こんな感じの普通のText Mesh Proで描画されたテキストを
テキストの装飾もそのまま反映されます(下線と取り消し線のみTextMeshProの実装の都合上未対応です)
2次元上で曲げる方法
奥行方向ではなく,ワードアートみたいなあくまでも2次元平面上で曲げたい,という場合はText Mesh Proのサンプルに動作するスクリプトが存在します.
TextMeshProをアセットストア(or Package Manager)からインポートし,Winodow -> TextMeshPro -> Import TMP Examples and Extras
からサンプルプロジェクトをダウンロードします.
Assets/TextMesh Pro/Examples & Extras/Scenes/25 - Sunny Days Example.unity
がそのサンプルで,開くと↓のようにテキストが曲がっている様子が見られると思います.
TextMeshProオブジェクトにアタッチされているWarpText.cs
というスクリプトが文字のメッシュを変形しています.
今回の奥行方向へ曲げる実装は,このスクリプトをベースに改造を行って作成したものです(大分変ってますが).
利用方法
利用したいプロジェクトにおいて,次のスクリプトをBendText.cs
という名前で保存します.
それを曲げたいTextMeshProのオブジェクトにアタッチするだけです.
スクリプト(長いので折り畳み)
using System;
using System.Linq;
using TMPro;
using UnityEngine;
namespace TweenableObject
{
[DefaultExecutionOrder(100)]
[ExecuteInEditMode]
[RequireComponent(typeof(TextMeshPro))]
public class BendText : MonoBehaviour
{
[SerializeField] private bool isActive;
[SerializeField] private bool flip;
[SerializeField] private float radius;
[SerializeField, Range(0, 360)] private float spread;
private RectTransform _rectTransform;
private TextMeshPro _textMeshPro;
private bool _isActive;
private bool _flip;
private float _lastRadius;
private float _lastSpread;
private Rect _lastRect;
private float _maxY;
private float _minY;
private void Awake()
{
_rectTransform = GetComponent<RectTransform>();
_textMeshPro = GetComponent<TextMeshPro>();
}
private void OnEnable()
{
ReCalculate();
}
private void OnDisable()
{
_textMeshPro.ForceMeshUpdate();
}
private void Update()
{
// EditModeでも動作するようUpdateで更新を検出
ReCalculate();
}
// パラメータやTextMeshProの変更を監視し,変更があればメッシュの再変形を行う
public void ReCalculate()
{
if (!_textMeshPro.havePropertiesChanged && _isActive == isActive && _flip == flip &&
_lastRadius == radius && _lastSpread == spread && _rectTransform.rect == _lastRect) return;
_isActive = isActive;
_flip = flip;
_lastRadius = radius;
_lastSpread = spread;
_lastRect = _rectTransform.rect;
if(isActive) SetCurve();
else _textMeshPro.ForceMeshUpdate();
var enu = _textMeshPro.textInfo.meshInfo.SelectMany(m => m.vertices).Select(v => v.y).ToArray();
_minY = enu.Min();
_maxY = enu.Max();
}
// Textに対しメッシュの変形を実行
private void SetCurve()
{
_textMeshPro.ForceMeshUpdate();
var textInfo = _textMeshPro.textInfo;
var characterCount = textInfo.characterCount;
var rect = _rectTransform.rect;
var minX = rect.xMin + _textMeshPro.margin.x;
var maxX = rect.xMax - _textMeshPro.margin.y;
for (var i = 0; i < characterCount; i++)
{
if (!textInfo.characterInfo[i].isVisible) continue;
var vertexIndex = textInfo.characterInfo[i].vertexIndex;
var materialIndex = textInfo.characterInfo[i].materialReferenceIndex;
var vertices = textInfo.meshInfo[materialIndex].vertices;
// 文字の中点座標を計算
Vector3 offsetToMidBaseline =
new Vector2((vertices[vertexIndex + 0].x + vertices[vertexIndex + 2].x) / 2,
textInfo.characterInfo[i].baseLine);
// 変形後の文字の中心位置を計算
var val = (offsetToMidBaseline.x - minX) / (maxX - minX);
var pos0 = CalcPositionFromCircle(val);
