gitはソースのバージョン管理をするツール。 vss,tfs,svnみたいなやつ。
ローカルリポジトリとリモートリポジトリが存在し、ローカルとリモート間でpushやpull requestでコードをmergeしあう特性がある。
GUIで使いたい場合はsourcetreeを使う。コードの全量がギガを超えるとかなり重くなる。
https://ja.atlassian.com/software/sourcetree
#インストール
##debian,ubuntuの場合
$ sudo apt-get install git
##macの場合
$ brew install git
初期設定
GUIから2段階認証してても良いと思う。
2段階した後はtokenをパスワードの場所に入れるようになる。
https://help.github.com/en/github/authenticating-to-github/creating-a-personal-access-token-for-the-command-line
git clone https://github.com/username/hoge.git
Username: user name
Password: token
秘密鍵生成(mac)
#-fで名前指定しないと.sshに元から他のキーがあるかもしれない
#ssh-keygen -t rsa -b 4096 -C "hoge@mail.com" -f ~/.ssh/git
# -t 暗号化方式を指定
# -b 暗号化強度を指定
# -C コメントを設定
ssh-keygen -t rsa -b 4096 -C "hoge@mail.com" -f ~/.ssh/git
ssh-keygen -t rsa -C "hoge@mail.com" -f ~/.ssh/git
バックグラウンドでssh-agentを開始
これも必要
eval "$(ssh-agent -s)"
Macの場合に追加
emacs ~/.ssh/config
Host *
AddKeysToAgent yes
UseKeychain yes
IdentityFile ~/.ssh/git
SSH 秘密鍵を ssh-agent に追加
~/.ssh/git.pubを確認してgitに登録
cat ~/.ssh/git.pub
# gitの名前の部分は上で指定したファイル名。
#mac
ssh-add -K ~/.ssh/git
#linux
ssh-add -k ~/.ssh/git
自分のプロフィールの設定。(リポジトリではない)
public keyの保存
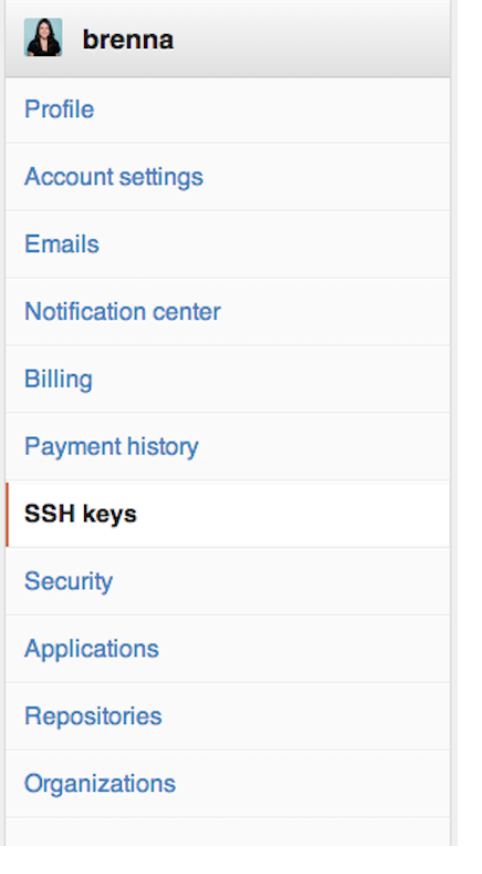
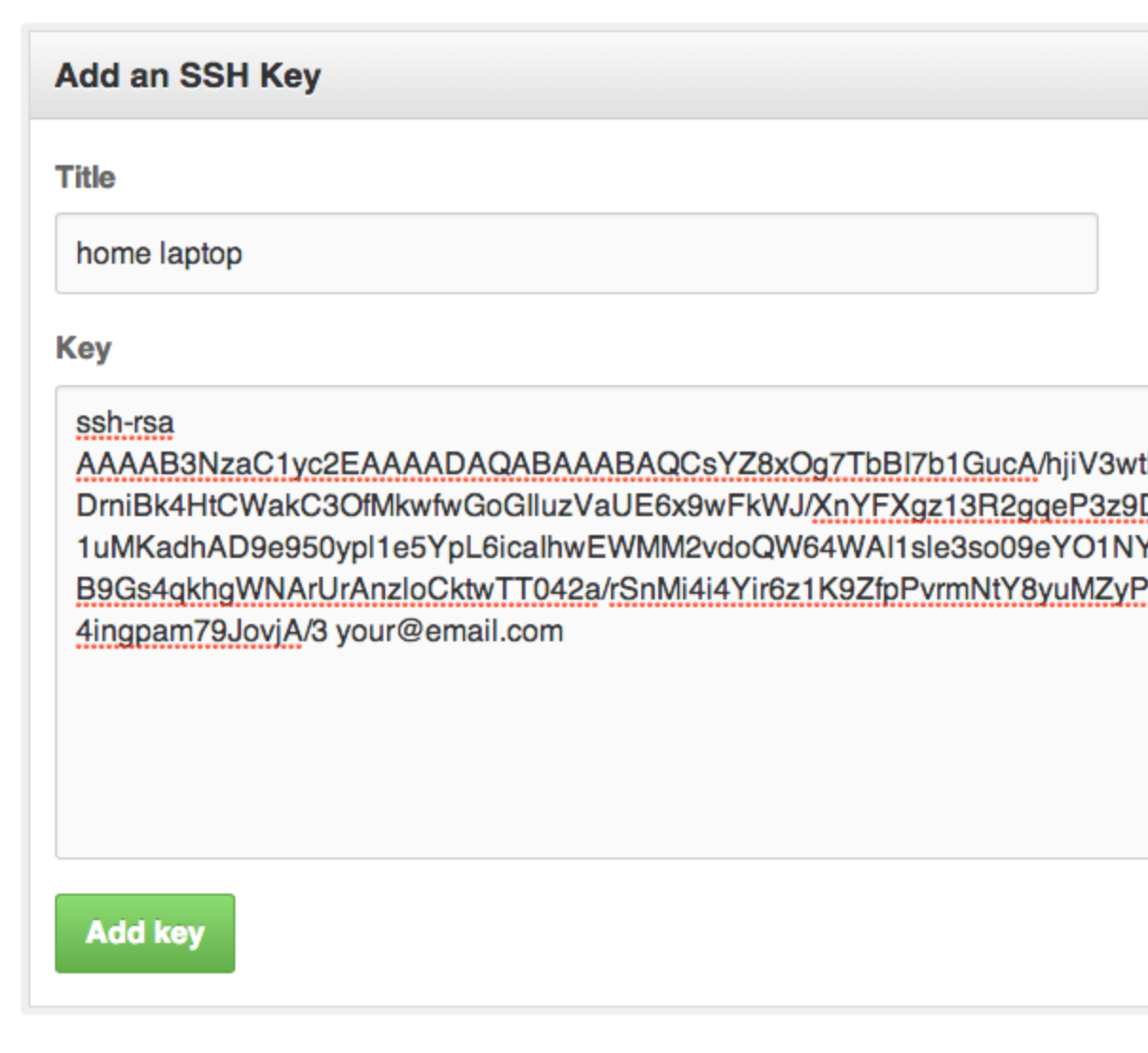
.git/configの
https://hoge.gitの部分を
git://hogehogeに変える。
もしname emailも設定されてなければ設定する。
git config user.name "FIRST_NAME LAST_NAME"
git config user.email "MY_NAME@example.com"
#一人で開発するときの基本的な流れ
リポジトリからソースを取ってくる
$ git clone hogehoge.git
"ソースコードを修正"
ローカルリポジトリに追加
$ git add hogehoge
変更したファイルを確認
$ git status
コミットする
$ git commit -m "コメント"
リモートリポジトリにプッシュ
$ git push origin master
※addを忘れるとコミットされないよ。
#間違ってaddした時
addしたものを全部gitから消す
$ git reset
#addを戻す
↓↓↓↓↓↓↓ あなたの記事の内容
コミットのみ取り消し
git reset --soft HEAD^
コミット取り消し、ファイルごと消す ※注意
git reset --hard HEAD
───────
```shell
$ git reset --hard HEAD
↑↑↑↑↑↑↑ 編集リクエストの内容
#masterをチェックアウト(masterに移動)
$ git checkout master
$ git pull
強制的にリモートからローカルに反映する時
git pullしてもローカル変わらないで、強制的に適応したい場合に使う。
