はじめに
修士論文の研究で、Hexoskinを使いました。
ただ、Hexoskinの使い方についての記事は、Qiitaでも他のブログでも皆無だったので大変苦労しました。
そこで今回は、同じ辛酸を嘗める人を減らすべく、HexoskinおよびHexoskin APIの導入について記事を書きます。
Hexoskinとは
Carre Technologies(カナダ)製のセンサー付きのスマートシャツです。
価格は一式で約7万円。
着るだけで下記のデータをクラウド上に記録することができます。
- 心拍情報(心電図、心拍数、心拍間隔など)
- 呼吸情報(呼吸数、呼吸率、酸素消費量など)
- 活動情報(歩数、歩行率、加速度など)
Hexoskinの構成図
専用アプリ経由で、Hexoskin社のクラウドに逐次データを送信する構成です。
Hexoskinのデータを取得する
今回は、Hexoskinの初期設定から、Hexoskin APIによるクラウドからのデータ取得までをやってみましょう。
1. 初期設定
初期設定は、QUICK START(公式)を見ながら進めていってください。
重要なポイントは、
① ロガーを充電する
② アカウント登録・設定をする( https://my.hexoskin.com/en/signup )
③ モバイル端末に専用アプリをインストールする
④ Bluetoothのペアリング(ロガーとモバイル端末)設定をする
2. APIの準備
Hexoskin APIは、認可方式にOauth2(とOauth1)が採用されています。
https://api.hexoskin.com/docs/index.html#before-getting-started
また、HexoskinでOauth2の認証情報(IDとsecret key)を取得するためには、開発元にメールを送る必要があります。
https://www.hexoskin.com/pages/developers
なので、上記URLに記載されたメールアドレス宛に、以下のようなメールを送りましょう。
Hello,
Please send me API keys, I'm eager to start developing an API to access Hexoskin data.
My account information to use API key is as follows.
******
Account email:
First name:
Last name:
Time zone:
******
(▲ 1.の②(https://my.hexoskin.com)で登録した情報を書く)
And just in case you need, additional information are described below.
******
My organization is:
Our project name is:
Our project summary:
******
(▲ APIの使用用途について(決まっていれば)書く)
Please let me know if there is missing information.
If not, please send me the API key.
Thanks a lot,
■-----------------------------------
(署名など)
-----------------------------------■
しばらくすると、こんな返信が来ます。
(Your Name),
Your OAuth2 information is:
client id: **********
secret: **********
You can update your client (to add redirect URIs, for example) using the API at this endpoint:
https://api.hexoskin.com/api/oauthclient/359/
OAuth2 is our preferred method of authentication. There's a section on our OAuth2 implementation here that I recommend you start with:
https://api.hexoskin.com/docs/page/oauth2/
We have a developer group here which we watch closely:
https://groups.google.com/forum/#!forum/hexoskin-developer
The rest of the API documentation is here:
https://api.hexoskin.com/docs/
There's a Python client available here:
https://bitbucket.org/carre/hexoskin-api-python-client
Don't hesitate to post to the list if you need a hand with something. Happy coding!
認証情報以外にも、ドキュメントや開発者グループなどの公式情報を知らせてくれるので、とても丁寧ですね。
さて、ここまでできれば、APIの準備は完了です。
3. APIからデータを取得
ではいよいよ、Hexoskin API経由でクラウドからデータを取得します。
今回は、Hexoskin APIにアクセスするのに便利な公式のPythonクライアントを利用したソースコードを示します。
以下のリンクより、cloneまたはdownloadしておいてください。
- Pythonクライアント - https://github.com/lguerdan/HexoBio
また、この時点でHexoskin未使用の場合は、一度試着して、テストデータを作っておくと良いです。
実行環境(動作確認済み)
- OS: macOS High Sierra
- Python: 2.7.14
事前準備①: 外部ライブラリのインストール
以下のライブラリをインストールしておいてください。
$ pip install requests urllib numpy pandas jupyter
事前準備②: 認証情報などをまとめる
今回は、認証情報などを外部ファイルにまとめて管理します。
{
"HexAPIkey": "「2. APIの準備」で取得したclient id",
"HexAPIsecret": "「2. APIの準備」で取得したsecret",
"HexUserName": "「1.の②」で登録したEmail",
"HexPassword": "「1.の②」で登録したPassword"
}
ファイル構造
HexoBio
├── credentials.json
├── get_rrinterval.ipynb
├── HxApi2_0.py
└── ...