var pos1 = CalcPositionFromCircle(val + 0.0001f);
var rotation = Quaternion.FromToRotation(Vector3.right, (pos1 - pos0).normalized);
// 各文字の4頂点への処理
for (var j = 0; j < 4; ++j)
{
var point = vertices[vertexIndex + j];
// 中点からの相対ベクトルを算出
point -= offsetToMidBaseline;
// 移動と回転を適用
point = rotation * point + new Vector3(pos0.x, offsetToMidBaseline.y, pos0.z);
vertices[vertexIndex + j] = point;
}
}
_textMeshPro.UpdateVertexData();
}
// 0~1のvalueに対し,円周上の位置を返す
private Vector3 CalcPositionFromCircle(float val)
{
var angle = (float) (1.5 * Math.PI + (val - 0.5f) * spread * Mathf.Deg2Rad);
var x = radius * Mathf.Cos(angle);
var z = (flip ? -1 : 1) * radius * (1 + Mathf.Sin(angle));
return new Vector3(x, 0, z);
}
private readonly Color _rectColor = new Color(0.98f, 0.498f, 0.196f);
private readonly Color _circleColor = new Color(0.176f, 0.784f, 1);
private void OnDrawGizmosSelected()
{
var old = Gizmos.matrix;
var transform1 = transform;
var lossyScale = transform1.lossyScale;
Gizmos.matrix = Matrix4x4.TRS(transform1.position + (flip ? -1 : 1) * transform1.forward * radius * lossyScale.z, transform1.rotation, lossyScale);
const int step = 5;
var rangeMin = (flip ? 0 : 180) - spread / 2;
var rangeMax = (flip ? 0 : 180) + spread / 2;
var from = new Vector3(radius * Mathf.Sin(rangeMin * Mathf.Deg2Rad), 0, radius * Mathf.Cos(rangeMin * Mathf.Deg2Rad));
for (var i = rangeMin; i <= 360 + rangeMin; i += step)
{
// 境界部での丸め込み
var deg = i;
if (deg - rangeMin > 0 && deg - rangeMin <= step) deg = rangeMin;
else if (deg - rangeMax > 0 && deg - rangeMax <= step) deg = rangeMax;
var to = new Vector3(radius * Mathf.Sin(deg * Mathf.Deg2Rad), 0, radius * Mathf.Cos(deg * Mathf.Deg2Rad));
if (deg == rangeMin || deg == rangeMax)
{
Gizmos.color = _rectColor;
Gizmos.DrawLine(new Vector3(to.x, _minY, to.z), new Vector3(to.x, _maxY, to.z));
Gizmos.DrawLine(new Vector3(to.x, _minY, to.z), new Vector3(to.x, _maxY, to.z));
}
if (from != to && rangeMin <= deg && deg <= rangeMax)
{
Gizmos.color = _rectColor;
Gizmos.DrawLine(new Vector3(from.x, _minY, from.z), new Vector3(to.x, _minY, to.z));
Gizmos.DrawLine(new Vector3(from.x, _maxY, from.z), new Vector3(to.x, _maxY, to.z));
}
else
{
Gizmos.color = _circleColor;
Gizmos.DrawLine(from, to);
}
from = to;
}
Gizmos.matrix = old;
}
}
}
パラメータの説明
オブジェクトにアタッチすると,Inspectorに↓のような項目があります.
-
Is Active
は曲げるかどうか(False
の時適用されない) -
Flip
は内側と外側どちらに曲げるか(Falseの時外側向き
) -
Radius
は曲げられる円の半径 -
Spread
は広がりの角度
を表します.
Spread
パラメータなど,広がったときの文字の位置はTextMeshProのPadding
等を考慮した位置になります.
つまり,Scene
ビューで表示される黄色い枠の端から端までを範囲として,それを"Spread度"曲げた描画が得られます.
制限事項
基本的なテキストの装飾はそのまま適用できますが,下線や取り消し線は↓のように曲げることができません.
これはTextMeshProのメッシュの仕様(下線を4頂点で表現している)ことによるもので,Shaderの自作やTextMeshPro自体の修正で対処できそうではありますが,大掛かりになりすぎるので今回は未対応にしました.
実装について
実装は次のような発想によるものです.
まず,TextMeshProの文字は,それぞれが下のように4つの頂点で位置を指定しています.
その,一つの文字につき4つの頂点は,TextMeshPro
コンポーネントのtextInfo.meshInfo[materialIndex].vertices
にVector3配列として格納されています.
そのmaterialIndex
はi
番目の文字ならtextInfo.characterInfo[i].materialReferenceIndex
で取得できます.