git fetch origin
git reset --hard origin/master
#ブランチ名の付け方
- feature
新規機能の開発用。
- release
次回リリースの準備用。
- hotfix
現在の製品バージョンのバグフィックス用。
#100MB超のファイルをコミットしてしまい githubに pushを拒否された時
めんどくさいので、あまりでかいファイルはアップしない方がいいと思った。
http://www.walbrix.com/jp/blog/2013-10-github-large-files.html
git filter-branch --force --index-filter \
'git rm --cached --ignore-unmatch でかいファイル.tar.gz' \
--prune-empty --tag-name-filter cat -- --all
git commit --amend -CHEAD
git push
#.gitignore例
↓↓↓↓↓↓↓ あなたの記事の内容
macではgiboを使ってる。
brew install gibo
gibo dump Macos >> .gitignore
gibo dump Emacs >> .gitignore
#laravelの時
gibo dump Laravel >> .gitignore
───────
```:.gitignore
↑↑↑↑↑↑↑ 編集リクエストの内容
!.gitkeep
*.DS_Store
*~
*.egg/
*.pyc
*.pyo
*.cpp
*.so
\#*\#
.\#*
.coverage
.eggs/
.idea/
!.gitkeep
*.DS_Store
*.caffemodel
*.pkl
*.bin
*.vec
*.txt
*.db
*.h5
*.jpg
*.jpeg
*.png
*.npz
openaigym.*
*.gz
*.dot
#forkとは
fork するという行為はオリジナルへの貢献を前提とする。もしあなたがオリジナルに貢献する意図がないならば、オリジナル・リポジトリを fork する必要はない。貢献の意図がない場合は読み込み専用の URI を用いて git clone するだけでよい。
>
非常にわかりやすい説明。forkとcloneはほぼ同じ。
git branch
#ブランチチェック
git branch
#全部チェック
git branch --all
リモートブランチをローカルにチェックアウト
git checkout -b ブランチ名 origin/ブランチ名
#git pull
サーバーブランチから最新を取得。
git branch --set-upstream-to=origin/master masterのように指定してから実行。
http://qiita.com/kjirou/items/e0469aac0e128be380d6
#新しいブランチをローカルにcheckout
http://blog.inouetakuya.info/entry/20120826/1345979787
$ git checkout -b new-branch origin/new-branch
#push
$ git push origin BRANCH_NAME
#pull
$ git pull origin BRANCH_NAME
全部pull
git pull --all
#ブランチ間比較
リモートmasterとローカルdevelopを比較する例。
git diff origin/master develop
#ブランチ間比較:ファイル名のみ
リモートmasterとローカルdevelopを比較する例。
git diff origin/master develop --name-only
#ブランチ間比較:1ファイルのみ
リモートmasterとローカルdevelop間でsample/hoge.pyファイルを比較する。
git diff origin/master develop sample/hoge.py
#今のブランチにmasterをマージする
git merge --no-commit -Xtheirs origin/master
特定のcommitまで戻る
git reset コミットid
#【注意!!!】【注意!!!】【注意!!!】【注意!!!】
