Playgroundによるコーディングです。
初めての方は下記をご参考ください。
第1回:【初心者向け】Swift3で爆速コーディングその1(画面作成とSnippetsの使い方)
第2回:【初心者向け】Swift3で爆速コーディングその2(UIViewと文字表示)
第3回:【初心者向け】Swift3で爆速コーディングその3(ボタンクリックとイベント)
第4回:【初心者向け】Swift3で爆速コーディングその4(画像とアニメーション)
ソースコード
今回の全ソースコードです。
import UIKit
import AVFoundation
class ViewController: UIViewController,AVAudioPlayerDelegate {
var audioPlayer : AVAudioPlayer!
var button : UIButton!
override func viewDidLoad() {
super.viewDidLoad()
// 再生する音源のURLを生成
let soundFilePath : String = Bundle.main.path(forResource: "sound", ofType: "mp3")!
let fileURL : URL = URL(fileURLWithPath: soundFilePath)
do{
// AVAudioPlayerのインスタンス化
audioPlayer = try AVAudioPlayer(contentsOf: fileURL)
// AVAudioPlayerのデリゲートをセット
audioPlayer.delegate = self
}
catch{
}
//ボタンの生成
button = UIButton()
button.frame = CGRect(x:0,y:0,width:100, height:30)
button.setTitle("▶︎", for: UIControlState.normal)
button.setTitleColor(UIColor.black, for: .normal)
button.backgroundColor = UIColor.green
button.layer.masksToBounds = true
button.layer.cornerRadius = 10.0
self.view.addSubview(button)
button.addTarget(self, action: #selector(self.onClick(_:)), for: UIControlEvents.touchUpInside)
}
// ボタンがタップされた時に呼ばれるメソッド
func onClick(_ sender: UIButton) {
// playingプロパティがtrueであれば音源再生中
if audioPlayer.isPlaying == true {
// audioPlayerを一時停止
audioPlayer.pause()
sender.setTitle("▶︎", for: .normal)
} else {
// audioPlayerの再生
audioPlayer.play()
sender.setTitle("■", for: .normal)
}
}
// 音楽再生が成功した時に呼ばれるメソッド
func audioPlayerDidFinishPlaying(_ player: AVAudioPlayer, successfully flag: Bool) {
print("Music Finish")
// 再度myButtonを"▶︎"に設定
button.setTitle("▶︎", for: .normal)
}
// デコード中にエラーが起きた時に呼ばれるメソッド
func audioPlayerDecodeErrorDidOccur(_ player: AVAudioPlayer, error: Error?) {
print("Error")
}
override func viewWillAppear(_ animated: Bool) {
super.viewWillAppear(animated)
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
}
}
let viewController = ViewController()
viewController.view.backgroundColor = UIColor.white
import PlaygroundSupport
PlaygroundPage.current.liveView = viewController
PlaygroundPage.current.needsIndefiniteExecution = true
プレビュー
再生ボタンを押したら、サウンドを再生します。
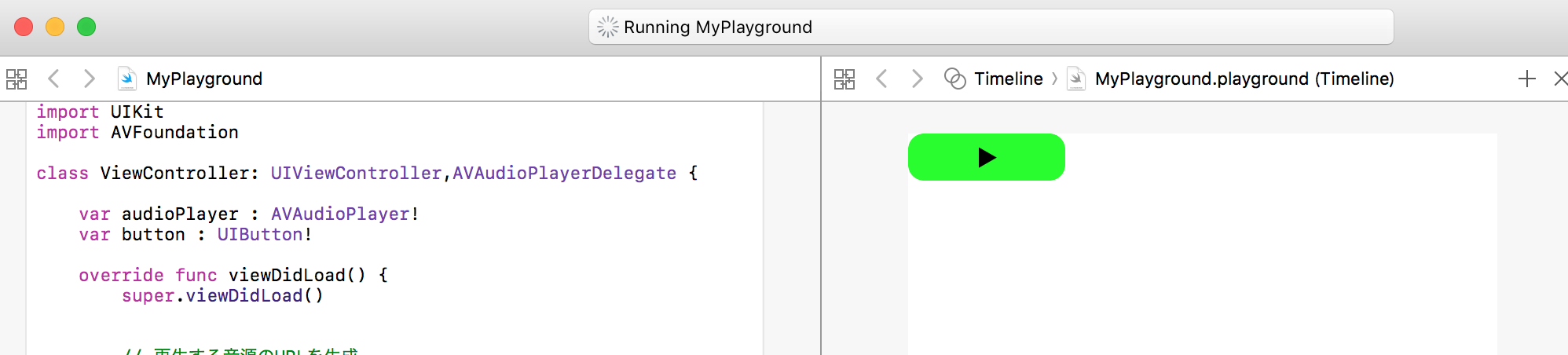
デリゲート
delegateは処理を移譲する機能です。
概念的にはJavaとかのインタフェースに近いです。
delegateを継承したクラスはdelegateメソッドを実装する必要があります。(optionalのメソッドは実装任意です)
今回はAVAudioPlayerクラスのイベント処理をするためAVAudioPlayerDelegateを使います。
class ViewController: UIViewController,AVAudioPlayerDelegate {
ViewDidLoadでデリゲートをセットします。
// AVAudioPlayerのデリゲートをセット
audioPlayer.delegate = self
今回はaudioPlayerDidFinishPlayingメソッドとaudioPlayerDecodeErrorDidOccurメソッドを実装します。
// 音楽再生が成功した時に呼ばれるメソッド
func audioPlayerDidFinishPlaying(_ player: AVAudioPlayer, successfully flag: Bool)
// デコード中にエラーが起きた時に呼ばれるメソッド
func audioPlayerDecodeErrorDidOccur(_ player: AVAudioPlayer, error: Error?)