この頂点位置を修正し,TextMeshPro
コンポーネントのUpdateVertexData()
メソッドを呼んであげることで,メッシュを動的に書き換えることができます.
各文字を円周上に配置する方法ですが,これは文字の中心座標の移動ベクトルと回転ベクトルを求め,それを適用することで行います.
↓のように,任意の場所への移動は座標の移動と回転により表すことができます.
それでこの2つのベクトルの求め方ですが,以下の様に行います(詳細はコードを見てください(投げやり))
SetCurve()
メソッドで行っている処理です.次の処理を各文字に対し適用しています.
- 黄色い矩形の左端(Sample Textで言う"S"側)を0,右端("t"側)を1とした1次元座標での中点の座標を求める
- 円周上の展開する範囲を0~1に射影し,中点の移動後の座標を求める(
CalcPositionFromCircle()
メソッドでの処理)- この移動後の座標 - 元の座標が移動ベクトル
- 中点の座標から0.0001だけ右に移動した点の円周上の座標も計算し,その差分を求める
- 差分を正規化し,Vector3.rightと一緒に
Quaternion.FromToRotation()
とかいう便利メソッドに突っ込むと回転ベクトルが得られる
- 差分を正規化し,Vector3.rightと一緒に
- この得られた2つのベクトルを4頂点に対し適用する
- コードでは4頂点の位置から一度中点座標を引き,移動と回転の適用後再び足すことで処理の簡略化を図っている
SceneビューでのGizmoの描画は別の方法で計算していますが,そっちのほうはコードを読んでください(端点座標だけわかればいいのですごく簡便な方法をとっています)
既知の問題
Text Mesh Proの実装上の仕様から,起きる問題が一部あります.
- 一部の透明オブジェクトとの間で,見る角度によってテキストが消える,オブジェクトの後ろから透けて見える
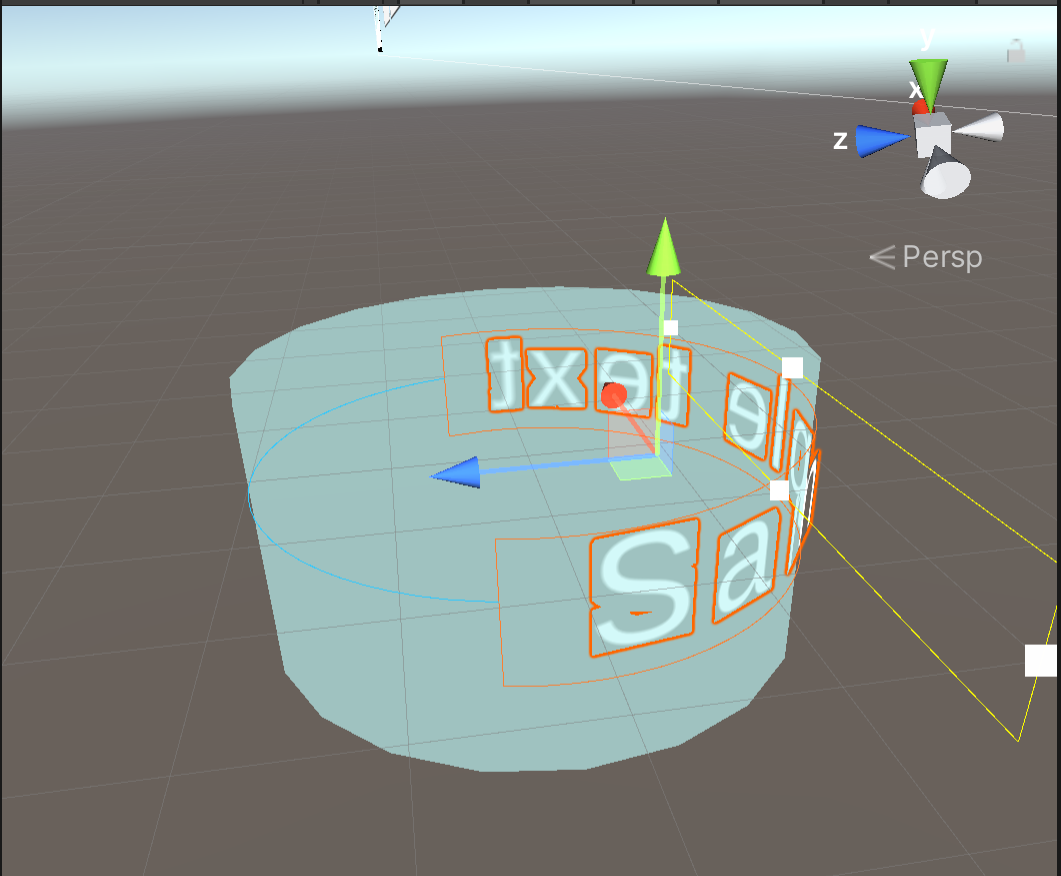
- また,逆に↓では本来オブジェクトの後ろにあるはずの文字も手前にあるように描画されてしまいます.

