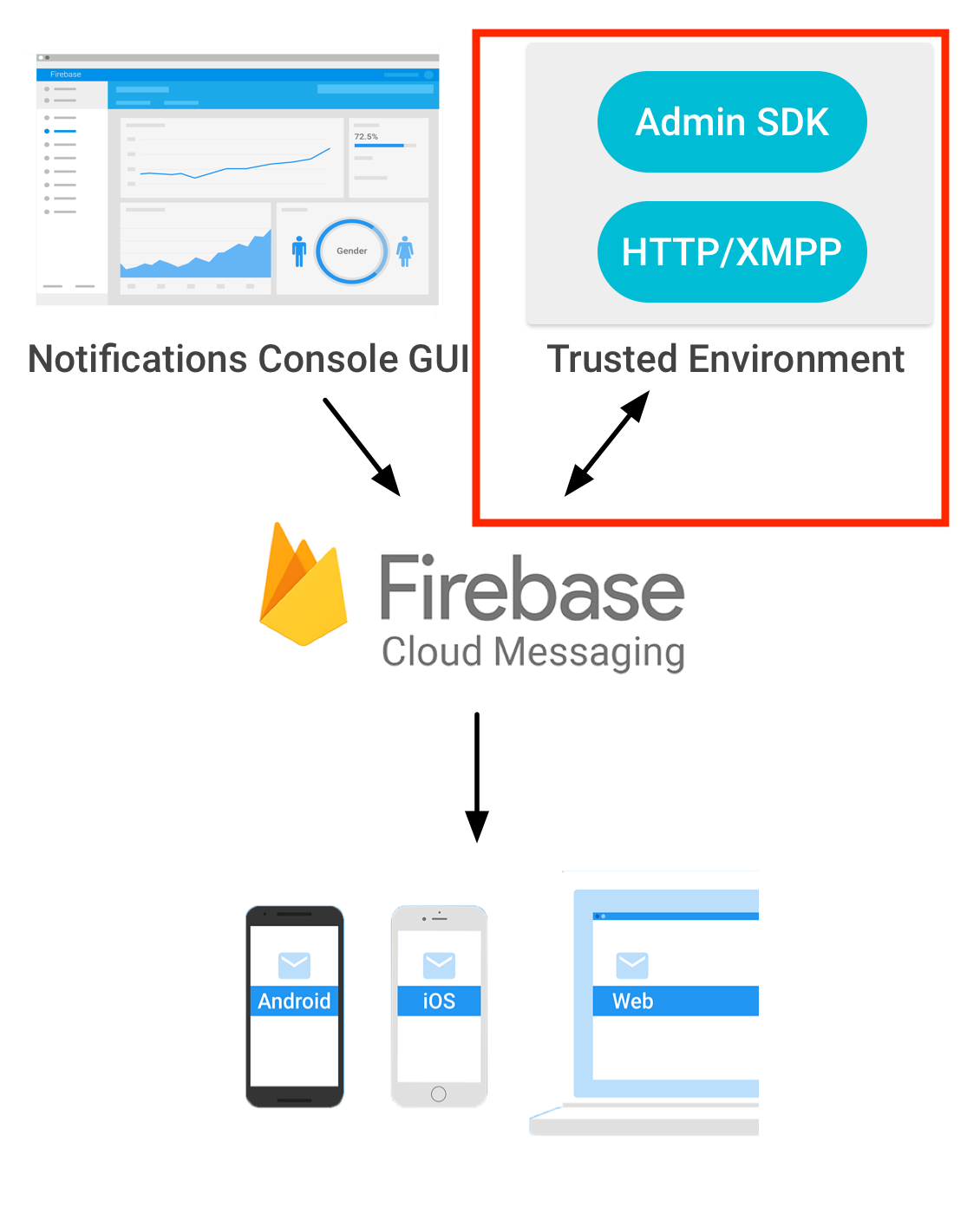
環境
- Python 3.7.3
準備
- Firebase Admin SDKをインストールします。
$ pip install firebase-admin
-
Firebase consoleからfirebase接続用の鍵ファイルを取得します。
-
以下の図のように、Firebase consoleの左上にある歯車マークを押して、プロジェクトの設定画面を開きます。
-
この画面から「サービスアカウント」のタブをクリックします。
-
「新しい秘密鍵の生成」ボタンを押すと、秘密鍵のファイルがダウンロードされます。
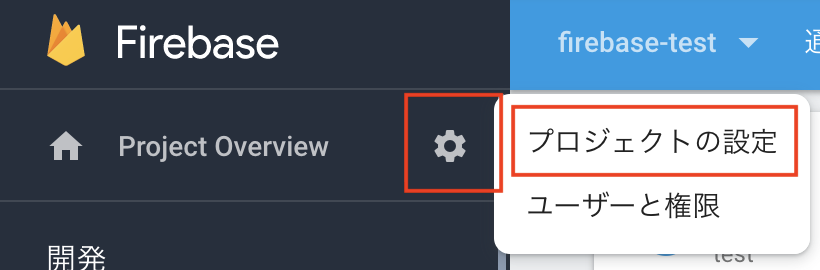
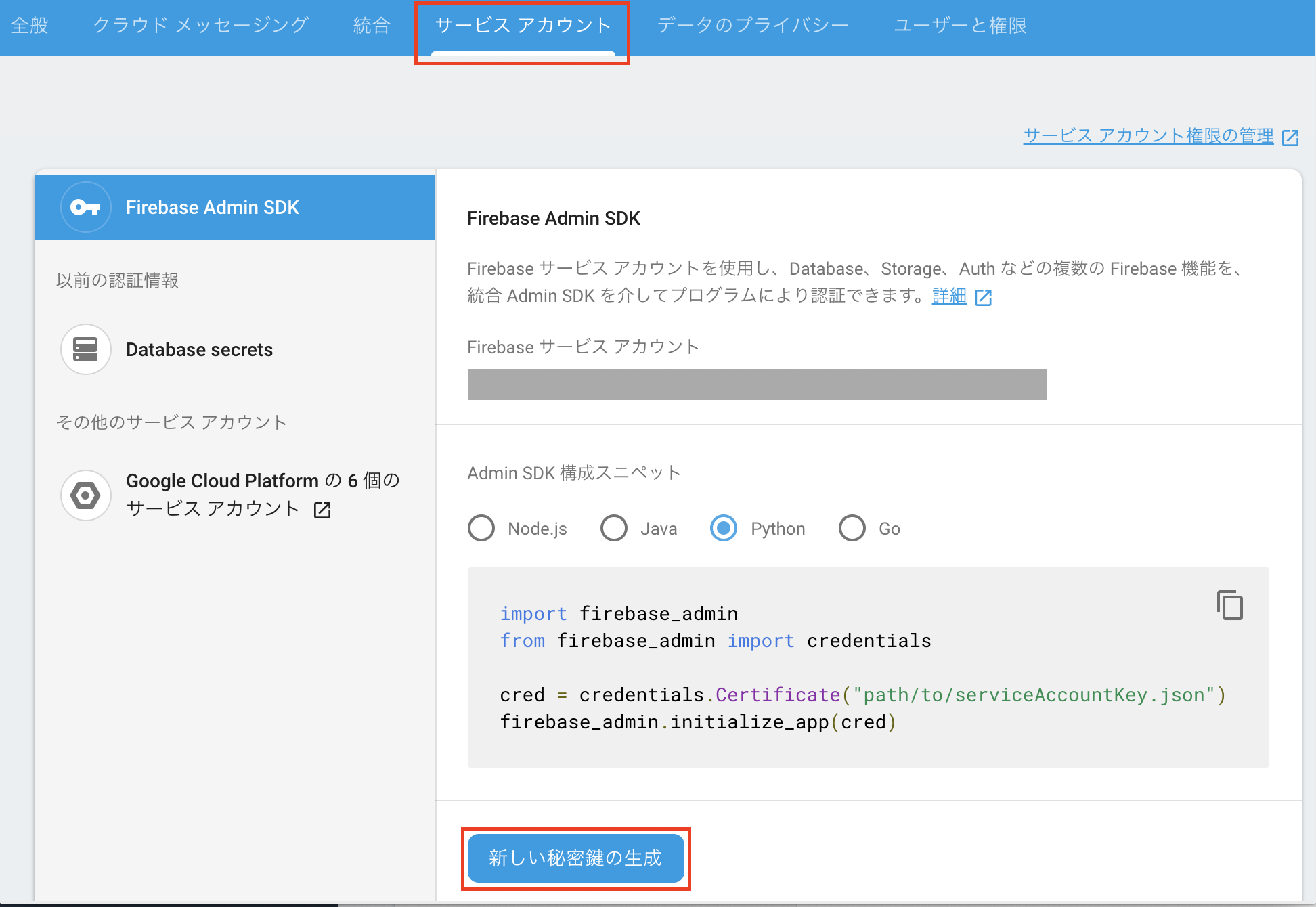
特定のスマホにPush通知
特定のスマホ(YOUR_REGISTRATION_TOKEN)へPush通知を送信します。
コードは以下です。path/to/serviceAccountKey.json
は、ダウンロードした秘密鍵のファイルを指定します。
import firebase_admin
from firebase_admin import credentials
from firebase_admin import messaging
cred = credentials.Certificate("path/to/serviceAccountKey.json")
firebase_admin.initialize_app(cred)
# This registration token comes from the client FCM SDKs.
registration_token = 'YOUR_REGISTRATION_TOKEN'
# See documentation on defining a message payload.
message = messaging.Message(
notification=messaging.Notification(
title='test server',
body='test server message',
),
token=registration_token,
)
# Send a message to the device corresponding to the provided
# registration token.
response = messaging.send(message)
# Response is a message ID string.
print('Successfully sent message:', response)
YOUR_REGISTRATION_TOKEN
は、iosの場合は、以下のSWIFTのコードで取得した端末ごとのトークン(result.token)になります。
InstanceID.instanceID().instanceID { (result, error) in
if let error = error {
print("Error fetching remote instance ID: \(error)")
} else if let result = result {
print("Remote instance ID token: \(result.token)")
self.instanceID.text = "Remote InstanceID token: \(result.token)"
}
}
トピックに参加しているスマホにPush通知
特定のトピックに参加している複数のスマホにPush通知する方法です。
例えば、"weather"のトピックに参加しているスマホにPush通知するときは、
メッセージの作成部分を以下のように変更します。
具体的には、token
の部分をtopic
に変更するだけです。
message = messaging.Message(
notification=messaging.Notification(
title='test server',
body='test server message',
),
topic='weather',
)
iosでトピックへの参加は、以下のようなコードで実現できます。
Messaging.messaging().subscribe(toTopic: "weather") { error in
print("Subscribed to weather topic")
}