環境
- Mac OSX(El Capitan)
- Python 2.7
準備
Google Drive APIの発行
プロジェクトの作成
- プロジェクトが無い場合は右上のExampleの箇所から新規プロジェクトの作成を行います

- プロジェクト名とIDを決める事が出来ます
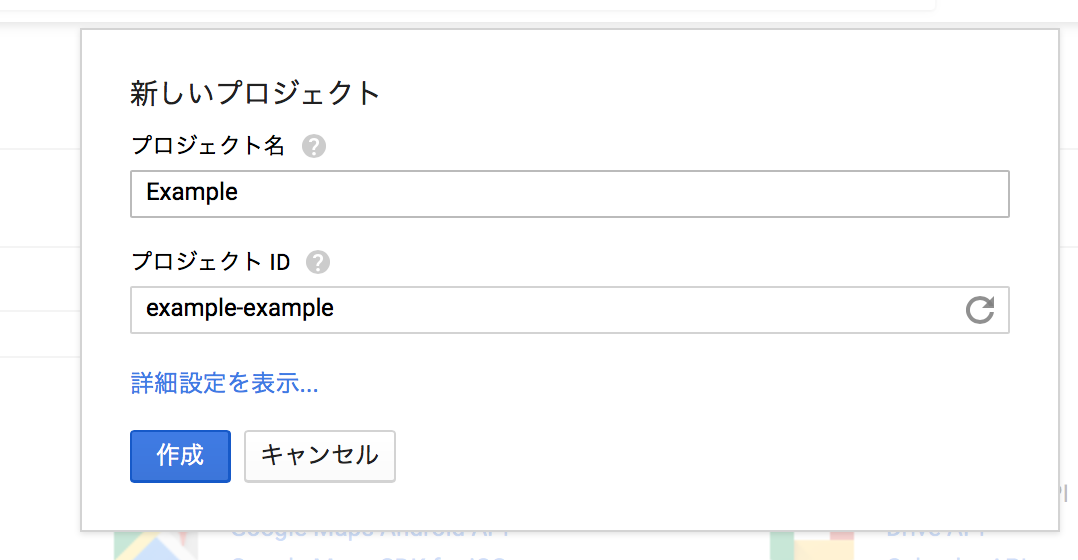
API
- Google Developers ConsoleからGoogle Drive APIを有効にします

- APIを有効にしたら左の認証情報を選択し、認証情報を作成 -> サービスアカウントキーを選択します (サービスアカウントが無い場合は作成して下さい)
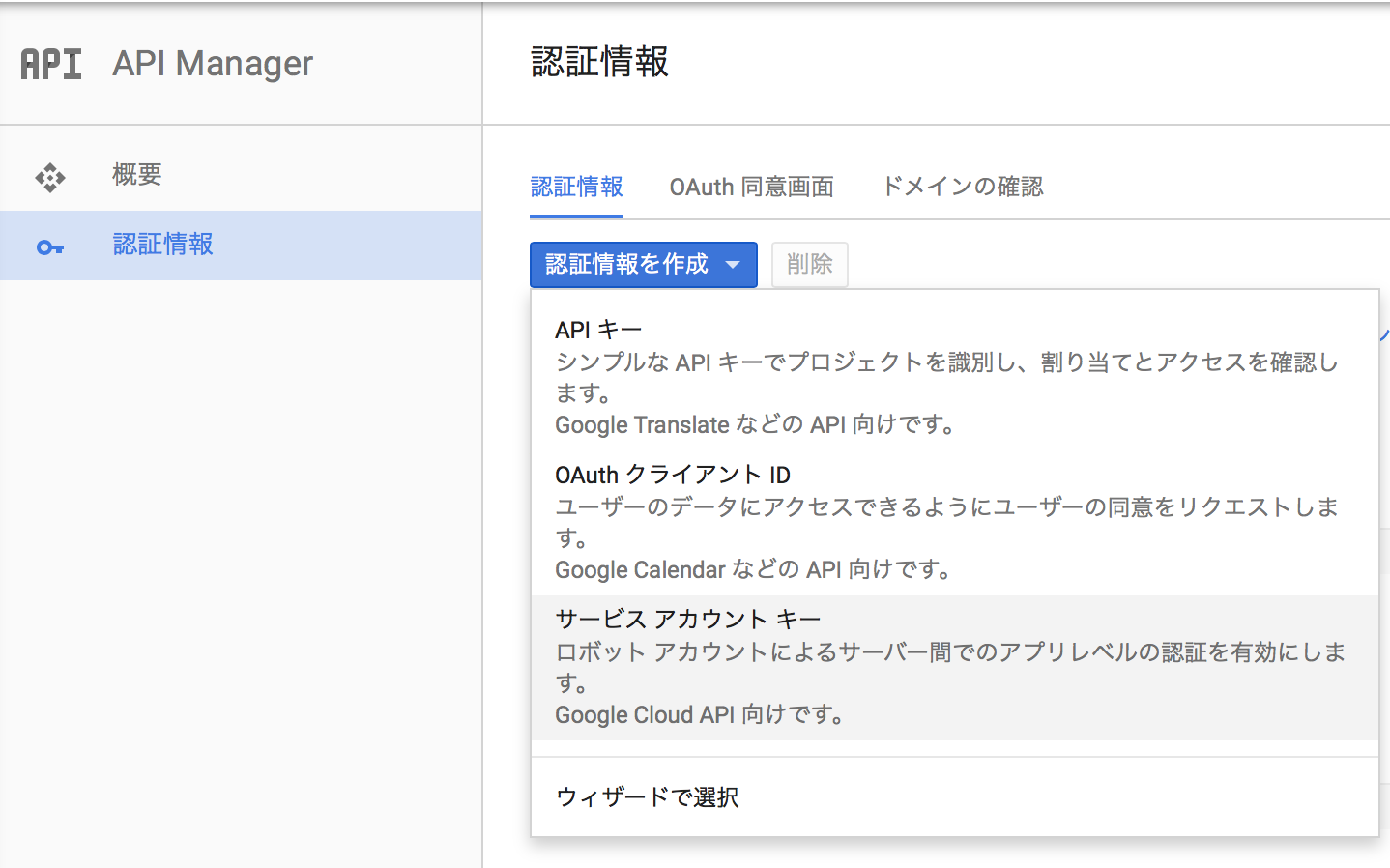
- JSONとP12形式が選択可能ですが、ここではJSONを利用します
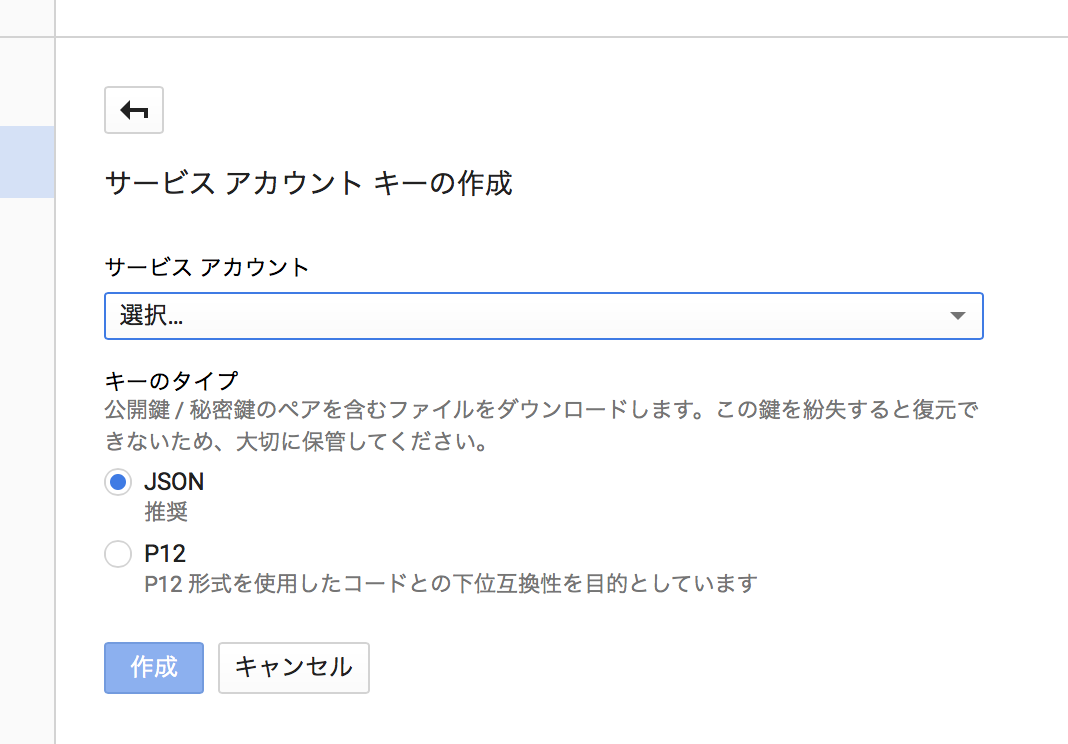
- 完了するとJSONファイルが自動的にダウンロードされます
スプレッドシート側の共有設定
- 使用するスプレッドシートの右上にある共有ボタンを押し、ダウンロードしたJSONファイルに記載されている
client_email
の欄のアドレスを入力します