これを回避するためのText Mesh Pro用のシェーダを書きました.(TextMeshProの標準シェーダの描画順をTransparentCutout
しただけです)
半透明オブジェクトとの描画順をいい感じにするシェーダー
Shader "TextMeshPro/Distance Field (Cutout)" {
Properties {
_FaceTex ("Face Texture", 2D) = "white" {}
_FaceUVSpeedX ("Face UV Speed X", Range(-5, 5)) = 0.0
_FaceUVSpeedY ("Face UV Speed Y", Range(-5, 5)) = 0.0
[HDR]_FaceColor ("Face Color", Color) = (1,1,1,1)
_FaceDilate ("Face Dilate", Range(-1,1)) = 0
[HDR]_OutlineColor ("Outline Color", Color) = (0,0,0,1)
_OutlineTex ("Outline Texture", 2D) = "white" {}
_OutlineUVSpeedX ("Outline UV Speed X", Range(-5, 5)) = 0.0
_OutlineUVSpeedY ("Outline UV Speed Y", Range(-5, 5)) = 0.0
_OutlineWidth ("Outline Thickness", Range(0, 1)) = 0
_OutlineSoftness ("Outline Softness", Range(0,1)) = 0
_Bevel ("Bevel", Range(0,1)) = 0.5
_BevelOffset ("Bevel Offset", Range(-0.5,0.5)) = 0
_BevelWidth ("Bevel Width", Range(-.5,0.5)) = 0
_BevelClamp ("Bevel Clamp", Range(0,1)) = 0
_BevelRoundness ("Bevel Roundness", Range(0,1)) = 0
_LightAngle ("Light Angle", Range(0.0, 6.2831853)) = 3.1416
[HDR]_SpecularColor ("Specular", Color) = (1,1,1,1)
_SpecularPower ("Specular", Range(0,4)) = 2.0
_Reflectivity ("Reflectivity", Range(5.0,15.0)) = 10
_Diffuse ("Diffuse", Range(0,1)) = 0.5
_Ambient ("Ambient", Range(1,0)) = 0.5
_BumpMap ("Normal map", 2D) = "bump" {}
_BumpOutline ("Bump Outline", Range(0,1)) = 0
_BumpFace ("Bump Face", Range(0,1)) = 0
_ReflectFaceColor ("Reflection Color", Color) = (0,0,0,1)
_ReflectOutlineColor("Reflection Color", Color) = (0,0,0,1)
_Cube ("Reflection Cubemap", Cube) = "black" { /* TexGen CubeReflect */ }
_EnvMatrixRotation ("Texture Rotation", vector) = (0, 0, 0, 0)
[HDR]_UnderlayColor ("Border Color", Color) = (0,0,0, 0.5)
_UnderlayOffsetX ("Border OffsetX", Range(-1,1)) = 0
_UnderlayOffsetY ("Border OffsetY", Range(-1,1)) = 0
_UnderlayDilate ("Border Dilate", Range(-1,1)) = 0
_UnderlaySoftness ("Border Softness", Range(0,1)) = 0
[HDR]_GlowColor ("Color", Color) = (0, 1, 0, 0.5)
_GlowOffset ("Offset", Range(-1,1)) = 0
_GlowInner ("Inner", Range(0,1)) = 0.05
_GlowOuter ("Outer", Range(0,1)) = 0.05
_GlowPower ("Falloff", Range(1, 0)) = 0.75
_WeightNormal ("Weight Normal", float) = 0
_WeightBold ("Weight Bold", float) = 0.5
_ShaderFlags ("Flags", float) = 0
_ScaleRatioA ("Scale RatioA", float) = 1
_ScaleRatioB ("Scale RatioB", float) = 1
_ScaleRatioC ("Scale RatioC", float) = 1
_MainTex ("Font Atlas", 2D) = "white" {}
_TextureWidth ("Texture Width", float) = 512
_TextureHeight ("Texture Height", float) = 512
_GradientScale ("Gradient Scale", float) = 5.0
_ScaleX ("Scale X", float) = 1.0
_ScaleY ("Scale Y", float) = 1.0
_PerspectiveFilter ("Perspective Correction", Range(0, 1)) = 0.875
_Sharpness ("Sharpness", Range(-1,1)) = 0
_VertexOffsetX ("Vertex OffsetX", float) = 0
_VertexOffsetY ("Vertex OffsetY", float) = 0
_MaskCoord ("Mask Coordinates", vector) = (0, 0, 32767, 32767)
_ClipRect ("Clip Rect", vector) = (-32767, -32767, 32767, 32767)
_MaskSoftnessX ("Mask SoftnessX", float) = 0
_MaskSoftnessY ("Mask SoftnessY", float) = 0
_StencilComp ("Stencil Comparison", Float) = 8
_Stencil ("Stencil ID", Float) = 0
_StencilOp ("Stencil Operation", Float) = 0
_StencilWriteMask ("Stencil Write Mask", Float) = 255
