ファイルをドラッグ&ドロップでアップロード可能にするDropzoneライブラリを
をASP.NET MVCに適用する
http://www.dropzonejs.com/
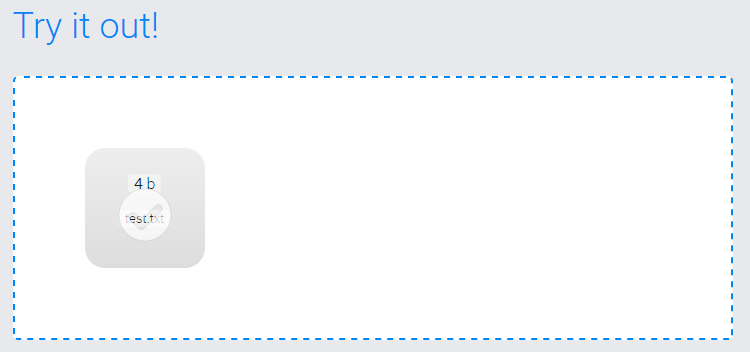
Visual Studio Nugetパッケージの管理画面から
DropzoneJSで検索をかけ、プロジェクトへインストールする
Viewにファイル送信領域を作成
<link href="~/Scripts/dropzone/dropzone.css" rel="stylesheet" />
<script src="~/Scripts/dropzone/dropzone.js"></script>
<div class="jumbotron">
<form action="~/Home/SaveUploadedFile" method="post" enctype="multipart/form-data" class="dropzone" id="dropzoneForm">
<div class="fallback">
<input name="file" type="file" multiple />
<input type="submit" value="Upload" />
</div>
</form>
</div>
Dropzoneの初期設定を行う
$(document).ready(function () {
Dropzone.options.dropzoneForm = {
init: function () {
this.on("complete", function (data) {
var res = JSON.parse(data.xhr.responseText);
});
}
};
});
#サーバー側:ファイルを受け取る処理の作成
##コントローラーの作成
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using System.IO;
namespace Dropzone.Controllers
{
public class FileUploadController : Controller
{
/// <summary>
///
/// </summary>
/// <returns></returns>
public ActionResult SaveUploadedFile()
{
bool isSavedSuccessfully = true;
string fName = "";
foreach (string fileName in Request.Files)
{
HttpPostedFileBase file = Request.Files[fileName];
//Save file content goes here
fName = file.FileName;
if (file != null && file.ContentLength > 0)
{
var originalDirectory = new DirectoryInfo(string.Format("{0}Images\\WallImages", Server.MapPath(@"\")));
string pathString = System.IO.Path.Combine(originalDirectory.ToString(), "imagepath");
var fileName1 = Path.GetFileName(file.FileName);
bool isExists = System.IO.Directory.Exists(pathString);
if (!isExists)
System.IO.Directory.CreateDirectory(pathString);
var path = string.Format("{0}\\{1}", pathString, file.FileName);
file.SaveAs(path);
}
}
if (isSavedSuccessfully)
{
return Json(new { Message = fName });
}
else
{
return Json(new { Message = "Error in saving file" });
}
}
}
}
##転送容量の設定を行う
Web.config
<configuration>
<system.web>
<httpRuntime targetFramework="4.6.1" maxRequestLength="65535" /> <!--転送容量-->
<system.webServer>
<security>
<requestFiltering>
<requestLimits maxAllowedContentLength="5242880" />
</requestFiltering>
</security>
</system.webServer>
</configuration>
##できあがり
###参考サイト
https://github.com/venkatbaggu/dropzonefileupload
http://venkatbaggu.com/file-upload-in-asp-net-mvc-using-dropzone-js-and-html5/