はじめに
最近困っていることがあって、
プログラミングに熱中していたら
水分をとることを忘れるんですよね。
それを対策するために、
水分補給を促すアプリを作ってみました!
こんなアプリを作りました
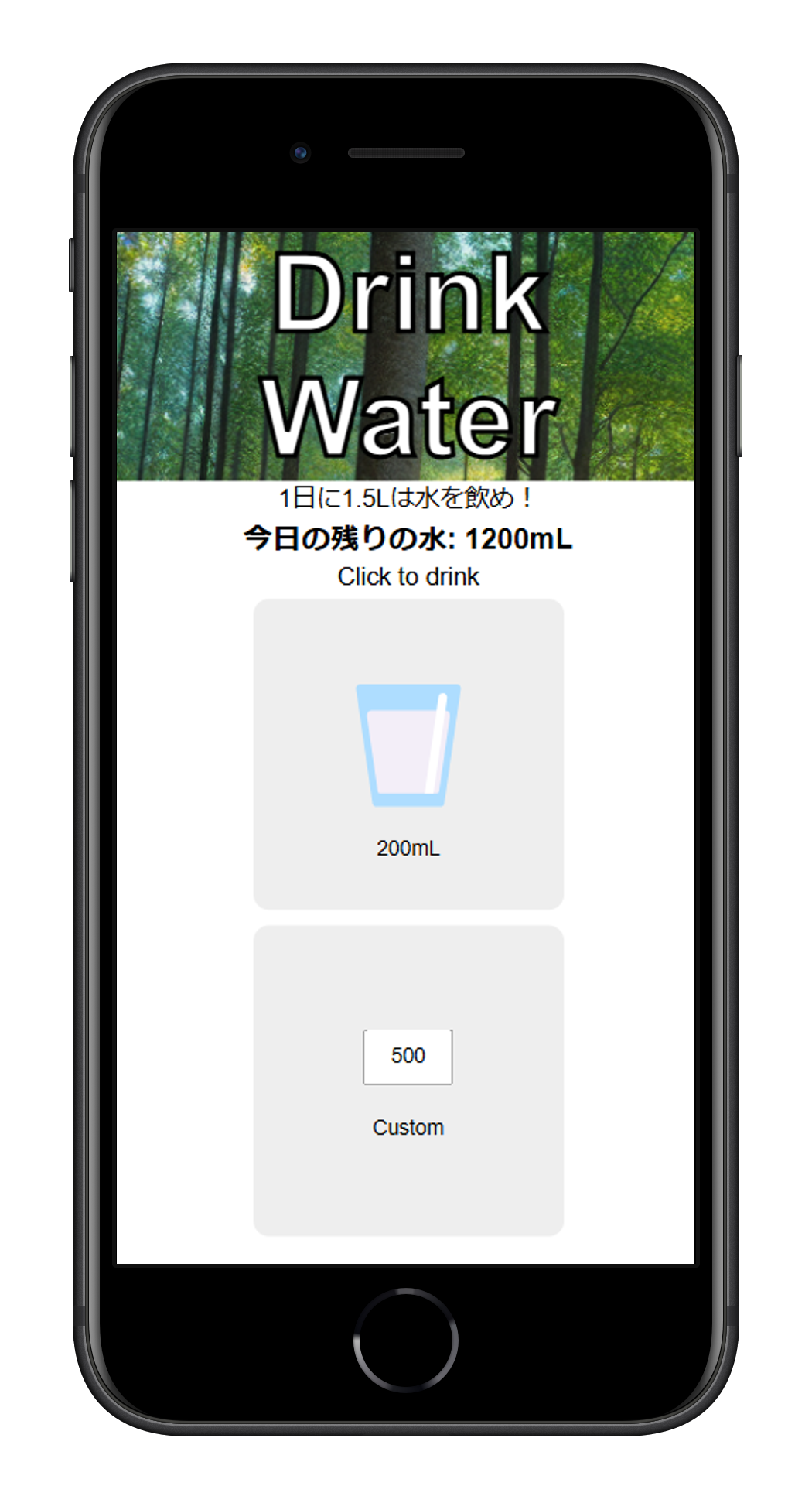
自分で作った理由
既存のアプリに頼るのもアリですが、
作るのもそれはそれで楽しそうなのでやってみました!