_StencilReadMask ("Stencil Read Mask", Float) = 255
_CullMode ("Cull Mode", Float) = 0
_ColorMask ("Color Mask", Float) = 15
}
SubShader {
Tags
{
"Queue"="AlphaTest"
"IgnoreProjector"="True"
"RenderType"="TransparentCutout"
}
Stencil
{
Ref [_Stencil]
Comp [_StencilComp]
Pass [_StencilOp]
ReadMask [_StencilReadMask]
WriteMask [_StencilWriteMask]
}
Cull [_CullMode]
ZWrite On
Lighting Off
Fog { Mode Off }
ZTest [unity_GUIZTestMode]
Blend One OneMinusSrcAlpha
ColorMask [_ColorMask]
Pass {
CGPROGRAM
#pragma target 3.0
#pragma vertex VertShader
#pragma fragment PixShader
#pragma shader_feature __ BEVEL_ON
#pragma shader_feature __ UNDERLAY_ON UNDERLAY_INNER
#pragma shader_feature __ GLOW_ON
#pragma multi_compile __ UNITY_UI_CLIP_RECT
#pragma multi_compile __ UNITY_UI_ALPHACLIP
#include "UnityCG.cginc"
#include "UnityUI.cginc"
// UI Editable properties
uniform sampler2D _FaceTex; // Alpha : Signed Distance
uniform float _FaceUVSpeedX;
uniform float _FaceUVSpeedY;
uniform fixed4 _FaceColor; // RGBA : Color + Opacity
uniform float _FaceDilate; // v[ 0, 1]
uniform float _OutlineSoftness; // v[ 0, 1]
uniform sampler2D _OutlineTex; // RGBA : Color + Opacity
uniform float _OutlineUVSpeedX;
uniform float _OutlineUVSpeedY;
uniform fixed4 _OutlineColor; // RGBA : Color + Opacity
uniform float _OutlineWidth; // v[ 0, 1]
uniform float _Bevel; // v[ 0, 1]
uniform float _BevelOffset; // v[-1, 1]
uniform float _BevelWidth; // v[-1, 1]
uniform float _BevelClamp; // v[ 0, 1]
uniform float _BevelRoundness; // v[ 0, 1]
uniform sampler2D _BumpMap; // Normal map
uniform float _BumpOutline; // v[ 0, 1]
uniform float _BumpFace; // v[ 0, 1]
uniform samplerCUBE _Cube; // Cube / sphere map
uniform fixed4 _ReflectFaceColor; // RGB intensity
uniform fixed4 _ReflectOutlineColor;
//uniform float _EnvTiltX; // v[-1, 1]
//uniform float _EnvTiltY; // v[-1, 1]
uniform float3 _EnvMatrixRotation;
uniform float4x4 _EnvMatrix;
uniform fixed4 _SpecularColor; // RGB intensity
uniform float _LightAngle; // v[ 0,Tau]
uniform float _SpecularPower; // v[ 0, 1]
uniform float _Reflectivity; // v[ 5, 15]
uniform float _Diffuse; // v[ 0, 1]
uniform float _Ambient; // v[ 0, 1]
uniform fixed4 _UnderlayColor; // RGBA : Color + Opacity
uniform float _UnderlayOffsetX; // v[-1, 1]
uniform float _UnderlayOffsetY; // v[-1, 1]
uniform float _UnderlayDilate; // v[-1, 1]
uniform float _UnderlaySoftness; // v[ 0, 1]
uniform fixed4 _GlowColor; // RGBA : Color + Intesity
uniform float _GlowOffset; // v[-1, 1]
uniform float _GlowOuter; // v[ 0, 1]
uniform float _GlowInner; // v[ 0, 1]
uniform float _GlowPower; // v[ 1, 1/(1+4*4)]
// API Editable properties
uniform float _ShaderFlags;
uniform float _WeightNormal;
uniform float _WeightBold;
uniform float _ScaleRatioA;
uniform float _ScaleRatioB;
uniform float _ScaleRatioC;
uniform float _VertexOffsetX;
uniform float _VertexOffsetY;
//uniform float _UseClipRect;
uniform float _MaskID;
uniform sampler2D _MaskTex;
uniform float4 _MaskCoord;
uniform float4 _ClipRect; // bottom left(x,y) : top right(z,w)
//uniform float _MaskWipeControl;
//uniform float _MaskEdgeSoftness;
//uniform fixed4 _MaskEdgeColor;
//uniform bool _MaskInverse;
uniform float _MaskSoftnessX;
