前回の続き
Menus and toolbars
こちらのサイトを日本語でざっくりとまとめていきます。
【ステータスバー】
Statusbar.py
# !/usr/bin/python3
# -*- coding: utf-8 -*-
import sys
from PyQt5.QtWidgets import QMainWindow, QApplication
class Example(QMainWindow):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
# ステータスバーのメッセージを設定
self.statusBar().showMessage('Ready')
self.setGeometry(300, 300, 250, 150)
self.setWindowTitle('Statusbar')
self.show()
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = Example()
sys.exit(app.exec_())
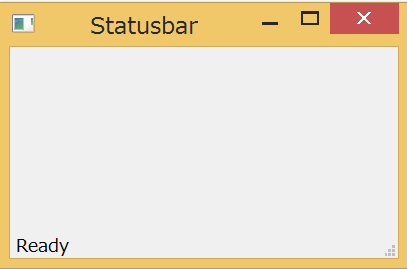
【メニューバー】
Menubar.py
# !/usr/bin/python3
# -*- coding: utf-8 -*-
import sys
from PyQt5.QtWidgets import QMainWindow, QAction, qApp, QApplication
from PyQt5.QtGui import QIcon
class Example(QMainWindow):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
# アイコン付加、ラベルはExitのアクションオブジェクト作成
exitAction = QAction(QIcon('imoyokan.jpg'), '&Exit', self)
# ショートカットできるようにする
exitAction.setShortcut('Ctrl+Q')
# カーソルを乗せるとステータスバーへ表示
exitAction.setStatusTip('Exit application')
# Exitボタンを押すと表示終了
exitAction.triggered.connect(qApp.quit)
self.statusBar()
# メニューバー作成
menubar = self.menuBar()
fileMenu = menubar.addMenu('&File')
# exitActionを紐づける
fileMenu.addAction(exitAction)
self.setGeometry(300, 300, 300, 200)
self.setWindowTitle('Menubar')
self.show()
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = Example()
sys.exit(app.exec_())
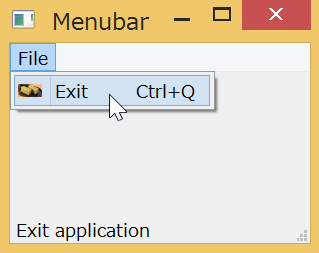
【ツールバー】
Toolbar.py
# !/usr/bin/python3
# -*- coding: utf-8 -*-
import sys
from PyQt5.QtWidgets import QMainWindow, QAction, qApp, QApplication
from PyQt5.QtGui import QIcon
class Example(QMainWindow):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
# 画像付き 吹き出しをExitにするアクションオブジェクト作成
exitAction = QAction(QIcon('imoyokan.jpg'), 'Exit', self)
exitAction.setShortcut('Ctrl+Q')
exitAction.triggered.connect(qApp.quit)
# ツールバー作成
self.toolbar = self.addToolBar('Exit')
self.toolbar.addAction(exitAction)
self.setGeometry(300, 300, 300, 200)
self.setWindowTitle('Toolbar')
self.show()
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = Example()
sys.exit(app.exec_())

【一緒にまとめて、テキスト入力追加】
Putting_it_together.py
# !/usr/bin/python3
# -*- coding: utf-8 -*-
import sys
from PyQt5.QtWidgets import QMainWindow, QTextEdit, QAction, QApplication
from PyQt5.QtGui import QIcon
class Example(QMainWindow):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
# テキスト入力欄の作成
textEdit = QTextEdit()
self.setCentralWidget(textEdit)
exitAction = QAction(QIcon('imoyokan.jpg'), 'Exit', self)
exitAction.setShortcut('Ctrl+Q')
exitAction.setStatusTip('Exit application')
exitAction.triggered.connect(self.close)
self.statusBar()
menubar = self.menuBar()
fileMenu = menubar.addMenu('&File')
fileMenu.addAction(exitAction)
toolbar = self.addToolBar('Exit')
toolbar.addAction(exitAction)
self.setGeometry(300, 300, 350, 250)
self.setWindowTitle('Main window')
self.show()
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = Example()
sys.exit(app.exec_())

次回はLayout managementをざっくり試していきます。