どういう構造?
クライアントは、開発環境がWindowsしかないので
PWAでアプリっぽく設計しました。
サーバーはVercel上のFlaskで。
コード
index.html
index.html
<!DOCTYPE html>
<html lang="ja-jp">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Drink Water</title>
<link rel="apple-touch-icon" href="/static/Icon1024.png">
<link rel="manifest" href="/manifest.json">
<style>
* {
font-family: "Arial", "メイリオ";
text-align: center;
margin: 0;
}
h1{
font-size: 70px;
color: white;
-webkit-text-stroke: 3px black;
}
.title{
background-image: url("/static/Icon1024.png");
}
.contents {
display: flex;
justify-content: center;
flex-direction: column;
align-items: center;
}
button {
margin: 5px;
width: 200px;
height: 200px;
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
padding: 0;
background-color: #eee;
border: 0;
border-radius: 10px;
}
button:hover{
background-color: #ddd;
}
button span {
margin: 5px;
font-size: 80px;
margin-bottom: 10px;
}
button input {
margin: 5px;
width: 50px;
height: 30px;
text-align: center;
}
</style>
</head>
<body>
<div class="title"><h1>Drink Water</h1></div>
<p>1日に1.5Lは水を飲め!</p>
<h3 id="remaining-water-volume">今日の残りの水: 1500mL</h3>
<p>Click to drink</p>
<div class="contents">
<button onclick="drink(200);">
<span>🥛</span>
200mL
</button>
<button onclick="custom();">
<input type="number" value="500" step="10" id="volume" onclick="preventButtonClick(event)">
<span></span>
Custom
</button>
</div>
<script>
let water = 0
fetch("/volume")
.then(req => req.text())
.then(data => {
water = parseInt(data);
document.querySelector("#remaining-water-volume").textContent = `今日の残りの水: ${water}mL`;
});
function drink(volume){
water -= volume;
document.querySelector("#remaining-water-volume").textContent = `今日の残りの水: ${water}mL`;
fetch(`/drink?volume=${water}`);
};
function custom(){
drink(document.querySelector("#volume").value);
};
function preventButtonClick(event) {
event.stopPropagation();
};
</script>
</body>
</html>
CSS,Javascriptは一か所にまとめちゃいました。
index.py
# app.pyにしたかったけどVercelだからできない
import json
from flask import Flask,render_template,request, send_file
import datetime
app = Flask('__app__')
@app.route('/')
def index():
return render_template("index.html")
@app.route("/drink")
def drink():
today = datetime.datetime.now().strftime("%Y-%m-%d")
volume = request.args.get("volume")
try:
if volume:
histroy = json.load(open("histroy.json"))
if today in [h["date"] for h in histroy]:
for h in histroy:
if h["date"] == today:
h["volume"] = int(volume)
else:
histroy.append({"date":today,"volume":int(volume)})
json.dump(histroy,open("histroy.json","w"))
except:
return "Bad request",422
return "OK"
@app.route("/volume")
def volume():
today = datetime.datetime.now().strftime("%Y-%m-%d")
histroy = json.load(open("histroy.json"))
for h in histroy:
if h["date"] == today:
return str(h["volume"])
return str(1500)
@app.route("/manifest.json")
def manifest():
return send_file("manifest.json")
if __name__ == '__main__':
app.run(host="0.0.0.0",port=10000)
作った感想
- 意外と、作るのは簡単だったし楽しかった
- PWA対応は結構便利
需要があれば一般公開します
コメント、いいねしてくれると嬉しいです._.