uniform float _MaskSoftnessY;
// Font Atlas properties
uniform sampler2D _MainTex;
uniform float _TextureWidth;
uniform float _TextureHeight;
uniform float _GradientScale;
uniform float _ScaleX;
uniform float _ScaleY;
uniform float _PerspectiveFilter;
uniform float _Sharpness;
float2 UnpackUV(float uv)
{
float2 output;
output.x = floor(uv / 4096);
output.y = uv - 4096 * output.x;
return output * 0.001953125;
}
fixed4 GetColor(half d, fixed4 faceColor, fixed4 outlineColor, half outline, half softness)
{
half faceAlpha = 1-saturate((d - outline * 0.5 + softness * 0.5) / (1.0 + softness));
half outlineAlpha = saturate((d + outline * 0.5)) * sqrt(min(1.0, outline));
faceColor.rgb *= faceColor.a;
outlineColor.rgb *= outlineColor.a;
faceColor = lerp(faceColor, outlineColor, outlineAlpha);
faceColor *= faceAlpha;
return faceColor;
}
float3 GetSurfaceNormal(float4 h, float bias)
{
bool raisedBevel = step(1, fmod(_ShaderFlags, 2));
h += bias+_BevelOffset;
float bevelWidth = max(.01, _OutlineWidth+_BevelWidth);
// Track outline
h -= .5;
h /= bevelWidth;
h = saturate(h+.5);
if(raisedBevel) h = 1 - abs(h*2.0 - 1.0);
h = lerp(h, sin(h*3.141592/2.0), _BevelRoundness);
h = min(h, 1.0-_BevelClamp);
h *= _Bevel * bevelWidth * _GradientScale * -2.0;
float3 va = normalize(float3(1.0, 0.0, h.y - h.x));
float3 vb = normalize(float3(0.0, -1.0, h.w - h.z));
return cross(va, vb);
}
float3 GetSurfaceNormal(float2 uv, float bias, float3 delta)
{
// Read "height field"
float4 h = {tex2D(_MainTex, uv - delta.xz).a,
tex2D(_MainTex, uv + delta.xz).a,
tex2D(_MainTex, uv - delta.zy).a,
tex2D(_MainTex, uv + delta.zy).a};
return GetSurfaceNormal(h, bias);
}
float3 GetSpecular(float3 n, float3 l)
{
float spec = pow(max(0.0, dot(n, l)), _Reflectivity);
return _SpecularColor.rgb * spec * _SpecularPower;
}
float4 GetGlowColor(float d, float scale)
{
float glow = d - (_GlowOffset*_ScaleRatioB) * 0.5 * scale;
float t = lerp(_GlowInner, (_GlowOuter * _ScaleRatioB), step(0.0, glow)) * 0.5 * scale;
glow = saturate(abs(glow/(1.0 + t)));
glow = 1.0-pow(glow, _GlowPower);
glow *= sqrt(min(1.0, t)); // Fade off glow thinner than 1 screen pixel
return float4(_GlowColor.rgb, saturate(_GlowColor.a * glow * 2));
}
float4 BlendARGB(float4 overlying, float4 underlying)
{
overlying.rgb *= overlying.a;
underlying.rgb *= underlying.a;
float3 blended = overlying.rgb + ((1-overlying.a)*underlying.rgb);
float alpha = underlying.a + (1-underlying.a)*overlying.a;
return float4(blended, alpha);
}
struct vertex_t {
UNITY_VERTEX_INPUT_INSTANCE_ID
float4 position : POSITION;
float3 normal : NORMAL;
fixed4 color : COLOR;
float2 texcoord0 : TEXCOORD0;
float2 texcoord1 : TEXCOORD1;
};
struct pixel_t {
UNITY_VERTEX_INPUT_INSTANCE_ID
UNITY_VERTEX_OUTPUT_STEREO
float4 position : SV_POSITION;
fixed4 color : COLOR;
float2 atlas : TEXCOORD0; // Atlas
float4 param : TEXCOORD1; // alphaClip, scale, bias, weight
float4 mask : TEXCOORD2; // Position in object space(xy), pixel Size(zw)
float3 viewDir : TEXCOORD3;
#if (UNDERLAY_ON || UNDERLAY_INNER)
float4 texcoord2 : TEXCOORD4; // u,v, scale, bias
fixed4 underlayColor : COLOR1;
#endif
float4 textures : TEXCOORD5;
};
// Used by Unity internally to handle Texture Tiling and Offset.