:ブランチ名でpushすると元のリモートブランチが消える。
#【注意!!!】【注意!!!】【注意!!!】【注意!!!】
git push origin :ブランチ名
git push origin ブランチ名
#過去のコミットを修正
viエディタのコマンド覚えてれば間違えたコメントなど編集し直せる。
git commit --amend
#readmeを書く
#ルートディレクトリー名を変える
guiから設定>名称変更ぽいやつで変更
git clone済みの場合は.git以下のconfigを編集
url=を変更後の名称に修正。でOK
エラー集
There is no tracking information for the current branch.
Please specify which branch you want to merge with.
See git-pull(1) for details.
解決策
git pull origin master
or
git pull origin hogehoge
or
git branch --set-upstream-to=origin/master master
git pull
The following untracked working tree files would be overwritten by checkout:
#全部消す
$ git checkout .
$ git clean -f
git@github.com: Permission denied (publickey).
fatal: Could not read from remote repository.
Please make sure you have the correct access rights
and the repository exists.
もう一回ssh-addやる必要ある。
eval "$(ssh-agent -s)"
ssh-add -k ~/.ssh/hoge
ssh -T git@github.com
毎回編集するのが手間であれば、設定ファイルに反映しても良い。
if ! ssh-add -l &>/dev/null; then
echo Adding keys...
ssh-add -k ~/.ssh/hoge
fi
source ~/.zshrc
特定ユーザーのログを確認する
$ git log --committer=[ユーザ名]
or
$ git log --author=[ユーザ名]
pushしても反映されない。
このコマンドでソースツリーのような感じで見れる。
origin/ブランチ名 (正確には、 リモート名/ブランチ名、以降「リモート追跡ブランチ」) みたいなやつがいて、それが変更されていなければ push できていません。
とありますが、変わってても反映されなかったので、ローカルのgitを再度cloneして手動でマージしてpushすると上がった。理由不明だった。
git log --graph --abbrev-commit --decorate --format=format:'%C(bold blue)%h%C(reset) - %C(bold green)(%ar)%C(reset) %C(white)%s%C(reset) %C(dim white)- %an%C(reset)%C(bold yellow)%d%C(reset)' --all
#未解決
##addをリセットする
git reset HEAD
$ git reset HEAD hogehoge/hogehoge.jpg
ワイルドカードも使える
git reset HEAD hogehoge/*.jpg
fatal: ambiguous argument 'HEAD': unknown revision or path not in the working tree.
Use '--' to separate paths from revisions, like this:
'git <command> [<revision>...] -- [<file>...]'
#参考
↓↓↓↓↓↓↓ あなたの記事の内容
https://git-scm.com/docs/git-reset
http://dackdive.hateblo.jp/entry/2014/05/27/112542
コマンド集。