Swift3.0を使って簡単なiPhoneアプリを作ってみます
初心者向け)Swift3.0で簡単なiPhoneアプリを作ってみるで作ったシンプルなSwift3.0アプリでビーコンを検出してみます
1. ビーコンを用意します
- ビーコンを用意し、UUID、major、minor値を確認します
- 手近にビーコンがない場合、iPhoneやMacbookをビーコンにすることができます
- Macbookからビーコンを発信する方法→SwiftとiBeaconを使ってお母さんが自分の部屋に近づいて来た事を警告するアプリをつくる
- iPhoneからビーコンを発信する方法→手軽にビーコンの動作を体験できる「Beacon入門」アプリが登場!
2. アプリを用意します
-
ビーコンを受信するアプリを作成します
-
XcodeでSwiftアプリを用意します
-
ViewController.swiftに下記のコードをコピペします
スタンプラリーサンプルアプリ
//
// ViewController.swift
// beaconappc
//
// Created by Tohru Suzuki on 2017/06/22.
// Copyright © 2017年 Tohru Suzuki. All rights reserved.
//
import UIKit
import CoreLocation
class ViewController: UIViewController, CLLocationManagerDelegate {
var myLocationManager:CLLocationManager!
var myBeaconRegion:CLBeaconRegion!
var beaconUuids: NSMutableArray!
var beaconDetails: NSMutableArray!
let label_state = UILabel()
let button_found = UIButton()
let label_score = UILabel()
let button_reset = UIButton()
let UUIDList = [
"AAAAAAAA-AAAA-AAAA-AAAA-AAAAAAAAAAAA",
"BBBBBBBB-BBBB-BBBB-BBBB-BBBBBBBBBBBB"
]
override func viewDidLoad() {
super.viewDidLoad()
myLocationManager = CLLocationManager()
myLocationManager.delegate = self
myLocationManager.desiredAccuracy = kCLLocationAccuracyBest
myLocationManager.distanceFilter = 1
let status = CLLocationManager.authorizationStatus()
print("CLAuthorizedStatus: \(status.rawValue)");
if(status == .notDetermined) {
myLocationManager.requestAlwaysAuthorization()
}
beaconUuids = NSMutableArray()
beaconDetails = NSMutableArray()
self.view.backgroundColor = UIColor.init(red:0.8, green: 0.84, blue: 1, alpha: 1)
let screenWidth:CGFloat = self.view.frame.width
let screenHeight:CGFloat = self.view.frame.height
label_state.frame = CGRect(x:screenWidth/12, y:120, width:screenWidth*11/12, height:50);
label_state.text = ""
label_state.textAlignment = NSTextAlignment.center
label_state.font = UIFont.systemFont(ofSize: 30)
self.view.addSubview(label_state)
button_found.frame = CGRect(x:screenWidth/4, y:200, width:screenWidth/2, height:50)
//button_found.frame = CGRect(x:screenWidth/4, y:screenHeight/2, width:screenWidth/2, height:70)
button_found.setTitle("発見!", for:UIControlState.normal)
button_found.titleLabel?.font = UIFont.systemFont(ofSize: 30)
button_found.backgroundColor = UIColor.init(red:0.9, green: 0.9, blue: 0.9, alpha: 1)
button_found.addTarget(self, action: #selector(ViewController.buttonTapped(sender:)), for: .touchUpInside)
self.view.addSubview(button_found)
label_score.frame = CGRect(x:screenWidth/12, y:280, width:screenWidth*11/12, height:50);
label_score.text = ""
label_score.textAlignment = NSTextAlignment.center
label_score.font = UIFont.systemFont(ofSize: 30)
self.view.addSubview(label_score)
button_reset.frame = CGRect(x:screenWidth/4, y:360, width:screenWidth/2, height:50)
//button_reset.frame = CGRect(x:screenWidth/4, y:screenHeight/2, width:screenWidth/2, height:50)
button_reset.setTitle("リセット", for:UIControlState.normal)
button_reset.titleLabel?.font = UIFont.systemFont(ofSize: 30)
button_reset.backgroundColor = UIColor.init(red:0.9, green: 0.9, blue: 0.9, alpha: 1)
button_reset.addTarget(self, action: #selector(ViewController.buttonTappedReset(sender:)), for: .touchUpInside)
self.view.addSubview(button_reset)
}
internal func buttonTapped(sender : AnyObject) {
if (label_state.text == "すぐそばです!!") {
label_score.text = "ポイントげっと!"