float4 _FaceTex_ST;
float4 _OutlineTex_ST;
pixel_t VertShader(vertex_t input)
{
pixel_t output;
UNITY_INITIALIZE_OUTPUT(pixel_t, output);
UNITY_SETUP_INSTANCE_ID(input);
UNITY_TRANSFER_INSTANCE_ID(input,output);
UNITY_INITIALIZE_VERTEX_OUTPUT_STEREO(output);
float bold = step(input.texcoord1.y, 0);
float4 vert = input.position;
vert.x += _VertexOffsetX;
vert.y += _VertexOffsetY;
float4 vPosition = UnityObjectToClipPos(vert);
float2 pixelSize = vPosition.w;
pixelSize /= float2(_ScaleX, _ScaleY) * abs(mul((float2x2)UNITY_MATRIX_P, _ScreenParams.xy));
float scale = rsqrt(dot(pixelSize, pixelSize));
scale *= abs(input.texcoord1.y) * _GradientScale * (_Sharpness + 1);
if (UNITY_MATRIX_P[3][3] == 0) scale = lerp(abs(scale) * (1 - _PerspectiveFilter), scale, abs(dot(UnityObjectToWorldNormal(input.normal.xyz), normalize(WorldSpaceViewDir(vert)))));
float weight = lerp(_WeightNormal, _WeightBold, bold) / 4.0;
weight = (weight + _FaceDilate) * _ScaleRatioA * 0.5;
float bias =(.5 - weight) + (.5 / scale);
float alphaClip = (1.0 - _OutlineWidth * _ScaleRatioA - _OutlineSoftness * _ScaleRatioA);
#if GLOW_ON
alphaClip = min(alphaClip, 1.0 - _GlowOffset * _ScaleRatioB - _GlowOuter * _ScaleRatioB);
#endif
alphaClip = alphaClip / 2.0 - ( .5 / scale) - weight;
#if (UNDERLAY_ON || UNDERLAY_INNER)
float4 underlayColor = _UnderlayColor;
underlayColor.rgb *= underlayColor.a;
float bScale = scale;
bScale /= 1 + ((_UnderlaySoftness*_ScaleRatioC) * bScale);
float bBias = (0.5 - weight) * bScale - 0.5 - ((_UnderlayDilate * _ScaleRatioC) * 0.5 * bScale);
float x = -(_UnderlayOffsetX * _ScaleRatioC) * _GradientScale / _TextureWidth;
float y = -(_UnderlayOffsetY * _ScaleRatioC) * _GradientScale / _TextureHeight;
float2 bOffset = float2(x, y);
#endif
// Generate UV for the Masking Texture
float4 clampedRect = clamp(_ClipRect, -2e10, 2e10);
float2 maskUV = (vert.xy - clampedRect.xy) / (clampedRect.zw - clampedRect.xy);
// Support for texture tiling and offset
float2 textureUV = UnpackUV(input.texcoord1.x);
float2 faceUV = TRANSFORM_TEX(textureUV, _FaceTex);
float2 outlineUV = TRANSFORM_TEX(textureUV, _OutlineTex);
output.position = vPosition;
output.color = input.color;
output.atlas = input.texcoord0;
output.param = float4(alphaClip, scale, bias, weight);
output.mask = half4(vert.xy * 2 - clampedRect.xy - clampedRect.zw, 0.25 / (0.25 * half2(_MaskSoftnessX, _MaskSoftnessY) + pixelSize.xy));
output.viewDir = mul((float3x3)_EnvMatrix, _WorldSpaceCameraPos.xyz - mul(unity_ObjectToWorld, vert).xyz);
#if (UNDERLAY_ON || UNDERLAY_INNER)
output.texcoord2 = float4(input.texcoord0 + bOffset, bScale, bBias);
output.underlayColor = underlayColor;
#endif