サウンド
サウンド周りのライブラリを利用するにはAVFoundationフレームワークをインポートします。
import AVFoundation
サウンドファイル(sound.mp3)を追加します。
適当なサウンドファイルを用意してください
追加の仕方は画像と同じなので第4回を参考にしてください
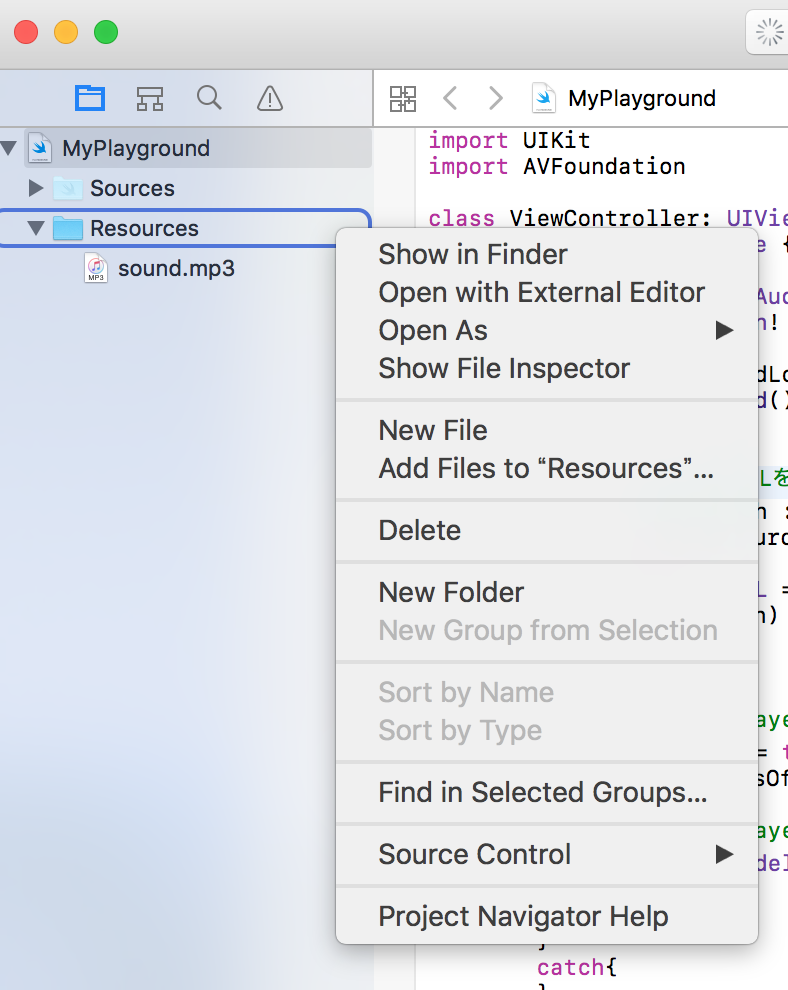
サウンドファイルをロードします。
// 再生する音源のURLを生成
let soundFilePath : String = Bundle.main.path(forResource: "sound", ofType: "mp3")!
let fileURL : URL = URL(fileURLWithPath: soundFilePath)
do{
// AVAudioPlayerのインスタンス化
audioPlayer = try AVAudioPlayer(contentsOf: fileURL)
// AVAudioPlayerのデリゲートをセット
audioPlayer.delegate = self
}
catch{
}
ボタンが押されたらonClickメソッドが呼ばれ、
サウンドを再生します。再生中なら一時停止になります。
ボタンとコントロールのイベント処理に関しては第3回を参考にしてください
// playingプロパティがtrueであれば音源再生中
if audioPlayer.isPlaying == true {
// audioPlayerを一時停止
audioPlayer.pause()
sender.setTitle("▶︎", for: .normal)
} else {
// audioPlayerの再生
audioPlayer.play()
sender.setTitle("■", for: .normal)
}
再生が終わるとデリゲートのメソッドがコールバックされます。
デリゲートのメソッドはこのようにコールバックメソッドがほとんどです。
// 音楽再生が成功した時に呼ばれるメソッド
func audioPlayerDidFinishPlaying(_ player: AVAudioPlayer, successfully flag: Bool)