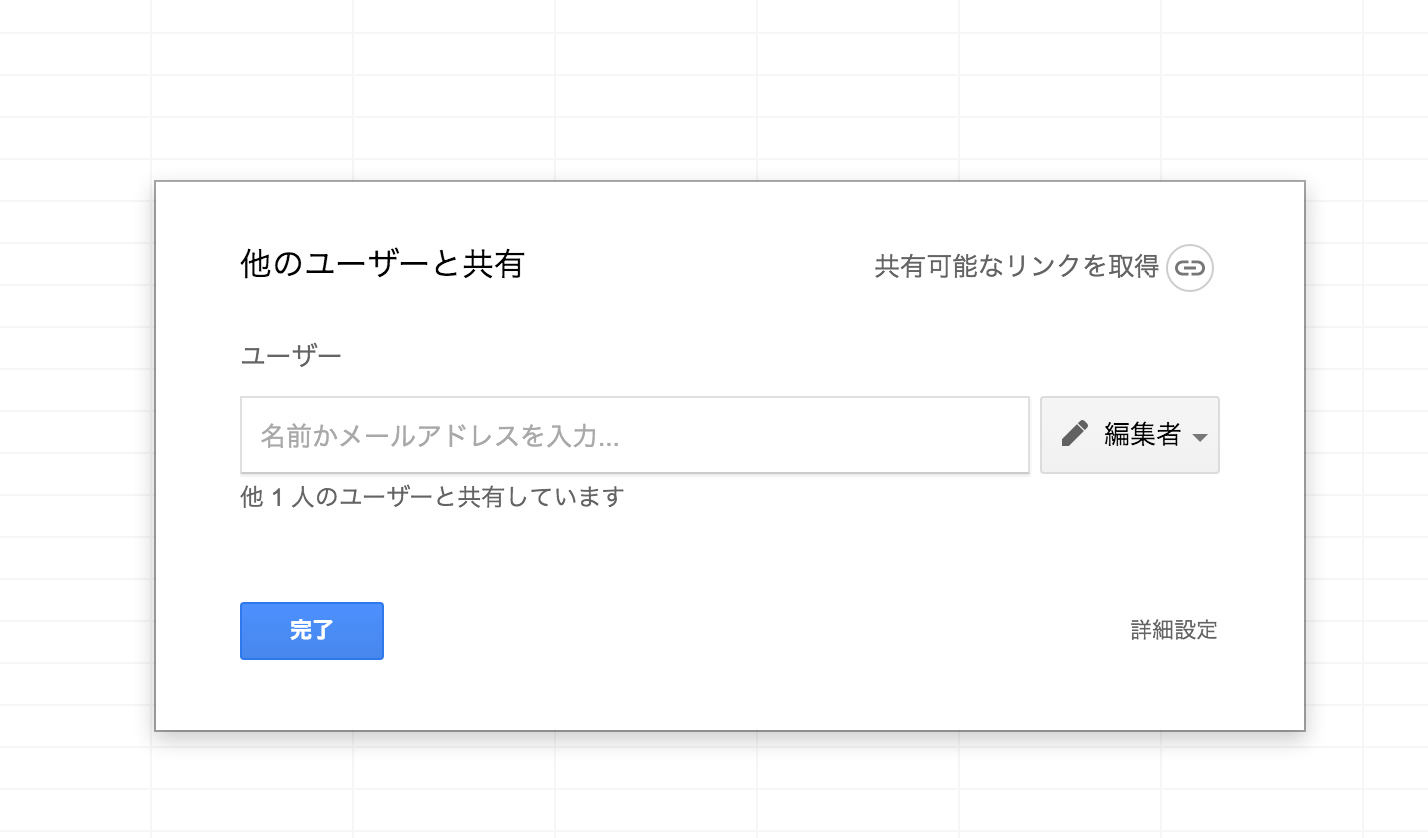
- id
urlのhttps://docs.google.com/spreadsheets/d/***********/edit
アスタリスク部分がidになります
Python
ライブラリのインストール
- ライブラリ
-
oauth2client
sudo pip install --upgrade oauth2client --ignore-installed six
-
gdata (google正規: 今回は使わない)
sudo pip install gdata --ignore-installed six
-
gspread (こちらの方が便利そうなので今回はこっちを使用します)
sudo pip install gspread --ignore-installed six
※ El Capitanでは System Integrity Protection (SIP)のせいでpipのinstallが失敗するので、--ignore-installed six
を最後に付けてます
Code
- 以前は
SignedJwtAssertionCredentials
クラスが使用されていたのですが、2016/02にライブラリのアップデートがありServiceAccountCredentials
を使うようになりました
import os
import gspread
from oauth2client.service_account import ServiceAccountCredentials
def main():
scope = ['https://spreadsheets.google.com/feeds']
doc_id = 'Your doc id'
path = os.path.expanduser("Path of json file")
credentials = ServiceAccountCredentials.from_json_keyfile_name(path, scope)
client = gspread.authorize(credentials)
gfile = client.open_by_key(doc_id)
worksheet = gfile.sheet1
records = worksheet.get_all_values()
for record in records:
print(record)
if __name__ == '__main__':
main()