output.textures = float4(faceUV, outlineUV);
return output;
}
fixed4 PixShader(pixel_t input) : SV_Target
{
UNITY_SETUP_INSTANCE_ID(input);
float c = tex2D(_MainTex, input.atlas).a;
#ifndef UNDERLAY_ON
clip(c - input.param.x);
#endif
float scale = input.param.y;
float bias = input.param.z;
float weight = input.param.w;
float sd = (bias - c) * scale;
float outline = (_OutlineWidth * _ScaleRatioA) * scale;
float softness = (_OutlineSoftness * _ScaleRatioA) * scale;
half4 faceColor = _FaceColor;
half4 outlineColor = _OutlineColor;
faceColor.rgb *= input.color.rgb;
faceColor *= tex2D(_FaceTex, input.textures.xy + float2(_FaceUVSpeedX, _FaceUVSpeedY) * _Time.y);
outlineColor *= tex2D(_OutlineTex, input.textures.zw + float2(_OutlineUVSpeedX, _OutlineUVSpeedY) * _Time.y);
faceColor = GetColor(sd, faceColor, outlineColor, outline, softness);
#if BEVEL_ON
float3 dxy = float3(0.5 / _TextureWidth, 0.5 / _TextureHeight, 0);
float3 n = GetSurfaceNormal(input.atlas, weight, dxy);
float3 bump = UnpackNormal(tex2D(_BumpMap, input.textures.xy + float2(_FaceUVSpeedX, _FaceUVSpeedY) * _Time.y)).xyz;
bump *= lerp(_BumpFace, _BumpOutline, saturate(sd + outline * 0.5));
n = normalize(n- bump);
float3 light = normalize(float3(sin(_LightAngle), cos(_LightAngle), -1.0));
float3 col = GetSpecular(n, light);
faceColor.rgb += col*faceColor.a;
faceColor.rgb *= 1-(dot(n, light)*_Diffuse);
faceColor.rgb *= lerp(_Ambient, 1, n.z*n.z);
fixed4 reflcol = texCUBE(_Cube, reflect(input.viewDir, -n));
faceColor.rgb += reflcol.rgb * lerp(_ReflectFaceColor.rgb, _ReflectOutlineColor.rgb, saturate(sd + outline * 0.5)) * faceColor.a;
#endif
#if UNDERLAY_ON
float d = tex2D(_MainTex, input.texcoord2.xy).a * input.texcoord2.z;
faceColor += input.underlayColor * saturate(d - input.texcoord2.w) * (1 - faceColor.a);
#endif
#if UNDERLAY_INNER
float d = tex2D(_MainTex, input.texcoord2.xy).a * input.texcoord2.z;
faceColor += input.underlayColor * (1 - saturate(d - input.texcoord2.w)) * saturate(1 - sd) * (1 - faceColor.a);
#endif
#if GLOW_ON
float4 glowColor = GetGlowColor(sd, scale);
faceColor.rgb += glowColor.rgb * glowColor.a;
#endif
// Alternative implementation to UnityGet2DClipping with support for softness.
#if UNITY_UI_CLIP_RECT
half2 m = saturate((_ClipRect.zw - _ClipRect.xy - abs(input.mask.xy)) * input.mask.zw);
faceColor *= m.x * m.y;
#endif
#if UNITY_UI_ALPHACLIP
clip(faceColor.a - 0.001);
#endif
return faceColor * input.color.a;
}
ENDCG
}
}
Fallback "TextMeshPro/Mobile/Distance Field"
CustomEditor "TMPro.EditorUtilities.TMP_SDFShaderGUI"
}
↑のファイルを保存し,Assets
以下の適当な場所に配置し,マテリアルの選択からTextMeshPro/Distance Field (Cutout)
を選択します.

これで↓のように正しい描画が得られます.
