Tweenアニメーションの作成
FPSAnimatorの基本的な使い方はこちらに記述しました。
今回の記事ではTweenAnimationに関して詳しく書きます。
Bitmapをアニメーションさせる
-
BitmapDrawerを作成
インスタンス作成時に、Bitmap をコンストラクタに設定。 -
DisplayObjectを生成し、FPSTextureView、FPSSurfaceView or ContainerにAddする。
withの後にTweenアニメーションをするならばtween()、放物線運動のアニメーションを行うならparabolic()を記述。
今回はTweenアニメーションなのでtween()。
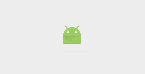
BitmapDrawer bitmapDrawer = new BitmapDrawer(bitmap)
.dpSize(this)
.scaleRegistration(bitmap.getWidth() / 2, bitmap.getHeight() / 2);
float x = 300;
float y = 400;
DisplayObject bitmapDisplay = new DisplayObject();
bitmapDisplay.with(bitmapDrawer)
.tween()
.tweenLoop(true)
.transform(x, y)
.to(500, x, y, 0, 6f, 6f, 0, Ease.SINE_IN_OUT)
.waitTime(300)
.transform(x, y, Util.convertAlphaFloatToInt(1f), 1f, 1f, 0)
.waitTime(300)
.end();
Textをアニメーションさせる
-
TextDrawerを作成
インスタンス作成時に、Stringの文字列と、textSizeやtextColorが設定されたPaintをコンストラクタに設定。
Paintにtypefaceを設定すれば、フォントを変えれますし、フォントアイコンを描画させることも可能です。 -
DisplayObjectを生成し、FPSTextureView、FPSSurfaceView or ContainerにAddする。
withの後にTweenアニメーションをするならばtween()、放物線運動のアニメーションを行うならparabolic()を記述。
今回はTweenアニメーションなのでtween()。
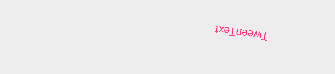
Paint paint = new Paint();
paint.setColor(ContextCompat.getColor(this, R.color.colorAccent));
paint.setTextSize(Util.convertDpToPixel(16, this));
String tweenTxt = "TweenText";
float textWidth = paint.measureText(tweenTxt);
TextDrawer textDrawer = new TextDrawer(tweenTxt, paint)
.rotateRegistration(textWidth / 2, textWidth / 2);
DisplayObject textDisplay = new DisplayObject();
textDisplay.with(textDrawer)
.tween()
.tweenLoop(true)
.transform(0, 800)
.waitTime(300)
.to(1000, windowWidth - textWidth, 800, 720f, Ease.SINE_OUT)
.waitTime(300)
.to(1000, 0, 800, 0f, Ease.SINE_IN)
.end();
Tweenのプロパティ
関数名 | 機能 |
---|---|
tweenLoop(boolean) | trueの場合、トゥイーンアニメーションが繰り返される。 |
transform(float x, float y) transform(float x, float y, int alpha, float scaleX, float scaleY, float rotation) |
アニメーションはせず、即座に引数の値に変化する。 |
toX(long animDuration, float x) toX(long animDuration, float x, Ease ease) |
xの値のアニメーションをさせる。値の単位はpixel |
toY(long animDuration, float y) toY(long animDuration, float y, Ease ease) |
yの値のアニメーションをさせる。値の単位はpixel |
alpha(long animDuration, float alpha) alpha(long animDuration, float alpha, Ease ease) |
透明度のアニメーションをさせる。ただし、Max値はint255である。1.0〜0の範囲内で指定したい場合、Util.convertAlphaIntToFloat(float)という関数が用意されています。 |
scale(long animDuration, float scaleX, float scaleY) scale(long animDuration, float scaleX, float scaleY, Ease ease) |
scaleのアニメーションをさせる。拡大の中心座標を操作したい場合、各DrawerにscaleRegistration関数が搭載されているので、そこで座標を設定する。 |
rotation(long animDuration, float rotation) rotation(long animDuration, float rotation, Ease ease) |
回転のアニメーションをさせる。回転の中心座標を操作したい場合、各DrawerにrotateRegistration関数が搭載されているので、そこで座標を設定する。 |
waitTime(long animDuration) | アニメーションせず、その時のプロパティで描画する。 |
call(AnimCallBack callBack) | トゥイーンの開始時や終了時など、特定のタイミングでコールバック関数を実行したい場合に使用 |
to(long animDuration, float x, float y) to(long animDuration, float x, float y, Ease ease) to(long animDuration, float x, float y, int alpha) to(long animDuration, float x, float y, int alpha, Ease ease) to(long animDuration, float x, float y, float scaleX, float scaleY) to(long animDuration, float x, float y, float scaleX, float scaleY, Ease ease) to(long animDuration, float x, float y, float rotation) to(long animDuration, float x, float y, float rotation, Ease ease) to(long animDuration, float x, float y, int alpha, float scaleX, float scaleY, float rotation, Ease ease) |
現在の状態から引数のターゲットの状態まで、animDurationの時間でトゥイーンアニメーションする。 |
記述例
DisplayObject bitmapDisplay = new DisplayObject();
bitmapDisplay
.with(new BitmapDrawer(bitmap))
.tween()
.tweenLoop(true)
.transform(bitmapX, bitmapY)
.toX(1600, UIUtil.getWindowWidth(this) - bitmap.getWidth(), Ease.BACK_IN_OUT)
.waitTime(1000)
.alpha(1000, 0f)
.alpha(1000, 1f)
.scale(500, -1f, 1f, Ease.CUBIC_IN)
.scale(500, 1f, 1f, Ease.CUBIC_OUT)
.call(new AnimCallBack() {
@Override
public void call() {
Snackbar.make(mFPSTextureView, "Animation finished!", Snackbar.LENGTH_LONG)
.setAction("Action", null).show();
}
})
.end();
Easing
ぜひ、libraryにgithubのStarをお願いします。
FPSAnimator