}
}
internal func buttonTappedReset(sender : AnyObject) {
label_score.text = ""
}
private func startMyMonitoring() {
for i in 0 ..< UUIDList.count {
let uuid: NSUUID! = NSUUID(uuidString: "\(UUIDList[i].lowercased())")
let identifierStr: String = "abcde\(i)"
myBeaconRegion = CLBeaconRegion(proximityUUID: uuid as UUID, identifier: identifierStr)
myBeaconRegion.notifyEntryStateOnDisplay = false
myBeaconRegion.notifyOnEntry = true
myBeaconRegion.notifyOnExit = true
myLocationManager.startMonitoring(for: myBeaconRegion)
}
}
func locationManager(_ manager: CLLocationManager, didChangeAuthorization status: CLAuthorizationStatus) {
print("didChangeAuthorizationStatus");
switch (status) {
case .notDetermined:
print("not determined")
break
case .restricted:
print("restricted")
break
case .denied:
print("denied")
break
case .authorizedAlways:
print("authorizedAlways")
startMyMonitoring()
break
case .authorizedWhenInUse:
print("authorizedWhenInUse")
startMyMonitoring()
break
}
}
func locationManager(_ manager: CLLocationManager, didStartMonitoringFor region: CLRegion) {
manager.requestState(for: region);
}
func locationManager(_ manager: CLLocationManager, didDetermineState state: CLRegionState, for region: CLRegion) {
switch (state) {
case .inside:
print("iBeacon inside");
manager.startRangingBeacons(in: region as! CLBeaconRegion)
break;
case .outside:
print("iBeacon outside")
break;
case .unknown:
print("iBeacon unknown")
break;
}
}
func locationManager(_ manager: CLLocationManager, didRangeBeacons beacons: [CLBeacon], in region: CLBeaconRegion) {
beaconUuids = NSMutableArray()
beaconDetails = NSMutableArray()
if(beacons.count > 0){
for i in 0 ..< beacons.count {
let beacon = beacons[i]
let beaconUUID = beacon.proximityUUID;
let minorID = beacon.minor;
let majorID = beacon.major;
let rssi = beacon.rssi;
var proximity = ""
switch (beacon.proximity) {
case CLProximity.unknown :
print("Proximity: Unknown");
proximity = "不明ですー"
break
case CLProximity.far:
print("Proximity: Far");
proximity = "遠いです。。"
break
case CLProximity.near:
print("Proximity: Near");
proximity = "近いです!"
break
case CLProximity.immediate:
print("Proximity: Immediate");
proximity = "すぐそばです!!"