実行手順
ライブラリのインストールやファイル構造で既にお気づきかと思いますが、今回のコーディングにはJupyter Notebookを用いました。
例として、**"rrinterval(心拍間隔)"**のデータフレームを出力するまでのコードを示します。
# -*- coding: utf-8 -*-
import HxApi2_0
import hexoskin.client
import urllib, json
import numpy as np
import pandas as pd
%matplotlib inline
# JSONから認証情報を読み込む
with open("credentials.json", "r") as f:
credentials = json.load(f)
username = credentials["HexUserName"]
password = credentials["HexPassword"]
publicKey = credentials["HexAPIkey"]
privateKey = credentials["HexAPIsecret"]
# userIDを取得
userURL = "https://" + username + ":" + password + "@api.hexoskin.com/api/user/"
userInfo = urllib.urlopen(userURL).read()
userInfoJSON = json.loads(userInfo)
userID = userInfoJSON["objects"][0]["id"]
# OAuth2.0認証を行なう
auth = HxApi2_0.SessionInfo(publicKey=publicKey,privateKey=privateKey,username=username,password=password)
# 記録したActivityのIDを取得
records = HxApi2_0.getRecordList(auth, limit = "2000")
all_recordID = [record['id'] for record in records]
last_recordID = all_recordID[len(all_recordID)-1] # 直近のActivityのID
# 直近のActivityのデータを取得
dataset = HxApi2_0.getRecordData(auth,recordID=last_recordID)
# rrinterval(心拍間隔)を選択
target = "rrinterval"
# numpyで配列を作成
np_data = np.array(dataset[target])
print np_data
# pandasのデータフレームを作成
df_data = pd.DataFrame(np_data)
# データフレームを整形
df_data = df_data.rename(columns={0: "ts", 1: "value"}) # カラムを設定
df_data = df_data.set_index("ts") # インデックスを設定
df_data = df_data.astype(float) # floatに型変換
df_data.drop(df_data.index[df_data["value"] == 0], inplace=True) # 値が0の行を削除
# 冒頭5行を出力
df_data.head(5)
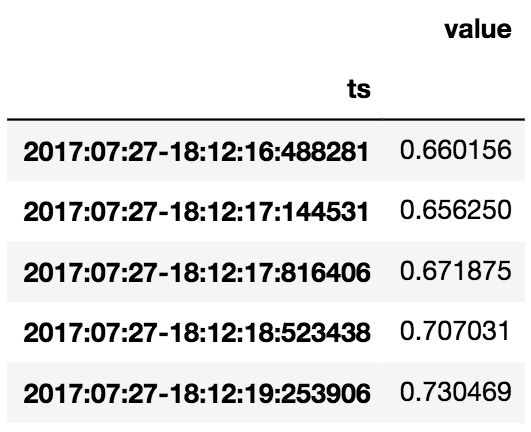
ちなみに、プロットした結果はこんな感じです。
# データをプロット
df_data.plot()
また、**rrinterval(心拍間隔)**以外を取得したい場合は、Pythonクライアントに入っているHxApi2_0.py
の以下の部分(20~40行目)で、対応するコメントアウトを外してください。
# Datatypes definitions
if MODEL == 'Hexoskin':
raw_datatypes = {#'acc':[4145,4146,4147],
#'ecg':[4113],
#'resp':[4129,4130]
}
datatypes = {#'activity':[49],
#'cadence':[53],
#'heartrate':[19],
#'minuteventilation':[36],
#'vt':[37],
#'breathingrate':[33],
#'hr_quality':[1000],
#'br_quality':[1001],
#'inspiration':[34],
#'expiration':[35],
#'batt':[247],
#'step':[52],
'rrinterval':[18],
#'qrs':[22],
}
これで、外したコメントアウトに対応するデータをtarget = "rrinterval"
の右辺と差し替えれば、そのデータを取得することができるようになります。
各データの詳細については、以下のドキュメントでご確認ください。
- Hexostore API Documentation - Data type
https://api.hexoskin.com/docs/resource/datatype/
終わりに
今回は、HexoskinおよびHexoskin APIを手早く試す方法についてまとめました。
この記事が少しでもHexoskin入門者の手助けになれば嬉しいです。
ちなみに現段階では、以下の3つが唯一かつ最も有力な学習リソースです。(2018/03/01 現在)
APIをもっと使いこなしたい場合は、こちらを参考にしてみてください。