break
}
beaconUuids.add(beaconUUID.uuidString)
var myBeaconDetails = "Major: \(majorID) "
myBeaconDetails += "Minor: \(minorID) "
myBeaconDetails += "Proximity:\(proximity) "
myBeaconDetails += "RSSI:\(rssi)"
print(myBeaconDetails)
beaconDetails.add(myBeaconDetails)
label_state.text = proximity
}
}
}
func locationManager(_ manager: CLLocationManager, didEnterRegion region: CLRegion) {
print("didEnterRegion: iBeacon found");
manager.startRangingBeacons(in: region as! CLBeaconRegion)
}
func locationManager(_ manager: CLLocationManager, didExitRegion region: CLRegion) {
print("didExitRegion: iBeacon lost");
manager.stopRangingBeacons(in: region as! CLBeaconRegion)
}
}
- 使用するビーコンのUUID/Major/Minorを上記のViewController.swiftのUUIDリストと置き換えます
- 上記のViewController.swiftのlabel1、label2、button1、button2を、用意したラベル(x2)、ボタン(x2)と置き換えて接続し直します
- 位置情報を使用可能にするため、ナビゲーターで「info.plist」を選択し、「Privacy - Location Always Usage Description」を追加します
4. iPhoneで確認します
- iPhoneにデプロイし、稼働を確認します
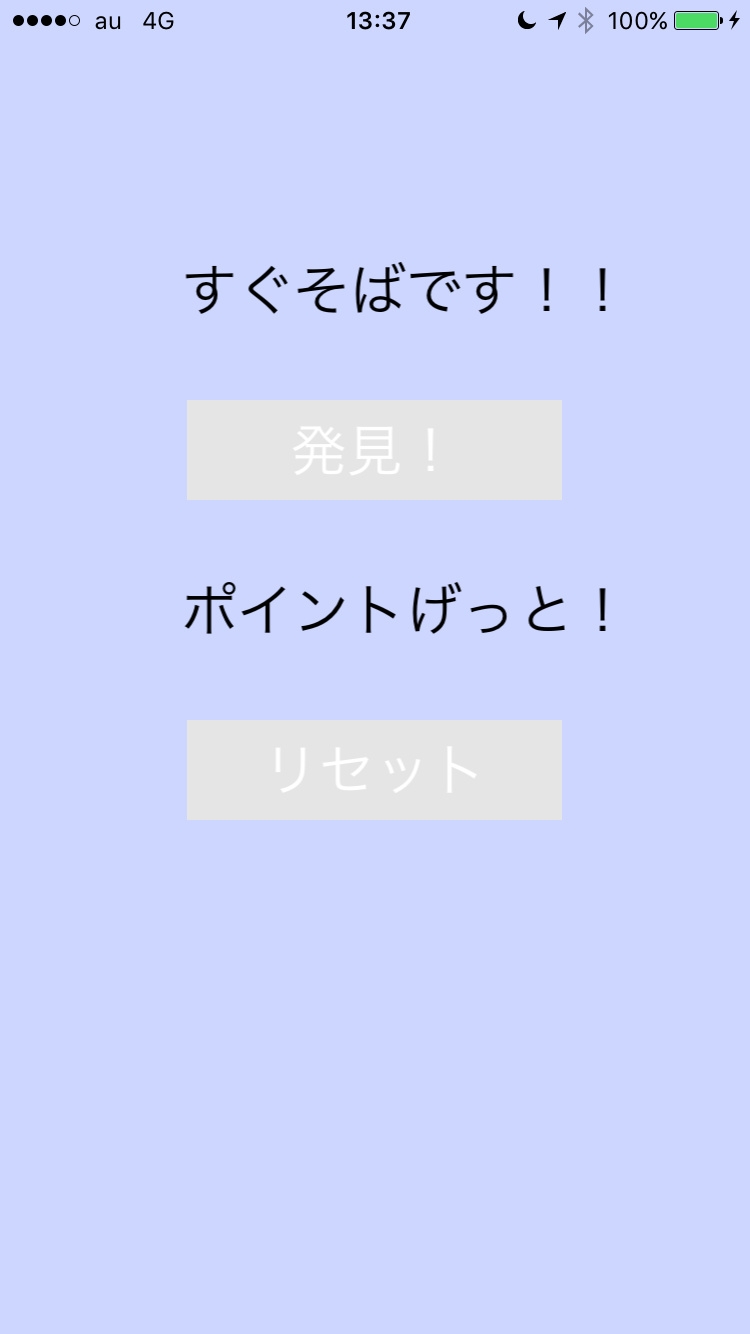